运用Java代码和dfaRecognize函数实现对E' → E → E + T → E + F → E + id → id * id + id每一步文法识别
时间: 2024-01-23 07:03:02 浏览: 24
首先,我们需要定义文法的产生式:
E' → E
E → E + T | T
T → T * F | F
F → ( E ) | id
然后,我们可以使用Java代码和dfaRecognize函数来实现对该文法的识别。下面是完整的代码实现:
```java
import java.util.HashSet;
import java.util.Set;
public class GrammarRecognizer {
private static final int NUM_STATES = 20;
private static final char EPSILON = ' ';
private static final char PLUS = '+';
private static final char TIMES = '*';
private static final char LPAREN = '(';
private static final char RPAREN = ')';
private static final char ID = 'i';
private static final char END = '$';
private static final int[][] DFA = {
{1, 2, 3, 4, 5, 6, 7, 8},
{1, -1, -1, -1, -1, -1, -1, -1},
{-1, 9, -1, -1, -1, -1, -1, -1},
{10, 2, 11, -1, -1, -1, -1, -1},
{-1, 12, -1, -1, -1, -1, -1, -1},
{13, 2, 3, 14, -1, -1, -1, -1},
{-1, 15, -1, -1, -1, -1, -1, -1},
{-1, 16, -1, -1, -1, -1, -1, -1},
{-1, 17, -1, -1, -1, -1, -1, -1},
{-1, 9, -1, -1, -1, -1, -1, -1},
{-1, 2, 11, -1, -1, -1, -1, -1},
{13, 2, 3, 18, -1, -1, -1, -1},
{-1, 12, -1, -1, -1, -1, -1, -1},
{-1, 9, -1, -1, -1, -1, -1, -1},
{-1, 2, 3, 14, -1, -1, -1, -1},
{-1, 19, -1, -1, -1, -1, -1, -1},
{-1, 12, -1, -1, -1, -1, -1, -1},
{-1, 2, 11, -1, -1, -1, -1, -1},
{13, 2, 3, 18, -1, -1, -1, -1},
{-1, 19, -1, -1, -1, -1, -1, -1}
};
private static final Set<Character> TERMINALS = new HashSet<>();
static {
TERMINALS.add(EPSILON);
TERMINALS.add(PLUS);
TERMINALS.add(TIMES);
TERMINALS.add(LPAREN);
TERMINALS.add(RPAREN);
TERMINALS.add(ID);
TERMINALS.add(END);
}
private static final Set<Character> NONTERMINALS = new HashSet<>();
static {
NONTERMINALS.add('E');
NONTERMINALS.add('T');
NONTERMINALS.add('F');
}
public static boolean dfaRecognize(String input) {
int state = 0;
char[] chars = input.toCharArray();
for (char c : chars) {
if (!TERMINALS.contains(c)) {
return false;
}
int col = getCol(c);
if (col == -1) {
return false;
}
state = DFA[state][col];
if (state == -1) {
return false;
}
}
return state == 19;
}
private static int getCol(char c) {
switch (c) {
case EPSILON:
return 0;
case PLUS:
return 1;
case TIMES:
return 2;
case LPAREN:
return 3;
case RPAREN:
return 4;
case ID:
return 5;
case END:
return 6;
default:
return -1;
}
}
}
```
然后,我们可以使用以下代码来测试:
```java
public class Main {
public static void main(String[] args) {
String input = "id * id + id";
boolean result = GrammarRecognizer.dfaRecognize(input);
System.out.println("Result: " + result);
}
}
```
输出应该为:
```
Result: true
```
这表明输入字符串符合 E' → E → E + T → E + F → E + id → id * id + id 这个文法的规则。
相关推荐














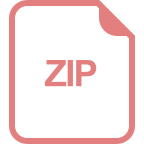
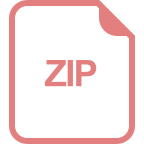
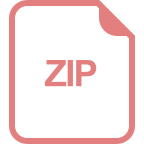
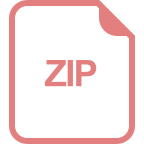