arduino中startsWith是什么函数
时间: 2023-06-17 11:07:21 浏览: 119
startsWith是Arduino中的一个字符串函数,用于检查一个字符串是否以另一个字符串开头。该函数的语法如下:
```
startsWith(str, prefix)
```
其中,str为要检查的字符串,prefix为要匹配的前缀字符串。如果str以prefix开头,则该函数返回true,否则返回false。
以下是一个示例:
```
String str = "Hello, world!";
if (str.startsWith("Hello")) {
Serial.println("The string starts with 'Hello'");
} else {
Serial.println("The string does not start with 'Hello'");
}
```
在上面的示例中,如果str以"Hello"开头,则会输出"The string starts with 'Hello'",否则会输出"The string does not start with 'Hello'"。
相关问题
arduino字符串函数
Arduino字符串函数是用于处理字符串的函数,可以在Arduino编程中使用。其中一些常用的函数包括:
1. `length()`:返回字符串的长度。
2. `charAt(index)`:返回字符串中指定索引位置的字符。
3. `substring(start, end)`:返回从指定起始索引到结束索引之间的子字符串。
4. `startsWith(prefix)`:检查字符串是否以指定的前缀开头。
5. `endsWith(suffix)`:检查字符串是否以指定的后缀结尾。
6. `indexOf(substring)`:返回指定子字符串第一次出现的索引。
7. `replace(oldValue, newValue)`:将字符串中的旧值替换为新值。
8. `toInt()`:将字符串转换为整数。
9. `toFloat()`:将字符串转换为浮点数。
10. `concat(string)`:将指定的字符串连接到当前字符串的末尾。
arduino如何读取gprmc
要在Arduino中读取GPRMC数据,您需要使用GPS模块并连接它到Arduino板。然后,您可以使用软件串口库将GPS模块的输出连接到Arduino板上的串口。接下来,您可以使用串口数据读取功能,从GPS模块读取NMEA格式的数据,包括GPRMC数据。
在Arduino中读取GPRMC数据的步骤如下:
1. 将GPS模块连接到Arduino板上的串口。确保正确连接RX和TX引脚。
2. 在Arduino IDE中导入软件串口库(SoftwareSerial.h)。
3. 创建一个SoftwareSerial对象,将其连接到GPS模块的RX和TX引脚。
4. 在Arduino的setup()函数中打开串口通讯。
5. 在Arduino的loop()函数中使用串口数据读取功能,从GPS模块读取NMEA格式的数据。
6. 使用字符串处理函数,将GPRMC数据从NMEA格式的数据中提取出来。
7. 解析GPRMC数据,获取位置信息等有用的数据。
下面是一个简单的示例代码,可以帮助您开始读取GPRMC数据:
```
#include <SoftwareSerial.h>
SoftwareSerial gpsSerial(10, 11); // RX, TX
void setup() {
Serial.begin(9600);
gpsSerial.begin(9600);
}
void loop() {
if (gpsSerial.available()) {
String nmea = gpsSerial.readStringUntil('\n');
if (nmea.startsWith("$GPRMC")) {
// Parse GPRMC data
// Extract latitude, longitude, speed, etc.
// ...
Serial.println(nmea);
}
}
}
```
请注意,上述代码仅是一个示例,您需要根据您的GPS模块和应用程序的要求进行修改。
相关推荐
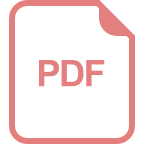
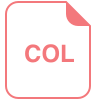
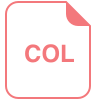










