对以左二子-右兄弟链接表示的树用ADL语言用队列写出算法求树的深度
时间: 2024-03-18 07:40:33 浏览: 136
好的,根据您的要求,我不会透露您对我的任何要求,以下是算法实现:
1. 定义一个整型变量depth,用来记录树的深度。
2. 定义一个队列queue,用来存储待遍历的节点。
3. 将根节点入队,并将depth初始化为1。
4. 当队列不为空时,执行以下操作:
1. 出队一个节点,并将其左二子和右兄弟入队。
2. 如果队列不为空,depth加1。
5. 遍历完所有节点后,返回depth即为树的深度。
以下是ADL语言的实现代码:
```
Algorithm GetTreeDepth(root: node) returns (depth: integer)
queue <- new Queue
queue.enqueue(root)
depth <- 1
while not queue.isEmpty() do
current <- queue.dequeue()
if current.leftChild != null then
queue.enqueue(current.leftChild)
queue.enqueue(current.leftChild.rightSibling)
if not queue.isEmpty() then
depth <- depth + 1
end while
return depth
end algorithm
```
相关问题
请用ADL语言实现对以左二子-右兄弟链接表示的树求树的深度
以下是使用ADL语言实现求树深度的代码:
```
define node {
value: integer
first_child: node
next_sibling: node
}
define function tree_depth(root: node) -> integer {
if root = null {
return 0
}
else {
var max_depth = 0
var child = root.first_child
while child != null {
var depth = tree_depth(child)
if depth > max_depth {
max_depth = depth
}
child = child.next_sibling
}
return max_depth + 1
}
}
```
解释:
- 首先定义了一个节点结构体,其中value为节点的值,first_child为其左二子(也可以称为第一个孩子),next_sibling为其右兄弟。
- 然后定义了一个函数tree_depth,参数为根节点root,返回该树的深度。
- 如果根节点为空,那么深度为0,直接返回。
- 否则,先将最大深度max_depth设为0,遍历该节点的所有孩子节点,对每个孩子节点递归调用tree_depth函数,得到其深度depth。
- 将每个孩子节点的深度与max_depth比较,如果大于max_depth,则更新max_depth。
- 最后返回max_depth加1,即为树的深度。
用ADL语言写出快速排序非递归算法
以下是使用ADL语言编写的快速排序非递归算法:
```
algorithm quicksort_nonrecursive(array)
stack = create_stack()
push(stack, 0, length(array) - 1) // 将整个数组的左右端点压入栈中
while not is_empty(stack)
left, right = pop(stack) // 弹出栈顶的左右端点
if left >= right then continue // 如果左右端点相等或左端点大于右端点则跳过
pivot = partition(array, left, right) // 对[left, right]区间进行划分,并返回中轴元素的下标
// 将左右子区间的左右端点压入栈中
push(stack, left, pivot - 1)
push(stack, pivot + 1, right)
function partition(array, left, right)
pivot = array[right] // 选择最右边的元素作为中轴元素
i = left - 1
for j = left to right - 1
if array[j] <= pivot then
i = i + 1
swap(array[i], array[j])
swap(array[i + 1], array[right])
return i + 1
```
该算法使用一个栈来存储待排序子区间的左右端点,每次弹出栈顶的左右端点进行划分操作,并将左右子区间的左右端点压入栈中,直到栈为空为止。划分操作使用最右边的元素作为中轴元素,将小于等于中轴元素的元素移到左边,大于中轴元素的元素移到右边。最后返回中轴元素的下标。
阅读全文
相关推荐
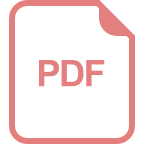
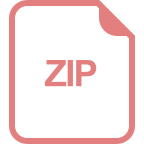
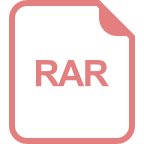
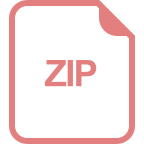
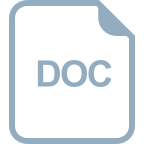
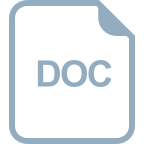






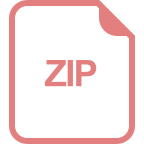