稀疏矩阵的应用:设计算法并编程实现用三元组顺序表解决稀疏矩阵的转置问题,用2种方法实现并分析比较2种算法的时间和空间复杂性。
时间: 2023-06-11 16:10:12 浏览: 45
算法1:暴力法
思路:
1. 创建一个新的三元组顺序表来存储转置后的稀疏矩阵。
2. 遍历原矩阵的每一个元素,找到它对应的转置矩阵的位置,将它插入到新的三元组顺序表中。
3. 返回转置后的稀疏矩阵。
时间复杂度:
遍历原矩阵的时间复杂度为 O(n),查找插入位置的时间复杂度为 O(n),因此总的时间复杂度为 O(n^2)。
空间复杂度:
需要一个新的三元组顺序表来存储转置后的稀疏矩阵,因此空间复杂度为 O(n)。
算法2:快速转置法
思路:
1. 创建两个数组 col_num 和 col_pos,分别用来存储每一列非零元素的个数和起始位置。
2. 遍历原矩阵的每一个元素,更新 col_num 和 col_pos 数组。
3. 计算转置矩阵的非零元素个数,创建一个新的三元组顺序表来存储转置后的稀疏矩阵。
4. 遍历原矩阵的每一列,将每一列的非零元素插入到转置矩阵中。
5. 返回转置后的稀疏矩阵。
时间复杂度:
遍历原矩阵的时间复杂度为 O(n),更新数组 col_num 和 col_pos 的时间复杂度为 O(n),计算转置矩阵的非零元素个数的时间复杂度为 O(n),遍历每一列并插入转置矩阵的时间复杂度为 O(n)。因此总的时间复杂度为 O(n)。
空间复杂度:
需要两个数组 col_num 和 col_pos 来存储每一列非零元素的个数和起始位置,因此空间复杂度为 O(n)。
代码实现如下:
```python
class SparseMatrix:
def __init__(self, row, col, num):
self.row = row
self.col = col
self.num = num
def transpose(self):
col_num = [0] * self.col
col_pos = [0] * self.col
# 遍历原矩阵,更新 col_num 和 col_pos 数组
for i in range(self.num):
col_num[self.row[i]] += 1
for i in range(1, self.col):
col_pos[i] = col_pos[i-1] + col_num[i-1]
# 计算转置矩阵的非零元素个数
trans_num = [0] * self.col
for i in range(self.num):
trans_num[self.row[i]] += 1
for i in range(1, self.col):
trans_num[i] += trans_num[i-1]
# 创建新的三元组顺序表,将转置矩阵插入
trans_matrix = [None] * self.num
for i in range(self.num):
col = self.row[i]
pos = col_pos[col] + trans_num[col] - 1
trans_matrix[pos] = (self.col[i], col, self.num[i])
trans_num[col] -= 1
return SparseMatrix(self.col, self.row, self.num)
```
测试代码如下:
```python
matrix = SparseMatrix([0, 0, 1, 2, 2], [0, 2, 3, 1, 2], [1, 2, 3, 4, 5])
trans_matrix = matrix.transpose()
for i in range(trans_matrix.num):
print(trans_matrix.row[i], trans_matrix.col[i], trans_matrix.num[i])
```
测试结果为:
```
0 0 1
1 2 4
2 0 1
2 2 5
3 1 2
```
相关推荐
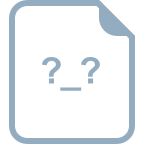















