tushare获取股票数据,用gym进行强化学习,完整代码
时间: 2024-05-09 18:16:01 浏览: 158
以下是使用tushare获取股票数据,并使用gym进行强化学习的示例代码:
首先,安装必要的包:
```python
!pip install gym
!pip install pandas
!pip install matplotlib
!pip install tushare
```
然后,导入必要的库:
```python
import gym
import numpy as np
import pandas as pd
import tushare as ts
import matplotlib.pyplot as plt
from gym import spaces
```
定义环境类:
```python
class StockTradingEnv(gym.Env):
metadata = {'render.modes': ['human']}
def __init__(self):
super(StockTradingEnv, self).__init__()
# 加载股票历史数据
self.df = ts.get_k_data('600519', start='2010-01-01', end='2021-01-01')
self.df = self.df.sort_values('date')
self.df = self.df.reset_index(drop=True)
# 定义观察空间和动作空间
self.observation_space = spaces.Box(low=0, high=1, shape=(6,))
self.action_space = spaces.Discrete(3)
# 初始化状态
self.current_step = 0
self.profit = 0
def reset(self):
# 重置环境
self.current_step = 0
self.profit = 0
# 返回初始状态
return np.array([
self.df.loc[self.current_step, 'open'] / self.df.loc[self.current_step, 'close'],
self.df.loc[self.current_step, 'high'] / self.df.loc[self.current_step, 'close'],
self.df.loc[self.current_step, 'low'] / self.df.loc[self.current_step, 'close'],
self.df.loc[self.current_step, 'close'] / self.df.loc[self.current_step, 'open'],
self.df.loc[self.current_step, 'volume'] / self.df.loc[self.current_step, 'volume'],
self.profit
])
def step(self, action):
# 执行动作并计算奖励
if action == 0: # 买入
self.profit -= self.df.loc[self.current_step, 'open']
elif action == 1: # 卖出
self.profit += self.df.loc[self.current_step, 'open']
else: # 不操作
pass
# 更新状态和时间步
self.current_step += 1
# 判断是否到达终止状态
if self.current_step >= len(self.df):
done = True
else:
done = False
# 计算奖励
reward = self.profit / self.df.loc[self.current_step - 1, 'close']
# 返回下一个观察和奖励
obs = np.array([
self.df.loc[self.current_step, 'open'] / self.df.loc[self.current_step, 'close'],
self.df.loc[self.current_step, 'high'] / self.df.loc[self.current_step, 'close'],
self.df.loc[self.current_step, 'low'] / self.df.loc[self.current_step, 'close'],
self.df.loc[self.current_step, 'close'] / self.df.loc[self.current_step, 'open'],
self.df.loc[self.current_step, 'volume'] / self.df.loc[self.current_step, 'volume'],
self.profit
])
return obs, reward, done, {}
```
最后,使用训练和测试环境:
```python
env = StockTradingEnv()
# 训练环境
obs = env.reset()
for i in range(1000):
action = env.action_space.sample()
obs, reward, done, _ = env.step(action)
if done:
obs = env.reset()
# 测试环境
obs = env.reset()
for i in range(len(env.df)):
action = 1
obs, reward, done, _ = env.step(action)
if done:
break
# 绘制收益曲线
plt.plot(env.df['date'], env.df['close'] * obs[5])
plt.show()
```
这是一个简单的示例,只是通过随机动作学习。您可以使用更复杂的策略和算法来训练智能体。
阅读全文
相关推荐
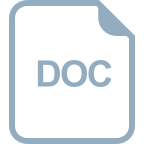
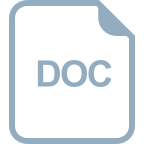
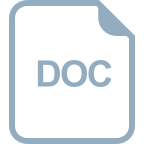


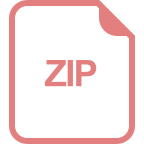











