使用rosserial_msgs/TopicInfo来和微控制器通讯控制两个电机,实现云台在X轴和Y轴的旋转,使用ROS中cpp写出来
时间: 2024-05-12 15:19:21 浏览: 17
以下是使用rosserial_msgs/TopicInfo来和微控制器通讯,控制两个电机实现云台在X轴和Y轴的旋转的ROS CPP代码。
```cpp
#include <ros.h>
#include <std_msgs/Int32.h>
#include <rosserial_msgs/TopicInfo.h>
ros::NodeHandle nh;
// Motor control pins
const int X_STEP_PIN = 2;
const int X_DIR_PIN = 3;
const int Y_STEP_PIN = 4;
const int Y_DIR_PIN = 5;
// Motor speed control variables
int x_speed = 0;
int y_speed = 0;
// ROS topic names
const char* x_topic_name = "x_axis";
const char* y_topic_name = "y_axis";
// ROS topics
ros::Publisher x_pub(x_topic_name, &x_speed);
ros::Publisher y_pub(y_topic_name, &y_speed);
ros::Subscriber<rosserial_msgs::TopicInfo> sub_topic_info("rosserial_topics", &topic_info_callback);
void topic_info_callback(const rosserial_msgs::TopicInfo& msg) {
// Check if the topic is for controlling the X-axis motor
if (strcmp(msg.topic_name, x_topic_name) == 0) {
// Set the speed of the X-axis motor
x_speed = msg.message_id;
digitalWrite(X_DIR_PIN, msg.topic_type == "std_msgs/Int32" ? x_speed > 0 : x_speed < 0);
digitalWrite(X_STEP_PIN, HIGH);
delayMicroseconds(abs(x_speed));
digitalWrite(X_STEP_PIN, LOW);
delayMicroseconds(abs(x_speed));
}
// Check if the topic is for controlling the Y-axis motor
else if (strcmp(msg.topic_name, y_topic_name) == 0) {
// Set the speed of the Y-axis motor
y_speed = msg.message_id;
digitalWrite(Y_DIR_PIN, msg.topic_type == "std_msgs/Int32" ? y_speed > 0 : y_speed < 0);
digitalWrite(Y_STEP_PIN, HIGH);
delayMicroseconds(abs(y_speed));
digitalWrite(Y_STEP_PIN, LOW);
delayMicroseconds(abs(y_speed));
}
}
void setup() {
// Setup motor control pins as outputs
pinMode(X_STEP_PIN, OUTPUT);
pinMode(X_DIR_PIN, OUTPUT);
pinMode(Y_STEP_PIN, OUTPUT);
pinMode(Y_DIR_PIN, OUTPUT);
// Setup ROS communication
nh.initNode();
nh.advertise(x_pub);
nh.advertise(y_pub);
nh.subscribe(sub_topic_info);
// Set default motor speeds to zero
x_speed = 0;
y_speed = 0;
}
void loop() {
// Publish motor speeds to ROS
x_pub.publish(&x_speed);
y_pub.publish(&y_speed);
// Handle ROS communication
nh.spinOnce();
}
```
解释:
- 首先,我们定义了ROS节点和电机控制的引脚。
- 接下来,我们定义了两个变量来存储X和Y轴电机的速度。
- 然后,我们定义了两个ROS主题,用来发布X和Y轴电机的速度。
- 我们还定义了一个ROS主题订阅器,用来接收来自微控制器的控制指令。
- 在setup()函数中,我们设置了电机控制引脚为输出,并初始化了ROS节点。
- 在loop()函数中,我们将电机速度发布到ROS主题,并处理来自微控制器的控制指令。
在topic_info_callback()函数中,我们检查收到的ROS主题信息是否是用于控制X轴电机或Y轴电机的信息。如果是,则将速度设置为传递的消息ID,并根据速度的正负值设置电机方向。然后,我们将X或Y轴电机旋转一步,根据速度的绝对值设置延迟时间,以控制电机速度。
注意,此代码仅用于演示目的,实际使用时需要根据实际情况进行修改和调整。
相关推荐
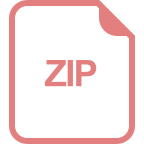
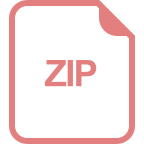














