在unity引擎中写一个图片从四周慢慢向中心虚化的shader,能够手动控制虚化的强度和范围
时间: 2023-11-27 07:53:54 浏览: 35
可以使用Unity的ShaderLab语言编写一个自定义的Shader来实现这个效果。下面是一个简单的实现示例:
首先,我们需要定义一些参数,包括虚化强度和范围:
```shaderlab
Shader "Custom/Blur" {
Properties {
_MainTex ("Texture", 2D) = "white" {}
_BlurStrength ("Blur Strength", Range(0, 10)) = 5
_BlurRange ("Blur Range", Range(0, 1)) = 0.5
}
SubShader {
Tags {"Queue"="Transparent" "RenderType"="Opaque"}
LOD 100
Pass {
CGPROGRAM
#pragma vertex vert
#pragma fragment frag
#include "UnityCG.cginc"
struct appdata {
float4 vertex : POSITION;
float2 uv : TEXCOORD0;
};
struct v2f {
float2 uv : TEXCOORD0;
float4 vertex : SV_POSITION;
};
sampler2D _MainTex;
float _BlurStrength;
float _BlurRange;
v2f vert (appdata v) {
v2f o;
o.vertex = UnityObjectToClipPos(v.vertex);
o.uv = v.uv;
return o;
}
fixed4 frag (v2f i) : SV_Target {
fixed4 texColor = tex2D(_MainTex, i.uv);
float4 blurColor = texColor;
// 计算距离图片中心的距离
float2 center = float2(0.5, 0.5);
float distance = distance(i.uv, center);
// 根据距离计算当前像素的虚化程度
float blurAmount = lerp(1, 0, smoothstep(0.5 - _BlurRange, 0.5 + _BlurRange, distance));
blurAmount = pow(blurAmount, _BlurStrength);
// 使用高斯模糊算法对当前像素进行虚化
blurColor += tex2D(_MainTex, i.uv + float2(-0.01, -0.01)) * 0.05;
blurColor += tex2D(_MainTex, i.uv + float2(0.01, 0.01)) * 0.05;
blurColor += tex2D(_MainTex, i.uv + float2(-0.01, 0.01)) * 0.05;
blurColor += tex2D(_MainTex, i.uv + float2(0.01, -0.01)) * 0.05;
blurColor *= 0.2;
blurColor = lerp(texColor, blurColor, blurAmount);
return blurColor;
}
ENDCG
}
}
FallBack "Diffuse"
}
```
在这个Shader中,我们定义了两个可调节的参数 `_BlurStrength` 和 `_BlurRange`,分别对应虚化的强度和范围。在`frag`函数中,我们首先计算了当前像素距离图片中心的距离,并根据距离计算了当前像素的虚化程度。然后,我们使用高斯模糊算法对当前像素进行虚化,并将虚化后的颜色与原始颜色进行插值,得到最终的颜色。
使用这个Shader很简单,只需要将它应用到需要虚化的对象上,并在材质中手动调节 `_BlurStrength` 和 `_BlurRange` 参数即可。
相关推荐
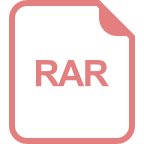
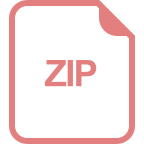














