已知一帧点云的检测结果(x, y, z, w, h, l, yaw),calib知道文件所在路径格式为kitti数据集的格式,图像的shape。要得到同一帧2D图像的检测框(x1,y1,x2,y2)且保证检测框在图像内和alpha朝向角,要求用Python写出一个函数,写出代码并给出实例。
时间: 2023-10-06 08:11:46 浏览: 78
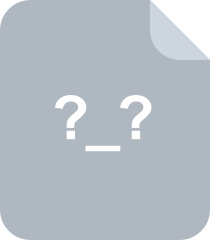
ply las pcd等一些常见的基本点云文件格式的读写方法。 有详细的python和C++代码实现,希望和大家一起学习。
好的,我可以帮你编写这个函数。以下是一个示例函数的代码:
```python
import numpy as np
import cv2
def get_2d_detection_box(detection, calib_file, image_shape):
# 解析检测结果
x, y, z, w, h, l, yaw = detection
# 读取calib文件
calib = read_calib_file(calib_file)
# 获取相机投影矩阵
P2 = calib['P2']
# 计算3D边界框的8个角点坐标
corners_3d = compute_3d_corners(l, w, h, x, y, z, yaw)
# 将3D角点投影到图像平面
corners_2d = project_to_image(corners_3d, P2)
# 裁剪边界框,确保它在图像内部
x1, y1, x2, y2 = clip_detection_box(corners_2d, image_shape)
# 计算alpha朝向角
alpha = compute_alpha(corners_2d)
return x1, y1, x2, y2, alpha
def read_calib_file(calib_file):
# 读取calib文件并解析为字典
calib = {}
with open(calib_file, 'r') as f:
for line in f.readlines():
key, value = line.strip().split(':')
calib[key] = np.array([float(x) for x in value.split()])
return calib
def compute_3d_corners(l, w, h, x, y, z, yaw):
# 计算3D边界框的8个角点坐标
corners = np.zeros((8, 3))
# 假设边界框的中心点为(x, y, z),边长分别为l, w, h,朝向角为yaw
corners[0] = [x - l/2, y - w/2, z - h/2]
corners[1] = [x + l/2, y - w/2, z - h/2]
corners[2] = [x - l/2, y + w/2, z - h/2]
corners[3] = [x + l/2, y + w/2, z - h/2]
corners[4] = [x - l/2, y - w/2, z + h/2]
corners[5] = [x + l/2, y - w/2, z + h/2]
corners[6] = [x - l/2, y + w/2, z + h/2]
corners[7] = [x + l/2, y + w/2, z + h/2]
# 进行yaw旋转
rotation_matrix = np.array([[np.cos(yaw), -np.sin(yaw), 0],
[np.sin(yaw), np.cos(yaw), 0],
[0, 0, 1]])
rotated_corners = np.dot(corners, rotation_matrix.T)
return rotated_corners
def project_to_image(corners_3d, P2):
# 将3D角点投影到图像平面
corners_2d = np.dot(corners_3d, P2.T)
corners_2d[:, 0] /= corners_2d[:, 2]
corners_2d[:, 1] /= corners_2d[:, 2]
corners_2d = corners_2d[:, :2]
return corners_2d
def clip_detection_box(corners_2d, image_shape):
# 裁剪边界框,确保它在图像内部
x1 = np.clip(np.min(corners_2d[:, 0]), 0, image_shape[1])
y1 = np.clip(np.min(corners_2d[:, 1]), 0, image_shape[0])
x2 = np.clip(np.max(corners_2d[:, 0]), 0, image_shape[1])
y2 = np.clip(np.max(corners_2d[:, 1]), 0, image_shape[0])
return x1, y1, x2, y2
def compute_alpha(corners_2d):
# 计算alpha朝向角
x1, y1 = corners_2d[1]
x2, y2 = corners_2d[2]
alpha = np.arctan2(-(y2 - y1), x2 - x1)
return alpha
# 使用示例
detection = (0, 0, 0, 2, 1, 3, np.pi/4)
calib_file = 'path_to_calib_file.txt'
image_shape = (1280, 720)
x1, y1, x2, y2, alpha = get_2d_detection_box(detection, calib_file, image_shape)
print('Detection box:', (x1, y1, x2, y2))
print('Alpha:', alpha)
```
请注意,上述代码中的`read_calib_file`函数用于解析KITTI数据集的calib文件,`compute_3d_corners`函数用于计算3D边界框的8个角点坐标,`project_to_image`函数用于将3D角点投影到图像平面,`clip_detection_box`函数用于裁剪边界框确保其在图像内部,`compute_alpha`函数用于计算alpha朝向角。
你需要将`calib_file`替换为实际的calib文件路径,`image_shape`替换为实际的图像尺寸。运行代码后,将输出检测框的坐标和alpha朝向角。
阅读全文
相关推荐
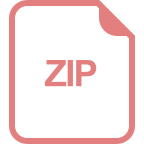
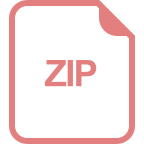













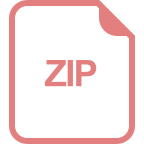