针对七巧板问题,设计存储形式,编程序完成涂色设计。
时间: 2023-12-25 11:03:49 浏览: 29
针对七巧板问题,可以采用一个二维数组来存储七巧板的形状,其中1表示该位置需要被涂色,0表示该位置不需要被涂色。例如,可以用以下方式存储七巧板的形状:
```
board = [
[0, 0, 1, 1, 0, 0],
[0, 1, 1, 1, 1, 0],
[1, 1, 1, 1, 1, 1],
[1, 1, 1, 1, 1, 1],
[0, 1, 1, 1, 1, 0],
[0, 0, 1, 1, 0, 0]
]
```
然后,可以编写一个递归函数来完成七巧板的涂色设计。函数的参数包括当前需要涂色的方格位置、当前已经涂过色的方格数量、以及已经涂色的颜色列表。具体实现如下:
```
colors = ['red', 'blue', 'green', 'yellow']
def paint(board, row, col, count, used_colors):
if count == sum(sum(board)):
return True
for color in colors:
if color in used_colors:
continue
if (row > 0 and board[row-1][col]) or \
(col > 0 and board[row][col-1]) or \
(row < len(board)-1 and board[row+1][col]) or \
(col < len(board[0])-1 and board[row][col+1]):
continue
board[row][col] = color
if paint(board, row, col+1, count+1, used_colors.union(set([color]))):
return True
if paint(board, row+1, col, count+1, used_colors.union(set([color]))):
return True
if paint(board, row, col-1, count+1, used_colors.union(set([color]))):
return True
if paint(board, row-1, col, count+1, used_colors.union(set([color]))):
return True
board[row][col] = 0
return False
paint(board, 0, 0, 0, set())
```
在上述代码中,我们首先定义了颜色列表colors,然后定义了递归函数paint,该函数的参数包括需要涂色的方格位置(row, col)、已经涂过色的方格数量count、以及已经涂色的颜色列表used_colors。在函数中,我们首先判断是否已经将所有方格都涂过色,如果是,则返回True。否则,我们枚举所有可以使用的颜色,如果该颜色已经被使用过,则跳过该颜色。接着,我们判断当前位置是否可以使用该颜色,如果不行,则跳过该颜色。如果可以使用该颜色,则将该颜色涂在当前位置,并继续递归调用paint函数,分别尝试涂色当前位置的右、下、左、上四个方向。如果某个方向成功涂色,则返回True。如果所有方向都不能成功涂色,则将当前位置的颜色清除,并返回False。
最后,我们调用paint函数,以左上角的方格作为起始点开始尝试涂色。如果成功涂色,则七巧板被涂色完成。
相关推荐
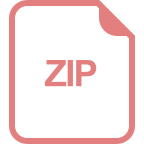














