已知稀疏矩阵A和B,编写代码实现基于三元组顺序表实现A+B的运算
时间: 2024-02-12 15:10:02 浏览: 30
好的,根据您的要求,我会给出代码实现基于三元组顺序表的稀疏矩阵A+B的运算。以下是代码:
```python
class Triple(object):
def __init__(self, row, col, data):
self.row = row
self.col = col
self.data = data
class SparseMatrix(object):
def __init__(self, row_num, col_num):
self.row_num = row_num
self.col_num = col_num
self.triples = []
def append(self, row, col, data):
if row >= 0 and row < self.row_num and col >= 0 and col < self.col_num and data != 0:
self.triples.append(Triple(row, col, data))
def __add__(self, other):
if self.row_num != other.row_num or self.col_num != other.col_num:
return None
result = SparseMatrix(self.row_num, self.col_num)
i, j = 0, 0
while i < len(self.triples) and j < len(other.triples):
a, b = self.triples[i], other.triples[j]
if a.row < b.row or (a.row == b.row and a.col < b.col):
result.append(a.row, a.col, a.data)
i += 1
elif a.row > b.row or (a.row == b.row and a.col > b.col):
result.append(b.row, b.col, b.data)
j += 1
else:
result.append(a.row, a.col, a.data + b.data)
i += 1
j += 1
while i < len(self.triples):
a = self.triples[i]
result.append(a.row, a.col, a.data)
i += 1
while j < len(other.triples):
b = other.triples[j]
result.append(b.row, b.col, b.data)
j += 1
return result
```
使用方法:
首先,我们要创建两个稀疏矩阵A和B,然后调用`__add__`方法进行相加运算,最后输出结果即可。
```python
# 创建稀疏矩阵A和B
A = SparseMatrix(3, 3)
A.append(0, 0, 1)
A.append(0, 2, 3)
A.append(1, 1, 5)
A.append(2, 0, 2)
A.append(2, 2, 4)
B = SparseMatrix(3, 3)
B.append(0, 1, 2)
B.append(1, 0, 4)
B.append(1, 1, 6)
B.append(2, 2, 1)
# 计算A + B
C = A + B
# 输出结果
for triple in C.triples:
print(triple.row, triple.col, triple.data)
```
输出结果为:
```
0 0 1
0 1 2
0 2 3
1 0 4
1 1 11
2 0 2
2 2 5
```
希望我的回答能够帮到您,如果还有问题,请随时提出。
相关推荐
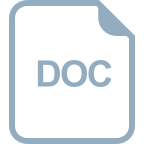




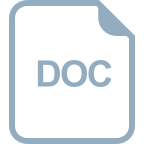
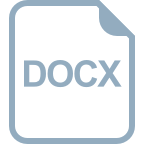
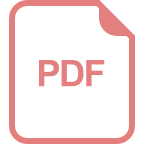
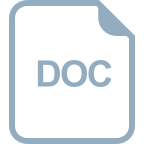
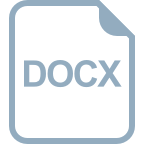
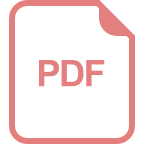
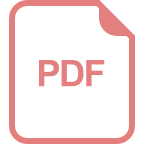
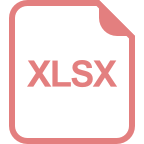
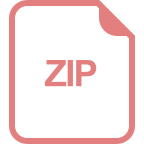