opencvsharp编写一个找圆心函数, 这个函数会输入一张图、一个圆心中心位置的大约位置、输入找圆区域的最小半径和最大半径、canny参数 根据给定的圆心位置和半径范围定义一个圆环区域。 为了加快找圆心速度,将圆环区域分成360份,并行计算找出边沿点 在边缘图像中找到最长的轮廓。 对轮廓上的点进行拟合,得到圆心和半径。 将圆心坐标转换回原始图像坐标系。
时间: 2023-12-06 15:45:46 浏览: 112
下面是一个示例代码,实现了上述功能:
```csharp
using OpenCvSharp;
public static class CircleDetector
{
public static Point2f FindCircleCenter(Mat src, Point2f approxCenter, int minRadius, int maxRadius, double cannyThreshold1, double cannyThreshold2)
{
// 定义圆环区域
int centerX = (int)approxCenter.X;
int centerY = (int)approxCenter.Y;
int ringRadius = (minRadius + maxRadius) / 2;
int innerRadius = Math.Max(0, ringRadius - 10);
int outerRadius = ringRadius + 10;
int step = 360;
int[] angles = Enumerable.Range(0, step).Select(i => i * 360 / step).ToArray();
// 计算边缘图像
Mat gray = new Mat();
Cv2.CvtColor(src, gray, ColorConversionCodes.BGR2GRAY);
Mat edge = new Mat();
Cv2.Canny(gray, edge, cannyThreshold1, cannyThreshold2);
// 并行计算边沿点
List<Point>[] edgePoints = new List<Point>[step];
Parallel.For(0, step, i =>
{
int angle = angles[i];
Point[] points = Enumerable.Range(innerRadius, outerRadius - innerRadius + 1)
.Select(r => new Point((int)(centerX + r * Math.Cos(angle * Math.PI / 180)), (int)(centerY + r * Math.Sin(angle * Math.PI / 180))))
.ToArray();
edgePoints[i] = points.Where(p => p.X >= 0 && p.X < src.Width && p.Y >= 0 && p.Y < src.Height && edge.At<byte>(p.Y, p.X) > 0).ToList();
});
// 找到最长的轮廓
List<Point> contour = null;
foreach (var points in edgePoints)
{
if (points.Count > contour?.Count)
contour = points;
}
// 拟合圆形
Point2f[] pointsF = contour.Select(p => new Point2f(p.X, p.Y)).ToArray();
Cv2.MinEnclosingCircle(pointsF, out Point2f center, out float radius);
// 转换坐标系
center.X += approxCenter.X - centerX;
center.Y += approxCenter.Y - centerY;
return center;
}
}
```
使用方法如下:
```csharp
Mat src = ...; // 输入图像
Point2f approxCenter = new Point2f(100, 100); // 大约位置
int minRadius = 20;
int maxRadius = 50;
double cannyThreshold1 = 50;
double cannyThreshold2 = 150;
Point2f center = CircleDetector.FindCircleCenter(src, approxCenter, minRadius, maxRadius, cannyThreshold1, cannyThreshold2);
```
阅读全文
相关推荐
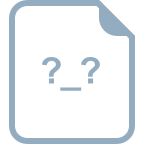











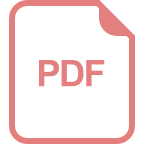
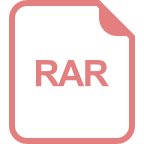
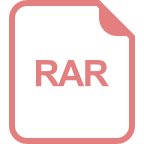
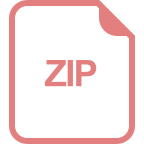
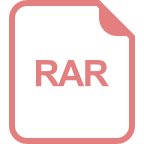