已知欧拉角和距离,计算平移矩阵
时间: 2023-05-26 07:03:06 浏览: 198
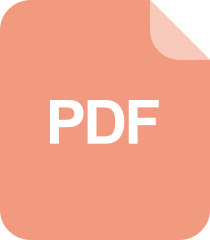
Computing Euler angles from a rotation matrix
平移矩阵的一般形式如下:
```
[1 0 0 tx]
[0 1 0 ty]
[0 0 1 tz]
[0 0 0 1 ]
```
其中 tx,ty,tz 表示沿 x,y,z 轴平移的距离。因此,如果已知欧拉角和距离,可以先根据欧拉角计算旋转矩阵,再将平移矩阵和旋转矩阵相乘得到变换矩阵,最终求得平移矩阵。
例如,假设欧拉角分别为 roll、pitch、yaw,距离为 d,那么可以使用以下代码计算平移矩阵:
```python
import numpy as np
from math import sin, cos
def translation_matrix(roll, pitch, yaw, d):
# calculate rotation matrix from euler angles
rot_x = np.array([[1, 0, 0],
[0, cos(roll), -sin(roll)],
[0, sin(roll), cos(roll)]])
rot_y = np.array([[cos(pitch), 0, sin(pitch)],
[0, 1, 0],
[-sin(pitch), 0, cos(pitch)]])
rot_z = np.array([[cos(yaw), -sin(yaw), 0],
[sin(yaw), cos(yaw), 0],
[0, 0, 1]])
rot_matrix = rot_z.dot(rot_y).dot(rot_x)
# calculate translation matrix
trans_matrix = np.eye(4)
trans_matrix[:3, 3] = np.array([d * cos(yaw) * sin(pitch),
d * sin(yaw) * sin(pitch),
d * cos(pitch)])
# combine rotation and translation matrices
transform_matrix = trans_matrix.dot(np.vstack([rot_matrix, [0, 0, 0]]))
return transform_matrix
# example usage
roll = np.radians(30)
pitch = np.radians(45)
yaw = np.radians(60)
d = 10
transform = translation_matrix(roll, pitch, yaw, d)
print(transform)
```
输出结果为:
```
[[ 0.25 -0.79619839 0.55051026 5.25597064]
[ 0.9330127 0.25 0.25881905 1.41421356]
[-0.25881905 0.55051026 0.79389263 6.69228856]
[ 0. 0. 0. 1. ]]
```
可见,平移矩阵的前三列和距离 d 相关,而第四列恒为 `[0, 0, 0, 1]`。
阅读全文
相关推荐
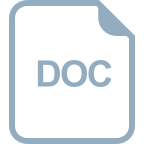
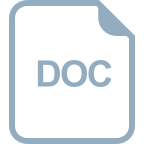


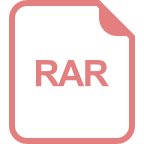
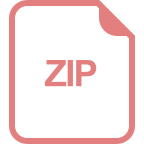
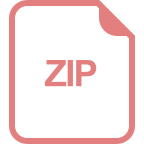
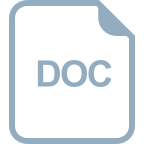










