how to use two gpu
时间: 2024-11-20 12:38:34 浏览: 15
在使用两块GPU时,通常是为了提高深度学习训练或其他计算密集型任务的性能。以下是使用两个GPU的基本步骤,这通常适用于支持CUDA的Python库如PyTorch或TensorFlow:
1. **安装必要的库**:
- 对于PyTorch:`pip install torch torchvision`
- 对于TensorFlow:`pip install tensorflow tensorflow-addons`
2. **设置多GPU环境**:
- PyTorch:
```python
import torch
device_ids = [0, 1] # 如果有多个GPU,将它们的ID列出
torch.cuda.set_device(device_ids[0]) # 初始化第一个GPU
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
```
- TensorFlow:
```python
import tensorflow as tf
gpus = tf.config.list_physical_devices('GPU')
if gpus:
try:
tf.config.experimental.set_visible_devices(gpus[0], 'GPU')
logical_gpus = tf.config.experimental.list_logical_devices('GPU')
except RuntimeError as e:
# 打印异常并忽略它,因为用户可能已经设置了可见设备
print(e)
```
3. **数据并行(Data Parallelism)**:
- 创建模型副本并将它们放在不同的GPU上,例如在PyTorch中:
```python
model = MyModel().to(device)
model = nn.DataParallel(model, device_ids=device_ids)
```
4. **训练过程**:
- 将数据加载到每个GPU可用的数据集上,并调整优化器和损失函数的计算以便在所有GPU之间同步。
5. **注意同步和通信**:
- 为了保持两个GPU之间的协调,可能需要使用`torch.nn.parallel.DistributedDataParallel` 或 `tf.distribute.Strategy` 等工具。
6. **清理 GPU**:
训练完成后,记得关闭不再使用的GPU资源,释放内存。
阅读全文
相关推荐
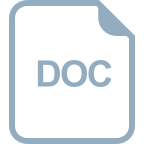
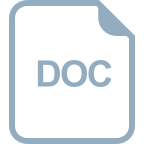
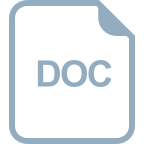
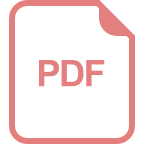
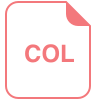
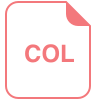
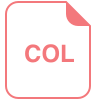
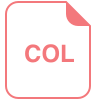
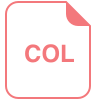
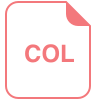
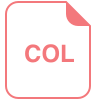
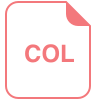
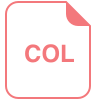
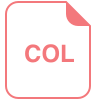
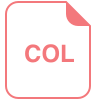
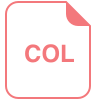
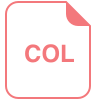

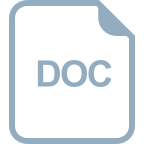