Enhance VSCode Features: Version Control, Auto Save, and Multiple Windows
发布时间: 2024-09-15 08:24:08 阅读量: 32 订阅数: 45 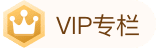
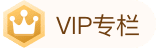
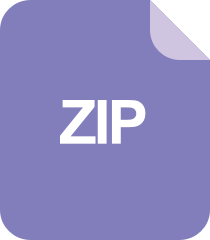
java计算器源码.zip
# 1.1 Basic Operations of Git
Git integration is a powerful feature in VSCode that allows developers to manage code version directly in the editor. VSCode provides comprehensive support for Git, including:
- **Initialize a Git repository:** Use the `git init` command to initialize a new Git repository in the current directory.
- **Add and commit changes:** Use the `git add` and `git commit` commands to add changes to the staging area and commit them to the local repository.
- **Push and pull changes:** Use the `git push` and `git pull` commands to push changes to a remote repository or pull changes from a remote repository.
- **View history:** Use the `git log` command to view commit history, including author, commit time, and commit message.
# 2. Version Control and Collaboration
### 2.1 Git Integration
#### 2.1.1 Basic Operations of Git
VSCode seamlessly integrates with the Git version control system, allowing developers to perform common Git operations directly in the IDE.
**Initialize a Git Repository:**
```
git init
```
**Add Files to Staging Area:**
```
git add <filename>
```
**Commit Changes:**
```
git commit -m "<commit message>"
```
**Push Changes to Remote Repository:**
```
git push origin <branch>
```
**Pull Changes from Remote Repository:**
```
git pull origin <branch>
```
#### 2.1.2 Git Collaboration Workflow
VSCode offers a wealth of features to support the Git collaboration workflow, including:
- **Code Review:** View and comment on code change requests, and collaborate with team members.
- **Conflict Resolution:** Detect and resolve conflicts while merging code.
- **Branch Management:** Create, switch, and merge branches to manage different development flows.
### 2.2 Other Version Control Systems
In addition to Git, VSCode also supports other version control systems, including:
#### 2.2.1 SVN
**Initialize an SVN Repository:**
```
svn init <repository path>
```
**Add Files to Repository:**
```
svn add <filename>
```
**Commit Changes:**
```
svn commit -m "<commit message>"
```
#### 2.2.2 Mercurial
**Initialize a Mercurial Repository:**
```
hg init
```
**Add Files to Repository:**
```
hg add <filename>
```
**Commit Changes:**
```
hg commit -m "<commit message>"
```
### 2.2.3 Comparison of Version Control Systems
| Feature | Git | SVN | Mercurial |
|---|---|---|---|
| Distributed | Yes | No | Yes |
| History | Non-linear | Linear | Non-linear |
| Branch Management | Powerful | Basic | Powerful |
| Conflict Resolution | Manual | Automatic | Manual |
| Community Support | Vast | Stable | Active |
**Table 1: Comparison of Version Control Systems**
### 2.2.4 Choosing a Version Control System
The choice of version control system depends on the specific needs of the project. Git is suitable for distributed development and frequent branch merging, while SVN and Mercurial are more suitable for centralized development and simple history management.
# 3. Auto Save and Recovery
### 3.1 Auto Save Mechanism
VSCode offers an auto save mechanism that automatically saves changes to the disk while the user is editing code. This helps prevent data loss due to unexpected events such as system crashes or power outages.
#### 3.1.1 Configure Auto Save Settings
The auto save feature is enabled by default, but users can configure it through the following steps:
1. Open VSCode Settings (File > Preferences > Settings).
2. Search for "auto save" in the search bar.
3. Find the "Files: Auto Save" setting.
4. Select one of the following options based on your needs:
- **Off (Never)**: Disable auto save.
- **On (Focus Change)**: Auto save when the user switches to other windows or applications.
- **On (Window Change)**: Auto save when the user closes or switches VSCode windows.
#### 3.1.2 The Principle of Auto Save
VSCode uses the following mechanisms to implement auto save:
1. **File Watcher:** VSCode monitors files edited by the user and detects any changes.
2. **Incremental Save:** When changes are detected, VSCode only saves the changed parts, not the entire file. This can improve save speed and reduce disk I/O.
3. **Periodic Save:** Even if the user does not make any edits, VSCode periodically saves open files. This helps prevent data loss due to system crashes or power outages.
### 3.2 Recover Unsaved Changes
If VSCode closes or crashes unexpectedly, users can recover unsaved changes.
#### 3.2.1 Recover Lost Files
If the user did not save the file before VSCode crashed, they can try to recover from the following locations:
- **Temporary Files:** VSCode creates temporary files in the user's directory, which contain unsaved changes. These files usually end with ".vscode-unsaved".
- **Backup Files:** VSCode may also create backup files that contain previous versions of saved files. These files usually end with ".vscode-backup".
Users can manually search for these files and copy them to a new file.
#### 3.2.2 Recover State After Crash
If VSCode crashes, users can recover the state before the crash when reopening. VSCode will automatically attempt to recover the following:
- Opened files
- Changes in the editor
- Debug sessions
- Terminal sessions
If recovery fails, users can try the following steps:
1. Open VSCode Settings (File > Preferences > Settings).
2. Search for "window.reopenFolders" in the search bar.
3. Ensure "window.reopenFolders" is set to "true".
4. Restart VSCode.
# 4. Multiple Windows and Workspace Management
### 4.1 Multiple Window Operations
#### 4.1.1 Create and Manage Multiple Windows
In VSCode, multiple windows can be created and managed, each with its own files, editors, and settings. To create a new window, you can:
- Press `Ctrl` + `N` (Windows/Linux) or `Cmd` + `N` (macOS).
- Click on the "File" > "New Window" in the menu bar.
After creating multiple windows, you can switch between them using:
- Use `Ctrl` + `Tab` (Windows/Linux) or `Cmd` + `Tab` (macOS) to cycle through open windows.
- Click on the window title bar to select a specific window.
#### 4.1.2 Synchronize and Share Data
By default, multiple windows in VSCode are independent and do not share data. However, the "Sync Settings" feature can be enabled to synchronize settings, extensions, and themes between windows. To enable this feature, follow these steps:
1. Click on "File" > "Preferences" > "Settings" in the menu bar.
2. Search for "Sync Settings" in the search bar.
3. Check the "Sync Settings" option.
Once "Sync Settings" is enabled, VSCode will synchronize the following data between all logged-in windows:
- User settings (`.vscode/settings.json` file)
- Installed extensions
- Installed themes
- Keyboard shortcuts
### 4.2 Workspace Management
#### 4.2.1 Create and Switch Workspaces
A workspace in VSCode is a collection of related files. Multiple workspaces can be created, each with its own settings and extensions. To create a new workspace, you can:
- Press `Ctrl` + `K` + `O` (Windows/Linux) or `Cmd` + `K` + `O` (macOS).
- Click on the "File" > "Open Folder" in the menu bar.
After selecting a folder, VSCode will create a new workspace containing all the files in that folder and its subfolders.
To switch to another workspace, you can:
- Use `Ctrl` + `K` + `T` (Windows/Linux) or `Cmd` + `K` + `T` (macOS) to cycle through open workspaces.
- Click on the workspace name in the window title bar to select a specific workspace.
#### 4.2.2 Workspace Settings and Extensions
Each workspace can have its own settings and extensions. Workspace settings are stored in the `.vscode/settings.json` file, while workspace extensions are stored in the `.vscode/extensions.json` file.
To modify workspace settings, follow these steps:
1. Click on "File" > "Preferences" > "Settings" in the menu bar.
2. Search for "workspace settings" in the search bar.
3. Switch between "User Settings" and "Workspace Settings".
4. Modify the desired settings.
To install workspace extensions, follow these steps:
1. Click on "View" > "Extensions" in the menu bar.
2. Search for the extension name in the search bar.
3. Click the "Install" button.
Installed extensions will only be available in the current workspace.
# 5. VSCode Extension Ecosystem
### 5.1 Extension Installation and Management
#### 5.1.1 Extension Marketplace
VSCode has a vast extension marketplace, offering various functionalities, including code editing enhancements, version control tools, debugging, and analysis. Users can browse and install extensions through the extension marketplace.
**Accessing the Extension Marketplace:**
- In VSCode, click on the extension icon (gear-shaped) in the left sidebar.
- In the extensions tab, click the "Browse Marketplace" button.
**Installing Extensions:**
- In the extension marketplace, search for the desired extension.
- Click on the extension name to view details.
- Click the "Install" button to install the extension.
#### 5.1.2 Installation and Updates of Extensions
**Installing Extensions:**
- Install extensions through the extension marketplace as described above.
- Download the .vsix file of the extension from the Visual Studio Code official website. Double-click the .vsix file to install the extension.
**Updating Extensions:**
- In VSCode, click on the extension icon in the left sidebar.
- In the extensions tab, click the "Update" button.
- VSCode will automatically check for and update all installed extensions.
### 5.2 Extension Recommendations
#### 5.2.1 Code Editing Enhancements
- **Auto Close Tag**: Automatically close tags in HTML, XML, and JavaScript.
- **Bracket Pair Colorizer**: Colorize matching brackets to improve code readability.
- **ESLint**: Check the syntax and style of JavaScript code.
- **Prettier**: Automatically format code to ensure consistent code style.
#### 5.2.2 Version Control Tools
- **GitLens**: Provides detailed information about Git repositories, including commit history, branches, and merges.
- **GitHub Pull Requests**: View and manage GitHub pull requests directly in VSCode.
- **SVN**: Integrates the Subversion version control system.
- **Mercurial**: Integrates the Mercurial version control system.
#### 5.2.3 Debugging and Analysis
- **Debugger for Chrome**: Debug Chrome extensions and web applications in VSCode.
- **Node.js Debugger**: Debug Node.js applications in VSCode.
- **Python Debugger**: Debug Python applications in VSCode.
- **Call Hierarchy**: Displays the hierarchy of function calls, facilitating navigation and understanding of the code.
# 6. VSCode Advanced Tips
### 6.1 Custom Commands and Shortcuts
#### 6.1.1 Creating Custom Commands
Custom commands allow you to perform specific tasks without using menus or keyboard shortcuts. To create a custom command, follow these steps:
1. Open settings (File > Preferences > Settings).
2. Search for "command palette" in the search bar.
3. Click on the "Keyboard Shortcuts" tab.
4. Click the "+" button to create a new command.
5. Enter a name for the command in the "Command" field.
6. Enter a shortcut key in the "Key Bindings" field.
7. Click the "Add" button.
For example, to create a command named "Say Hello", you can use the following command:
```
{
"key": "ctrl+shift+h",
"command": "sayHello",
"args": []
}
```
#### 6.1.2 Modifying Shortcuts
VSCode allows you to modify existing shortcuts or create shortcuts for commands that do not have them. To modify shortcuts, follow these steps:
1. Open settings (File > Preferences > Settings).
2. Search for "command palette" in the search bar.
3. Click on the "Keyboard Shortcuts" tab.
4. Find the command you want to modify in the command list.
5. Enter a new shortcut key in the "Key Bindings" field.
6. Press the "Enter" key to save changes.
For example, to change the shortcut key for the "Save" command from "Ctrl+S" to "F2", you can add the following to your settings:
```
{
"key": "f2",
"command": "workbench.action.files.save"
}
```
### 6.2 Scripts and Automation
#### 6.2.1 Using the VSCode Extension API
The VSCode extension API allows you to create custom scripts and automate tasks. To use the extension API, follow these steps:
1. Install the `vscode-extension-api` package.
2. Create a new file and name it `extension.js`.
3. Write your script in the file.
4. Run the `npm start` command to start the extension.
For example, the following script will add a line of text to the current file:
```javascript
const vscode = require('vscode');
function activate(context) {
context.subscriptions.push(***mands.registerCommand('extension.sayHello', () => {
vscode.window.showInformationMessage('Hello, world!');
}));
}
function deactivate() {}
module.exports = {
activate,
deactivate
};
```
#### 6.2.2 Writing Custom Scripts
You can also write custom scripts to automate tasks in VSCode. To write custom scripts, follow these steps:
1. Create a new file and name it `script.js`.
2. Write your script in the file.
3. Use the `F1` command palette to run the script.
For example, the following script will open all files in the directory of the current file:
```javascript
const vscode = require('vscode');
function openAllFiles() {
const workspaceFolders = vscode.workspace.workspaceFolders;
if (workspaceFolders) {
for (const folder of workspaceFolders) {
vscode.workspace.openTextDocument(folder.uri).then((document) => {
vscode.window.showTextDocument(document);
});
}
}
}
openAllFiles();
```
0
0