Automating Build and Deployment of Frontend Projects in VSCode
发布时间: 2024-09-15 08:52:28 阅读量: 25 订阅数: 45 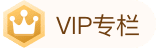
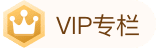
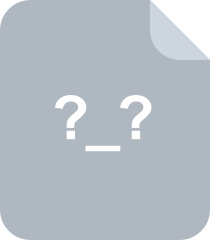
Automating and Testing a REST API epub
# 1. Overview of Automated Build and Deployment for Frontend Projects in VSCode
VSCode, as a powerful code editor, offers rich support for automating the build and deployment processes of frontend projects. By leveraging VSCode's built-in features and extensions, developers can streamline and optimize their development workflows, thereby enhancing productivity and code quality.
This chapter will outline the benefits of automating build and deployment for frontend projects in VSCode, including:
* Enhancing build and deployment efficiency by reducing manual operations
* Ensuring consistency and repeatability in the build and deployment process
* Promoting team collaboration and knowledge sharing
* Improving code quality and reliability
# 2. Automated Build for Frontend Projects in VSCode
### 2.1 Using Task Runner Explorer
#### 2.1.1 Creating and Configuring Tasks
In VSCode, Task Runner Explorer is a convenient tool for creating and managing build tasks. To create a task, navigate to the "Tasks" tab and click on "Create Task."
```json
{
"version": "2.0.0",
"tasks": [
{
"label": "build",
"type": "shell",
"command": "npm run build",
"args": [],
"problemMatcher": []
}
]
}
```
***label**: The name of the task, which will be displayed in Task Runner Explorer.
***type**: The type of the task, which can be "shell", "process", or "external".
***command**: The command to be run.
***args**: Arguments to be passed to the command (optional).
***problemMatcher**: A pattern used to parse problems from command output (optional).
#### 2.1.2 Running and Debugging Tasks
After creating a task, you can run it by clicking the "Run" button in Task Runner Explorer or by using the shortcut (by default, Ctrl+Shift+B). To debug a task, click the "Debug" button or use the shortcut (by default, F5). This will run the task in debug mode, allowing you to set breakpoints and step through the code.
### 2.2 Integration of Common Build Tools
#### 2.2.1 npm and yarn
npm and yarn are popular package managers used for managing dependencies in frontend projects. VSCode offers built-in support for npm and yarn, allowing you to run commands directly from the editor.
```json
{
"version": "2.0.0",
"tasks": [
{
"label": "install",
"type": "shell",
"command": "npm install",
"args": [],
"problemMatcher": []
},
{
"label": "build",
"type": "shell",
"command": "npm run build",
"args": [],
"problemMatcher": []
}
]
}
```
#### 2.2.2 webpack and Rollup
webpack and Rollup are module bundlers used for packaging and building JavaScript code. VSCode offers extensions for these tools, allowing you to configure and run build tasks directly from the editor.
```json
{
"version": "2.0.0",
"tasks": [
{
"label": "webpack",
"type": "webpack",
"webpack": {
"config": "./webpack.config.js"
},
"problemMatcher": []
},
{
"label": "rollup",
"type": "rollup",
"rollup": {
"input": "./src/main.js",
"output": {
"file": "./dist/main.js",
"format": "iife"
}
},
"problemMatcher": []
}
]
}
```
### 2.3 Optimization of the Build Process
#### 2.3.1 Caching and Parallel Builds
To optimize the build process, VSCode offers caching and parallel build functionality. Caching stores build results, thus avoiding the need to rebuild the same code repeatedly. Parallel builds allow multiple tasks to run simultaneously, reducing overall build time.
```json
{
"version": "2.0.0",
"tasks": [
{
"label": "build",
"type": "shell",
"command": "npm run build",
"args": [],
"problemMatcher": [],
"options": {
"cache": true,
"parallel": true
}
}
]
}
```
#### 2.3.2 Build Monitoring and Reporting
VSCode offers build monitoring and reporting features, allowing you to track build progress and identify potential issues. The build monitor displays the real-time status of a build, while the build report provides detailed information about the build results.
```json
{
"version": "2.0.0",
"tasks": [
{
"label": "build",
"type": "shell",
"command": "npm run build",
"args": [],
"problemMatcher
```
0
0
相关推荐


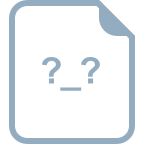
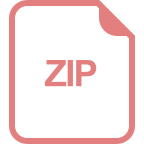
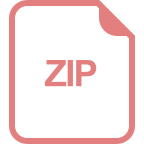
