Improving Technical Skills Through VSCode Extension Development
发布时间: 2024-09-15 08:38:44 阅读量: 22 订阅数: 36 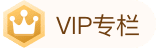
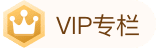
# Improving Technical Proficiency through VSCode Extension Development
## 1. Overview of VSCode Extensions
VSCode extensions are powerful tools that enhance the functionality of VSCode to better fit specific tasks or workflows. They allow developers to create custom features, integrate external tools, and automate repetitive tasks. Extensions can be tailored to meet the unique needs of each developer, improving productivity and efficiency.
The VSCode extension ecosystem is vast and active, offering a wide range of extensions that cover functionalities from code suggestions and debugging to version control and code generation. These extensions are developed by the community and distributed through the VSCode Marketplace, making it easy for developers to discover and install the extensions they need.
## 2. Basics of VSCode Extension Development
### 2.1 Setting Up the Development Environment
#### Requirements
- Node.js 16+
- Visual Studio Code 1.60+
#### Installing Dependencies
```bash
npm install -g yo generator-code
```
#### Creating an Extension Project
```bash
yo code
```
### 2.2 Introduction to Extension Manifest File
The extension manifest file `package.json` defines the basic information and configuration of the extension.
```json
{
"name": "my-extension",
"displayName": "My Extension",
"version": "1.0.0",
"publisher": "my-publisher",
"categories": ["Programming Languages"],
"activationEvents": ["onStartup", "onLanguage:javascript"],
"main": "./extension.js",
"contributes": {
"commands": [
{
"command": "extension.helloWorld",
"title": "Hello World"
}
]
}
}
```
| Parameter | Description |
|---|---|
| `name` | The unique identifier of the extension |
| `displayName` | The name displayed in VSCode |
| `version` | The version number of the extension |
| `publisher` | The publisher of the extension |
| `categories` | The categories of the extension |
| `activationEvents` | Events that trigger the activation of the extension |
| `main` | The main entry file of the extension |
| `contributes` | The extension's contributions to VSCode, such as commands, views, language support, etc. |
### 2.3 Extension Activation and Uninstallation Mechanism
#### Extension Activation
Extensions can be activated through the following events:
- `onStartup`: When VSCode starts up
- `onLanguage`: When a specific language file is opened
- `onView`: When a specific view is opened
- `onCommand`: When a specific command is executed
When an extension is activated, VSCode calls the `activate` function.
#### Extension Uninstallation
When a user uninstalls an extension, VSCode calls the `deactivate` function. In the `deactivate` function, the extension should release all resources, such as event listeners, Webview, etc.
## 3.1 Code Suggestions and Auto-Completion Extensions
#### 3.1.1 Extension Development Process
**1. Create an Extension Project**
Use the VSCode Extension Developer Kit (Yeoman generator) to create a new extension project:
```bash
yo code
```
**2. Configure the Extension Manifest File**
In the `package.json` file, configure the basic information of the extension, including the name, version, activation events, etc.
**3. Develop the Extension Code**
Create the extension code files in the `src` directory, for example `extension.ts`, and implement the extension logic.
**4. Debug the Extension**
Use VSCode's debugging feature to debug the extension code.
**5. Package the Extension**
Use the `vsce` tool to package the extension into a `.vsix` file.
#### 3.1.2 Practical Example: Code
0
0
相关推荐
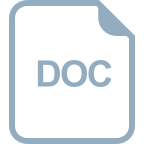
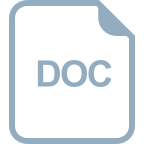
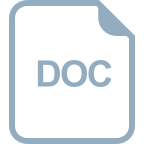
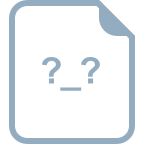
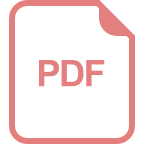
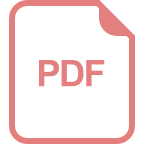
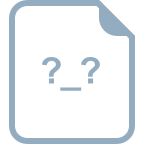
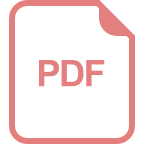
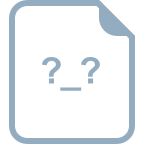