Solving Common Code Issues with VSCode Debugger
发布时间: 2024-09-15 08:21:46 阅读量: 26 订阅数: 29 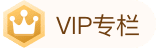
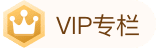
# Using VSCode Debugger to Solve Common Code Issues
## 1.1 Setting Breakpoints and Step Debugging
In VSCode, setting breakpoints is an effective method to pause code execution and inspect variable values and call stacks. To set a breakpoint, place the cursor on the left side of the line number and click the left mouse button. The breakpoint will be indicated by a red dot.
For step debugging, use the keyboard shortcuts F10 or F11 (for macOS, use Command + F10 or Command + F11). F10 executes code line by line, while F11 executes code function by function.
With step debugging, you can observe the code execution flow, identify errors, and understand the code logic.
## 2. Tips for Debugging Common Code Issues
### 2.1 Debugging Syntax Errors and Logical Errors
#### 2.1.1 Setting Breakpoints and Step Debugging
Setting a breakpoint is a way to pause execution at a specific line of code to inspect variable values and call stacks. In VSCode, breakpoints can be set by clicking on the left side of the code line.
**Code Block:**
```
# Set breakpoint
breakpoint()
```
**Logical Analysis:**
The `breakpoint()` function pauses the program when executed, allowing you to inspect variable values and call stacks.
#### 2.1.2 Inspecting Variable Values and Call Stacks
The call stack shows the current executing function and its call order. In VSCode, you can view the call stack by clicking on the "Call Stack" tab in the debug toolbar. Variable values can be inspected by entering the variable name in the debug console.
**Code Block:**
```
# Inspect variable value
print(variable_name)
```
**Logical Analysis:**
The `print()` function outputs the variable value to the console for inspection.
### 2.2 Debugging Runtime Errors
#### 2.2.1 Handling Exceptions and Errors
Exceptions are errors that occur during program execution. VSCode automatically catches exceptions and displays error messages. Exceptions can be handled using `try-except` blocks.
**Code Block:**
```
try:
# Code that might raise an exception
except Exception as e:
# Handle the exception
```
**Logical Analysis:**
The `try` block contains code that might raise an exception. If an exception occurs, the `except` block is executed and can handle the exception.
#### 2.2.2 Using Logs and Trace Statements
Logs and trace statements can help identify runtime errors. Log statements write messages to log files or the console, while trace statements print messages during code execution.
**Code Block:**
```
# ***
***("This is a log message")
# Trace statement
print("This is a trace message")
```
**Logical Analysis:**
The `logging` module is used for writing log messages, while the `print()` function is used for printing trace messages. These messages help identify errors and understand the code execution flow.
### 2.3 Debugging Performance Issues
#### 2.3.1 Analyzing Code Execution Time
Performance analysis tools can be used to analyze code execution time. VSCode offers a built-in profiler, which can be accessed by clicking on the "Performance" tab in the debug toolbar.
**Table: Profiler Features**
| Feature | Description |
|---|---|
| Profiling | Analyze code execution time and identify bottlenecks |
| Call Graph | Show function call relationships and identify hotspots |
| Memory Analysis | Analyze memory usage and identify memory leaks |
#### 2.3.2 Optimizing Algorithms and Data Structures
Performance issues are often caused by inefficient algorithms and data structures. Optimizing algorithms and data structures can improve code performance.
**Code Block:**
```
# Optimizing algorithm
def optimized_algorithm(data):
# Use a more efficient algorithm
return result
# Optimizing data structure
class OptimizedDataStructure:
# Use more efficient storage and retrieval methods
```
**Logical Analysis:**
The `optimized_algorithm()` function uses a more efficient algorithm, while the `OptimizedDataStructure` class uses more efficient storage and retrieval methods. These optimizations can improve code performance.
# 3.1 Debugging Python Code
#### 3.1.1 Installing Python Debug Extension
To debug Python code, you first need to install the Python debug extension. Open VSCode's extension market, search for "Python" and install the "Python" extension. After installation, restart VSCode to activate the extension.
#### 3.1.2 Setting Debug Configuration and Breakpoints
To debug Python code, you need to create a debug configuration. In VSCode, open the "Debug" view (Ctrl + Shift + D). Click the "Create New Configuration" button, then select "Python". In the "Debugger" field, select "Python".
Next, set breakpoints. A breakpoint is a point where the program pauses execution. To set a breakpoint, place the cursor next to the line number where you want to pause, and then click the blue circle next to the line number.
**Code Block: Setting Breakpoint**
```python
# Program code
def main():
x = 10
y = 20
z = x + y
print(z)
# Set breakpoint
breakpoint()
```
**Logical Analysis:**
This code block sets a breakpoint; when the `breakpoint()` statement is executed, the program will pause.
**Parameter Description:**
* `breakpoint()`: Sets a breakpoint.
**Code Block: Debugging Python Code**
```python
# Program code
def main():
x = 10
y = 20
z = x + y
print(z)
# Set breakpoint
breakpoint()
# Start debugging
import pdb
pdb.set_trace()
# Continue debugging
pdb.next()
pdb.step()
pdb.continue()
```
**Logical Analysis:**
This code block uses the `pdb` module for interactive debugging. The `pdb.set_trace()` statement pauses the program and opens an interactive debugging session. The `pdb.next()`, `pdb.step()`, and `pdb.continue()` commands are used to control the debugging session.
**Parameter Description:**
* `pdb.set_trace()`: Pauses the program and opens an interactive debugging session.
* `pdb.next()`: Executes the next line of code without entering a function.
* `pdb.step()`: Executes the next line of code and enters a function if one is called.
* `pdb.continue()`: Continues program execution until the next breakpoint or the end of the program.
# 4. Advanced Usage of the VSCode Debugger
### 4.1 Using Remote Debugging
#### 4.1.1 Connecting to a Remote Computer
Remote debugging allows you to debug code on a remote computer without running the code locally. This is useful for debugging code running on a server or other computers that are not directly accessible.
To connect to a remote computer, open the "Debug" view in VSCode (Ctrl + Shift + D), and then click the "Attach to Process" button. In the "Attach to Process" dialog box, select the "Remote" tab, and then enter the IP address or hostname of the remote computer.
#### 4.1.2 Debugging Remote Code
After connecting to the remote computer, you can debug remote code as if it were local code. You can set breakpoints, step through the code, and inspect variable values.
### 4.2 Using Custom Debuggers
#### 4.2.1 Creating Custom Debugger Configurations
VSCode allows you to create custom debugger configurations to support non-standard languages or tools. To create a custom debugger configuration, open the "launch.json" file, and then add a new debugger configuration.
```json
{
"version": "0.2.0",
"configurations": [
{
"name": "My Custom Debugger",
"type": "custom",
"request": "launch",
"program": "my_debugger",
"args": [
"--arg1",
"--arg2"
],
"cwd": "${workspaceFolder}"
}
]
}
```
In the above example, we created a custom debugger configuration named "My Custom Debugger". This configuration specifies the debugger program ("my_debugger"), arguments ("--arg1" and "--arg2"), and the working directory ("${workspaceFolder}").
#### 4.2.2 Extending Debug Features
Custom debugger configurations allow you to extend the capabilities of the VSCode debugger. You can add custom commands, variable views, and visualization tools to enhance the debugging experience.
### 4.3 Debugging Multithreaded and Concurrent Code
#### 4.3.1 Setting Thread Breakpoints
Multithreaded and concurrent code can be challenging to debug because multiple threads execute simultaneously. To debug multithreaded code, you can use VSCode's thread breakpoints.
To set a thread breakpoint, open the "Debug" view (Ctrl + Shift + D), and then click on the "Threads" tab. In the "Threads" tab, select the thread you want to set a breakpoint on, and then click the "Add Breakpoint" button.
#### 4.3.2 Visualizing Thread Execution
VSCode provides tools for visualizing thread execution. To visualize thread execution, open the "Debug" view (Ctrl + Shift + D), and then click on the "Threads" tab. In the "Threads" tab, select the "Show Thread Activity" button.
The "Thread Activity" view will display a graphical representation of thread execution. You can use this view to understand how threads interact and whether there are any deadlocks or race conditions.
# 5. Best Practices for Using the VSCode Debugger
### 5.1 Writing Debuggable Code
Writing debuggable code is a key step in the debugging process. Here are some best practices:
***Use clear variable names and comments:** Clear variable names and comments can help you easily understand the intention and behavior of the code.
***Break down complex code into modules:** Breaking down complex code into smaller modules can make debugging easier. Each module should focus on a specific task and be loosely coupled with other modules.
### 5.2 Using Debugging Tools for Continuous Integration
Continuous Integration (CI) pipelines can help automate the debugging process. Here are some best practices:
***Integrate debuggers into CI/CD pipelines:** Integrating debuggers into CI/CD pipelines can allow you to automatically run debug tests when code is committed.
***Automate the debugging process:** Automating the debugging process with scripts or tools can save time and increase efficiency. For example, you can use the `vscode-debug` library to write custom debug scripts.
### 5.3 Other Best Practices
In addition to the above best practices, there are other tips that can help you effectively use the VSCode debugger:
***Use conditional breakpoints:** Conditional breakpoints allow you to trigger a breakpoint only when certain conditions are met. This can help you focus on specific code paths or edge cases.
***Use watch expressions:** Watch expressions allow you to monitor changes in variable values. This can help you track the behavior of variables during code execution.
***Use the debug console:** The debug console allows you to input commands and expressions to interactively debug code. This can enable you to dynamically modify variable values or perform other debugging tasks.
***Use source code maps:** Source code maps allow you to view the original source code while debugging compiled code. This can make the debugging process more intuitive.
***Use debug extensions:** There are many debug extensions available in the VSCode Marketplace that can enhance debugging capabilities. For example, you can use the `Debugger for Chrome` extension to debug JavaScript code.
# 6. Future Developments for the VSCode Debugger
### 6.1 AI-Assisted Debugging
Artificial Intelligence (AI) technology is rapidly being integrated into various software development tools, and the VSCode debugger is no exception. In the future, AI will play an increasingly important role in the debugging process, helping developers solve problems more easily and efficiently.
#### 6.1.1 Automated Error Detection and Repair
AI algorithms can analyze code and automatically detect potential errors and issues. This will significantly reduce the time developers spend manually searching for errors, thereby increasing debugging efficiency. Additionally, AI can provide automatic repair suggestions to help developers quickly resolve problems.
#### 6.1.2 Smart Code Suggestions
AI technology can also provide smart code suggestions to help developers write more debuggable code. For example, AI algorithms can suggest optimal breakpoint locations, variable names, and comments, thereby simplifying the debugging process.
### 6.2 Cloud Debugging
The rise of cloud computing brings new possibilities for debuggers. In the future, the VSCode debugger will be able to remotely debug code on cloud platforms.
#### 6.2.1 Remotely Debugging Code on Cloud Platforms
Cloud debugging allows developers to debug code on remote servers or cloud platforms without the need to set up a complex debugging environment locally. This is particularly useful for debugging distributed systems, microservices, and containerized applications.
#### 6.2.2 Collaborative Debugging and Code Review
Cloud debugging also supports collaborative debugging and code review. Multiple developers can simultaneously connect to a remote debugging session, collaboratively solve problems, and review code. This can improve team collaboration efficiency and ensure code quality.
0
0
相关推荐
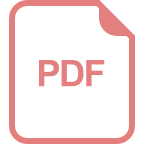
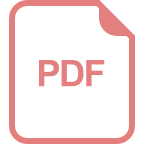
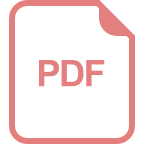
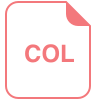
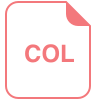
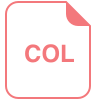
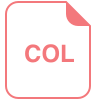
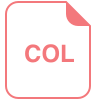
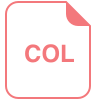