Efficient Use of Task Manager in VSCode
发布时间: 2024-09-15 08:45:13 阅读量: 26 订阅数: 37 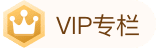
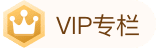
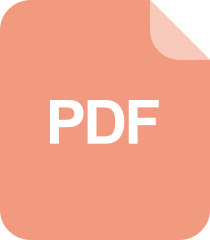
Efficient Use of Local Edge Histogram Descriptor
# Efficient Use of VSCode's Task Manager
VSCode's Task Manager is a powerful tool for automating various tasks within the VSCode editor. It allows developers to define and run custom tasks, such as compiling, running, debugging, testing, and formatting code. By utilizing the task manager, developers can streamline their workflow, boosting productivity and efficiency.
The Task Manager is located in the "Tasks" panel of VSCode, accessible via the "View" menu or the keyboard shortcut "Ctrl+Shift+B". It provides an intuitive interface that enables developers to create, configure, and run tasks. The task manager supports multiple languages and frameworks, including JavaScript, Python, Java, C++, and Go.
# Advanced Techniques in Task Management
### Creating and Configuring Tasks
#### Selecting Task Types and Setting Parameters
VSCode Task Manager supports various task types, including:
- **shell**: Executes shell commands in the terminal
- **process**: Runs executable files or scripts directly
- **npm**: Runs npm scripts
- **gulp**: Executes gulp tasks
- **grunt**: Executes grunt tasks
Each task type has specific parameters to configure its behavior. For example, parameters for a `shell` task include:
- **command**: The command to execute
- **args**: The list of arguments to pass to the command
- **cwd**: The working directory of the command
- **env**: A list of environment variables
#### Managing Environment Variables and Dependencies
Tasks can access VSCode's global environment variables or define their own. Environment variables can be set via the `env` parameter, for example:
```json
{
"label": "My Task",
"type": "shell",
"command": "echo",
"args": ["$MY_VAR"],
"env": {
"MY_VAR": "Hello World"
}
}
```
Tasks can also specify dependencies to ensure that other tasks run before them. Dependencies can be set via the `dependsOn` parameter, for example:
```json
{
"label": "Task 2",
"type": "shell",
"command": "echo",
"args": ["Task 1"],
"dependsOn": ["Task 1"]
}
```
### Running and Debugging Tasks
#### Executing Tasks and Controlling Output
Tasks can be executed in several ways:
- **Task Panel**: Open the task panel on the left side of VSCode and select the task.
- **Command Panel**: Press `Ctrl`+`Shift`+`P` to open the command panel, then type `task`.
- **Shortcut Keys**: Assign a shortcut key to a task and execute it by pressing the shortcut.
Task output can be controlled to filter and format the output. For example, parameters for a `shell` task include:
- **showOutput**: Specifies whether to display task output
- **echoCommand**: Specifies whether to show the command in task output
- **suppressTaskName**: Specifies whether to suppress the task name in task output
#### Debugging Task Settings and Usage
VSCode Task Manager supports task debugging, allowing the inspection of variables and code during task execution. To enable task debugging, set the `debug` parameter to `true` in the task configuration, for example:
```json
{
"label": "My Task",
"type": "shell",
"command": "echo",
"args": ["$MY_VAR"],
"debug": true
}
```
During task execution, variables and code can be inspected via the debugging tools.
### Automating and Integrating Tasks
#### Command-Line Invocation of Tasks
The Task Manager can be invoked via the command line to automate task execution. The command-line command is:
```
code --tasks
```
The task name can be passed as an argument to specify the task to be executed, for example:
```
code --tasks myTask
```
#### Integrating Tasks into Build Systems
Tasks can be integrated into build systems to automate the build process. For example, tasks can be integrated into a `gulp` build as follows:
```javascript
gulp.task('build', function() {
return gulp.src('src/*.js')
.pipe(gulp.dest('dist'));
});
gulp.task('test', function() {
return gulp.src('test/*.js')
.pipe(gulp.dest('dist'));
});
```
Then, tasks can be created in the VSCode task panel to call these `gulp` tasks, for example:
```json
{
"label": "Build",
"type": "gulp",
"task": "build"
}
```
# Practical Applications of Task Manager
### Compiling and Running Code with Task Manager
#### Configuring Compile Tasks for Different Languages
VSCode Task Manager supports configuring compile tasks for various programming languages, including Java, C++, Python, and JavaScript. The configuration methods for different languages vary slightly.
**Java Compile Task Configuration**
```json
{
"version": "2.0.0",
"tasks": [
{
"label": "javac",
"type": "java",
"command": "javac",
"args": ["-d", "${workspaceFolder}/bin", "${file}"],
"problemMatcher": "$java"
}
]
}
```
**Parameter Explanation:**
* `label`: The name of the task, displayed in the task list.
* `type`: The type of the task, here `java`.
* `command`: The compilation command, here `javac`.
* `args`: Arguments for the compilation command, specifying the output directory and source file here.
* `problemMatcher`: The problem
0
0
相关推荐
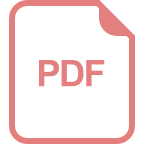
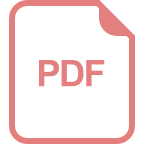





