图算法:揭开图论世界的神秘面纱,探索图论算法的奥秘
发布时间: 2024-08-25 06:28:55 阅读量: 9 订阅数: 11 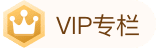
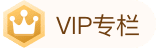
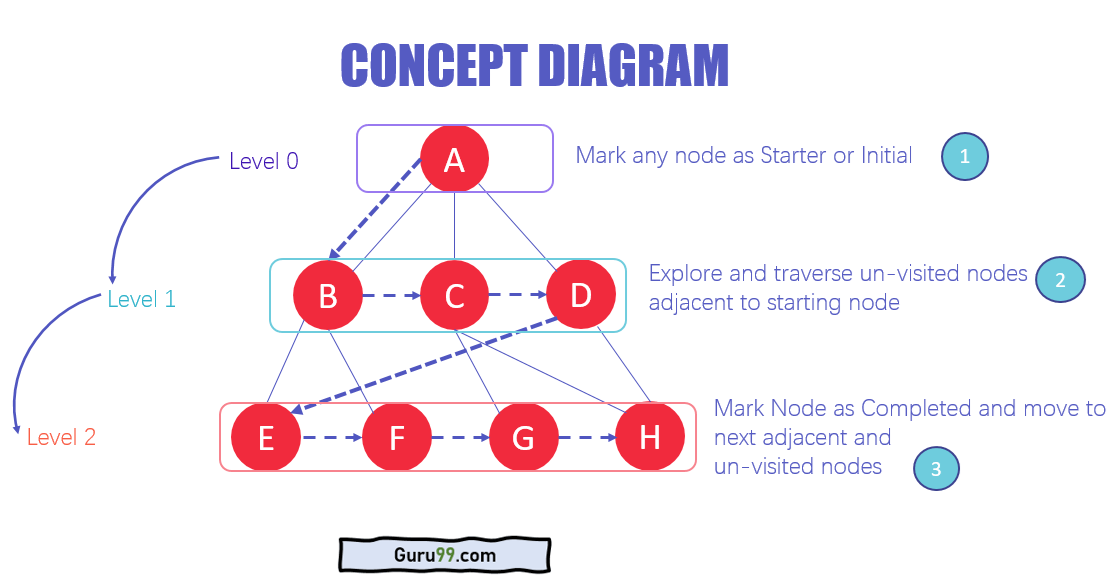
# 1. 图论基础**
图论是研究图结构及其性质的数学分支。图由一组称为顶点的元素和一组称为边的元素组成。顶点表示图中的对象,而边表示对象之间的关系。
图论基础包括图的基本概念,如顶点、边、度、路径、回路和连通分量。它还涉及图的表示方法,如邻接矩阵和邻接表。这些概念为理解图论算法和应用奠定了基础。
# 2. 图论算法基础
图论算法是计算机科学中用于解决图论问题的算法。图论问题涉及到由节点(顶点)和边组成的图结构。图论算法广泛应用于各种领域,包括网络、社交网络、交通网络和生物信息学。
### 2.1 深度优先搜索
#### 2.1.1 基本原理
深度优先搜索(DFS)是一种图论算法,它通过递归遍历图中的节点,沿着每个分支探索到最深处,然后再回溯到较浅的节点。DFS算法使用栈数据结构来跟踪已访问的节点,并确保每个节点只被访问一次。
#### 2.1.2 应用场景
DFS算法广泛应用于以下场景:
- **连通性检测:**确定图中是否所有节点都相互连接。
- **环检测:**检测图中是否存在环。
- **拓扑排序:**对无环图中的节点进行排序,使得每个节点都排在其所有后继节点之前。
### 2.2 广度优先搜索
#### 2.2.1 基本原理
广度优先搜索(BFS)是一种图论算法,它通过层序遍历图中的节点,首先访问所有与起始节点相邻的节点,然后再访问这些节点的相邻节点,以此类推。BFS算法使用队列数据结构来跟踪已访问的节点,并确保每个节点只被访问一次。
#### 2.2.2 应用场景
BFS算法广泛应用于以下场景:
- **最短路径:**找到图中两个节点之间的最短路径。
- **网络流:**最大化图中从源节点到汇节点的流量。
- **连通分量:**将图划分为连通的子图。
### 2.3 最小生成树算法
#### 2.3.1 克鲁斯卡尔算法
克鲁斯卡尔算法是一种最小生成树(MST)算法,它通过以下步骤构建图的MST:
1. 将图中的所有边按权重从小到大排序。
2. 从权重最小的边开始,依次添加边到MST中。
3. 如果添加的边会形成环,则跳过该边。
4. 重复步骤2和3,直到MST包含图中的所有节点。
#### 2.3.2 普里姆算法
普里姆算法是一种MST算法,它通过以下步骤构建图的MST:
1. 选择一个起始节点,将其添加到MST中。
2. 从MST中选择一个节点,并找到与该节点相邻的权重最小的边。
3. 如果添加的边不会形成环,则将其添加到MST中。
4. 重复步骤2和3,直到MST包含图中的所有节点。
### 2.4 最短路径算法
#### 2.4.1 迪杰斯特拉算法
迪杰斯特拉算法是一种最短路径算法,它通过以下步骤找到图中从源节点到所有其他节点的最短路径:
1. 将源节点的距离设为0,其他所有节点的距离设为无穷大。
2. 从距离最小的节点开始,将其所有相邻节点的距离更新为源节点到该节点的距离加上边权重。
3. 重复步骤2,直到所有节点的距离都被更新。
4. 最短路径为源节点到每个节点的距离。
#### 2.4.2 Floyd-Warshall算法
Floyd-Warshall算法是一种最短路径算法,它通过以下步骤找到图中任意两点之间的最短路径:
1. 初始化一个距离矩阵,其中每个元素表示两个节点之间的最短路径。
2. 对于每个中间节点,更新距离矩阵中所有元素,使得它们表示通过该中间节点的最短路径。
3. 重复步骤2,直到距离矩阵不再发生变化。
4. 距离矩阵中的元素表示任意两点之间的最短路径。
# 3.1 网络流算法
网络流算法是一类解决网络中流量分配问题的算法。网络中包含节点和边,节点代表流量的源点或汇点,边代表流量流动的路径。网络流算法的目标是找到从源点到汇点的最大流量或最小割。
### 3.1.1 最大流算法
最大流算法解决的是在给定网络中找到从源点到汇点的最大流量。最常用的最大流算法是福特-福尔克森算法。
#### 福特-福尔克森算法
福特-福尔克森算法通过不断寻找增广路径来增加网络的流量。增广路径是一条从源点到汇点的路径,其上的流量小于边的容量。
算法步骤如下:
```python
def ford_fulkerson(graph, source, sink):
# 初始化残余网络
residual_graph = graph.copy()
# 初始化最大流为 0
max_flow = 0
# 循环寻找增广路径
while True:
# 寻找增广路径
path = find_augmenting_path(residual_graph, source, sink)
# 如果没有增广路径,则退出循环
if not path:
break
# 计算增广路径上的最小流量
min_flow = min(residual_graph[edge[0]][edge[1]]['capacity'] for edge in path)
# 更新残余网络
for edge in path:
residual_graph[edge[0]][edge[1]]['capacity'] -= min_flow
residual_graph[edge[1]][edge[0]]['capacity'] += min_flow
# 更新最大流
max_flow += min_flow
return max_flow
```
#### 参数说明:
* `graph`: 输入网络,是一个字典,其中键是节点,值为字典,其中键是边,值为边的容量。
* `source`: 源点。
* `sink`: 汇点。
#### 代码逻辑分析:
1. 初始化残余网络为输入网络的副本。
2. 初始化最大流为 0。
3. 循环寻找增广路径。
4. 如果没有增广路径,则退出循环。
5. 计算增广路径上的最小流量。
6. 更新残余网络。
7. 更新最大流。
8. 返回最大流。
### 3.1.2 最小割算法
最小割算法解决的是在给定网络中
0
0
相关推荐
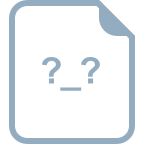
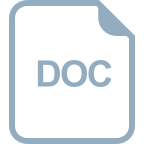
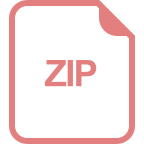
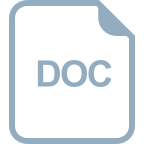
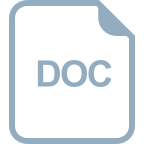
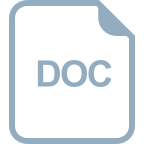
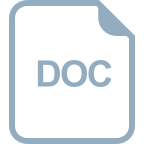
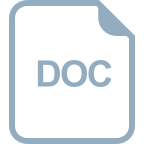
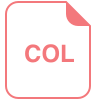