图论算法实战:探索图论世界的深度与广度
发布时间: 2024-08-23 23:59:31 阅读量: 10 订阅数: 18 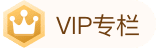
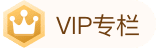
.png)
# 1. 图论算法基础
图论算法是计算机科学中用于解决与图相关问题的算法。图是一种数据结构,用于表示对象之间的关系。图论算法可以用来解决各种问题,例如查找最短路径、最大匹配和最小生成树。
### 1.1 图的基本概念和性质
图由一组顶点和一组边组成。顶点表示对象,边表示对象之间的关系。图可以是有向的或无向的。有向图的边具有方向,而无向图的边没有方向。
图的度是指顶点连接的边的数量。入度是指进入顶点的边的数量,出度是指从顶点发出的边的数量。
# 2. 图论算法的理论与实现
### 2.1 图论基础理论
#### 2.1.1 图的基本概念和性质
**图的定义**
图是一种数据结构,由顶点(点)和边(线)组成。顶点表示实体,边表示实体之间的关系。
**图的性质**
* **连通性:**如果图中任意两个顶点之间都存在路径,则该图是连通的。
* **环:**如果图中存在一条从某个顶点出发,经过若干条边后又回到该顶点的路径,则该图包含环。
* **权重:**边可以具有权重,表示边上关系的强度或成本。
* **有向图和无向图:**如果边具有方向,则该图是有向图;否则,该图是无向图。
#### 2.1.2 图的表示和存储
**邻接矩阵**
邻接矩阵是一个二维数组,其中元素表示顶点之间的权重。对于无向图,邻接矩阵是对称的。
**邻接表**
邻接表是一个数组,其中每个元素是一个链表,存储与该顶点相邻的顶点。
**十字链表**
十字链表是一种更紧凑的邻接表表示,其中每个顶点存储指向其相邻顶点的指针,以及指向其相邻边的指针。
### 2.2 图论算法的实现
#### 2.2.1 深度优先搜索(DFS)
**算法描述**
DFS 是一种遍历图的递归算法,从一个起始顶点开始,深度优先地探索其相邻顶点。
**代码实现**
```python
def dfs(graph, start):
"""深度优先搜索算法
Args:
graph: 图数据结构
start: 起始顶点
"""
visited = set() # 已访问的顶点集合
def dfs_helper(node):
if node in visited:
return
visited.add(node)
for neighbor in graph[node]:
dfs_helper(neighbor)
dfs_helper(start)
```
**逻辑分析**
* `visited` 集合用于跟踪已访问的顶点。
* `dfs_helper` 函数递归地访问相邻顶点。
* 算法时间复杂度为 O(V + E),其中 V 是顶点数,E 是边数。
#### 2.2.2 广度优先搜索(BFS)
**算法描述**
BFS 是一种遍历图的层序算法,从一个起始顶点开始,逐层探索其相邻顶点。
**代码实现**
```python
def bfs(graph, start):
"""广度优先搜索算法
Args:
graph: 图数据结构
start: 起始顶点
"""
queue = [start] # 队列用于存储待访问的顶点
visited = set() # 已访问的顶点集合
while queue:
node = queue.pop(0)
if node in visited:
continue
visited.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
queue.append(neighbor)
```
**逻辑分析**
* `queue` 队列用于存储待访问的顶点。
* 算法逐层遍历图,直到队列为空。
* 算法时间复杂度为 O(V + E),其中 V 是顶点数,E 是边数。
#### 2.2.3 最小生成树算法
**算法描述**
最小生成树算法用于寻找图中连接所有顶点的最小权重边集。
**代码实现**
```python
def prim(graph):
"""Prim 最小生成树算法
Args:
graph: 图数据结构
"""
# 初始化
visited = set() # 已访问的顶点集合
mst = [] # 最小生成树边集
current_node = next(iter(graph)) # 起始顶点
visited.add(current_node)
while len(visited) < len
```
0
0
相关推荐
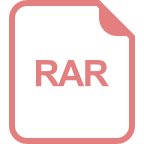
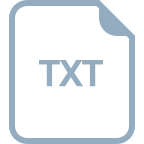
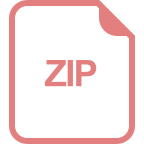
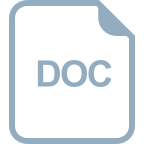
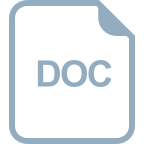
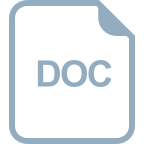
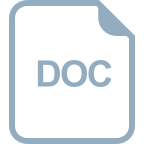
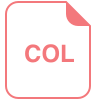
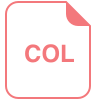