Optimizing Production Scheduling and Resource Allocation: Applications of MATLAB Linear Programming in Manufacturing
发布时间: 2024-09-15 09:50:12 阅读量: 23 订阅数: 26 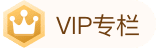
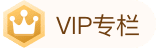
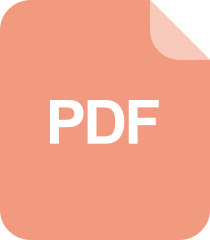
An Energy-Aware Algorithm for Optimizing Resource Allocation in Software Defined Network
# Optimizing Production Scheduling and Resource Allocation: The Application of MATLAB Linear Programming in Manufacturing
## 1. Theoretical Foundation of Linear Programming**
Linear programming is a mathematical optimization technique used to maximize or minimize a linear objective function subject to certain constraints. It is widely applied in manufacturing, finance, and logistics, among other fields.
The standard form of a linear programming problem is as follows:
```
max/min z = c^T x
subject to: Ax ≤ b, x ≥ 0
```
Where:
* z is the objective function, representing the value to be maximized or minimized
* c is the coefficient vector for the objective function
* x is the vector of decision variables
* A is the constraint matrix
* b is the constraint value vector
## 2. MATLAB Linear Programming Modeling
### 2.1 Mathematical Model of Linear Programming Problem
A linear programming problem (LP) is a mathematical optimization problem where both the objective function and constraints are linear. Its mathematical model is as follows:
**Objective Function:**
```
maximize z = c^T x
```
**Constraints:**
```
Ax ≤ b
x ≥ 0
```
Where:
***z** is the objective function value
***c** is the coefficient vector for the objective function
***x** is the vector of decision variables
***A** is the constraint matrix
***b** is the constraint vector
### 2.2 MATLAB Linear Programming Solvers
MATLAB offers various functions for solving linear programming problems, including:
***linprog()**: Used for solving standard linear programming problems
***intlinprog()**: Used for solving integer linear programming problems
***quadprog()**: Used for solving quadratic programming problems
The syntax and parameters for these functions are as follows:
```
[x, fval, exitflag, output] = linprog(f, A, b, Aeq, beq, lb, ub, x0, options)
```
***f**: Coefficient vector for the objective function
***A**: Constraint matrix
***b**: Constraint vector
***Aeq**: Equality constraint matrix
***beq**: Equality constraint vector
***lb**: Lower bound for decision variables
***ub**: Upper bound for decision variables
***x0**: Initial solution
***options**: Solver options
### 2.3 Steps for Model Establishment and Solution
The steps for establishing and solving a linear programming model using MATLAB are as follows:
1. **Establish the mathematical model:** Based on the actual problem, establish the mathematical model for the linear programming problem, including the objective function and constraints.
2. **Write MATLAB code:** Use MATLAB solver functions to write code, inputting model parameters and solver options.
3. **Solve the model:** Run the MATLAB code to solve the linear programming problem.
4. **Analyze the results:** Check the solution results, including the objective function value, decision variable values, and solution status.
**Code Example:**
```matlab
% Objective function coefficient vector
f = [3; 2];
% Constraint matrix
A = [1, 1; 2, 1];
% Constraint vector
b = [4; 6];
% Solve the linear programming problem
[x, fval, exitflag] = linprog(f, A, b);
% Output results
disp('Decision Variables:');
disp(x);
disp('Objective Function Value:');
disp(fval);
disp('Solution Status:');
disp(exitflag);
```
**Code Logic Analysis:**
* Lines 1-3 define the objective function coefficient vector, constraint matrix, and constraint
0
0
相关推荐
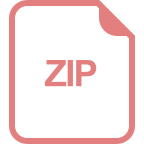
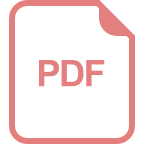
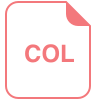
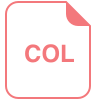
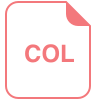
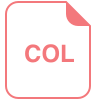
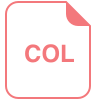
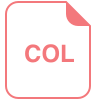