Unveiling MATLAB's Linear Programming Solver:揭秘MATLAB线性规划求解器 A Deep Dive into the Algorithm Principles and Implementation 大揭秘:算法原理与实现
发布时间: 2024-09-15 09:27:14 阅读量: 29 订阅数: 31 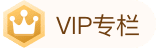
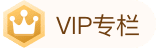
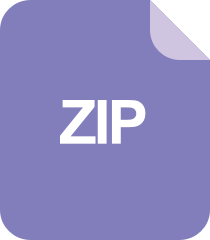
Unveiling-the-ActiLife-Algorithm--Converting-Raw-Acceleration-Data-to-Activity-Count:2015年无线健康大会论文
# Demystifying MATLAB's Linear Programming Solver: Algorithm Principles and Implementation Revealed
MATLAB is an advanced programming language widely used for scientific computation and data analysis. It provides a suite of powerful functions and toolboxes, including solvers for linear programming problems.
Linear programming is a mathematical optimization technique used to maximize or minimize a linear objective function under given constraints. MATLAB's linear programming solver is based on two primary algorithms: the Simplex method and the Interior Point method.
The Simplex method is an iterative algorithm that finds the optimal solution by moving through the feasible domain. It starts with an initial feasible solution and progressively improves it through iterative steps until the optimal solution is reached.
# Theoretical Foundations of Linear Programming
### 2.1 Establishing a Linear Programming Model
Linear programming (LP) is a mathematical optimization technique used to find the best values for a set of variables to maximize or minimize an objective function under given constraints. An LP model typically consists of the following components:
- **Objective Function:** The linear function to be maximized or minimized.
- **Decision Variables:** The unknowns to be determined.
- **Constraints:** The linear inequalities or equations imposed on the decision variables.
The standard form of an LP model is as follows:
```
Maximize/Minimize z = c^T x
Subject to:
Ax ≤ b
x ≥ 0
```
Where:
- `z` is the value of the objective function.
- `x` is the decision variable vector.
- `c` is the objective function coefficient vector.
- `A` is the constraint matrix.
- `b` is the constraint constant vector.
### 2.2 Standard Form and Dual Form of Linear Programming Problems
**Standard Form**
A standard form LP model satisfies the following conditions:
- All constraints are inequalities.
- All decision variables are non-negative.
**Dual Form**
The dual form LP model is derived from the standard form model by the following transformations:
- Convert the objective function from minimization to maximization.
- Reverse the inequality signs in the constraints.
- Replace the non-negativity constraints on decision variables with non-positivity constraints.
The standard form of the dual LP model is as follows:
```
Minimize w = b^T y
Subject to:
A^T y ≥ c
y ≥ 0
```
Where:
- `w` is the value of the dual objective function.
- `y` is the dual variable vector.
### 2.3 Feasible Domain and Optimal Solution of Linear Programming Problems
**Feasible Domain**
The feasible domain of an LP problem is the set of decision variable values that satisfy all constraints. It can be a convex set (where all points can be represented as a convex combination of any two other points) or a non-convex set.
**Optimal Solution**
The optimal solution of an LP problem is the value of the decision variables that maximizes or minimizes the objective function within the feasible domain. The optimal solution may be unique or there may be multiple.
**Code Block:**
```matlab
% Define the linear programming model
c = [3; 2]; % Objective function coefficients
A = [2 1; 1 2]; % Constraint matrix
b = [6; 4]; % Constraint constants
% Solve the linear programming problem
[x, fval, exitflag] = linprog(c, [], [], A, b, zeros(2, 1), []);
% Display results
disp('Decision variable values:');
disp(x);
disp('Objective function value:');
disp(fval);
```
**Logical Analysis:**
This code uses MATLAB's `linprog` function to solve a linear programming problem. The input parameters of the `linprog` function include:
- `c`: Objective function coefficient vector
- `A`: Constraint matrix
- `b`: Constraint constant vector
- `zeros(2, 1)`: Non-negativity constraint of the decision variables
The `linprog` function returns the following output parameters:
- `x`: Decision variable values
- `fval`: Objective function value
- `exitflag`: Solution status flag
**Parameter Description:**
- The default solving algorithm of the `linprog` function is the Simplex method, but other algorithms can be selected by setting option parameters.
- The `linprog` function also supports other types of constraints, such as equality constraints and range constraints.
- The `linprog` function can handle large sparse LP problems.
# 3.1 The Simplex Method
#### 3.1.1 Basic Principles of the Simplex Method
The Simplex method is an iterative algorithm for solving linear programming problems. Its basic principle is to start from a feasible solution to the problem and iteratively approach the optimal solution through a series of steps.
During each iteration, the Simplex method selects a non-basic variable (i.e., a variable not in the basis) to enter the basis and selects a basic variable to leave the basis. In this way, the Simplex method gradually improves the feasible solution until the optimal solution is found.
#### 3.1.2 Algorithm Steps of the Simplex Method
The steps of the Simplex method are as follows:
1. Convert the linear programming problem into standard form.
2. Find an initial feasible solution.
3. If the current feasible solution is not optimal, select a non-basic variable to enter the basis.
4. Select a basic variable to leave the basis.
5. Update the values of the basis and non-basic variables.
6. Repeat steps 3-5 until the optimal solution is found.
**Code Block:**
```matlab
% Define the linear programming problem
f = [-3, -4];
A = [2, 1; 1, 2];
b = [8; 6];
lb = [0; 0];
ub = [];
% Solve the linear
```
0
0
相关推荐







