揭秘计算几何基础:点、线、面的几何表示与运算(入门必读)
发布时间: 2024-08-26 03:26:06 阅读量: 10 订阅数: 22 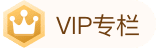
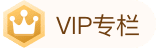
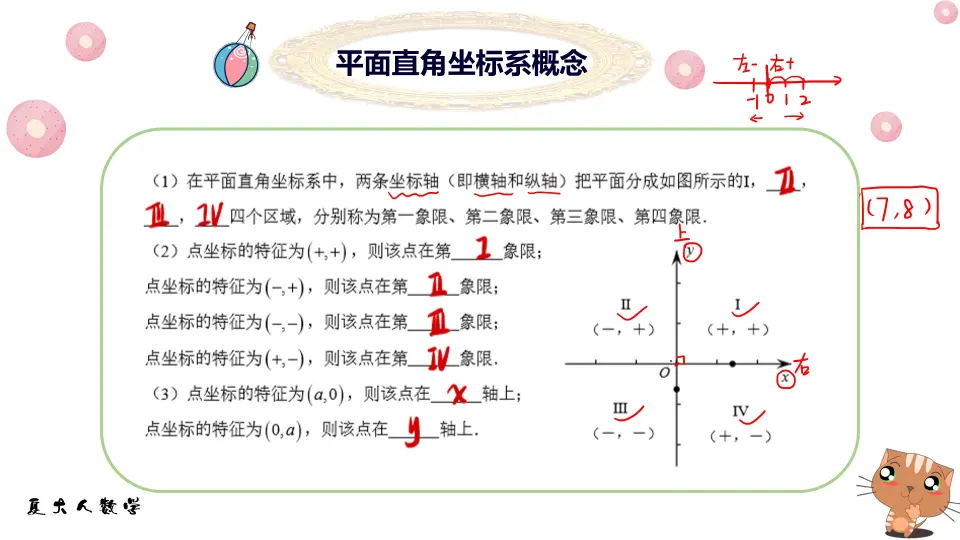
# 1. 计算几何简介
计算几何是计算机科学的一个分支,它研究几何问题的算法表示和解决方法。它将几何问题抽象为数据结构和算法,使计算机能够有效地处理和解决这些问题。
计算几何在计算机图形学、机器人、地理信息系统和分子建模等领域有着广泛的应用。它为这些领域提供了高效的几何计算方法,使计算机能够处理复杂的三维模型、规划机器人运动、分析地理数据和模拟分子结构。
# 2. 线、面在计算几何中的表示
在计算几何中,点、线、面是构成几何图形的基本元素。它们的表示和运算对于计算几何算法至关重要。
### 2.1 点的表示与运算
#### 2.1.1 点的坐标表示
点通常用其坐标来表示,坐标系的选择取决于具体问题。在二维空间中,点通常用笛卡尔坐标系中的 (x, y) 表示;在三维空间中,则用 (x, y, z) 表示。
#### 2.1.2 点的距离和夹角计算
**距离计算**
两个点之间的距离可以用欧几里得距离公式计算:
```python
def distance(p1, p2):
"""计算两个点之间的欧几里得距离。
参数:
p1 (tuple): 第一个点,(x, y)
p2 (tuple): 第二个点,(x, y)
返回:
float: 两个点之间的距离
"""
dx = p2[0] - p1[0]
dy = p2[1] - p1[1]
return (dx**2 + dy**2)**0.5
```
**夹角计算**
两个点之间的夹角可以用点积公式计算:
```python
def angle(p1, p2):
"""计算两个点之间的夹角。
参数:
p1 (tuple): 第一个点,(x, y)
p2 (tuple): 第二个点,(x, y)
返回:
float: 两个点之间的夹角(弧度)
"""
dot_product = p1[0] * p2[0] + p1[1] * p2[1]
magnitude1 = (p1[0]**2 + p1[1]**2)**0.5
magnitude2 = (p2[0]**2 + p2[1]**2)**0.5
return math.acos(dot_product / (magnitude1 * magnitude2))
```
### 2.2 线段的表示与运算
#### 2.2.1 线段的端点表示
线段由其两个端点表示,端点通常用坐标表示。
#### 2.2.2 线段的长度和方向计算
**长度计算**
线段的长度可以用两端点之间的距离计算:
```python
def length(segment):
"""计算线段的长度。
参数:
segment (tuple): 线段,((x1, y1), (x2, y2))
返回:
float: 线段的长度
"""
p1, p2 = segment
return distance(p1, p2)
```
**方向计算**
线段的方向可以用单位方向向量表示,单位方向向量可以通过两端点之间的差值计算:
```python
def direction(segment):
"""计算线段的单位方向向量。
参数:
segment (tuple): 线段,((x1, y1), (x2, y2))
返回:
tuple: 单位方向向量,(dx, dy)
"""
p1, p2 = segment
dx = p2[0] - p1[0]
dy = p2[1] - p1[1]
magnitude = (dx**2 + dy**2)**0.5
return (dx / magnitude, dy / magnitude)
```
### 2.3 多边形的表示与运算
#### 2.3.1 多边形的顶点表示
多边形由其顶点表示,顶点通常用坐标表示。
#### 2.3.2 多边形的周长和面积计算
**周长计算**
多边形的周长可以通过其所有边长的和计算:
```python
def perimeter(polygon):
"""计算多边形的周长。
参数:
polygon (list): 多边形,[(x1, y1), (x2, y2), ...]
返回:
float: 多边形的周长
"""
perimeter = 0
for i in range(len(polygon)):
p1 = polygon[i]
p2 = polygon[(i + 1) % len(polygon)]
perimeter += distance(p1, p2)
return perimeter
```
**面积计算**
多边形的面积可以通过三角剖分法计算:
```python
def area(polygon):
"""计算多边形的面积。
参数:
polygon (list): 多边形,[(x1, y1), (x2, y2), ...]
返回:
float: 多边形的面积
"""
area = 0
for i in range(len(polygon)):
p1 = polygon[i]
p2 = polygon[(i + 1) % len(polygon)]
area += (p1[0] * p2[1] - p2[0] * p1[1])
return abs(area) / 2
```
# 3.1 点集的凸包算法
#### 3.1.1 凸包的定义和性质
**凸包**是指包含给定点集的所有凸多边形中面积最小的凸多边形。凸多边形是指其内部任意两点的连线都在多边形内部的多边形。
凸包具有以下性质:
- 凸包的边数等于点集中的极值点(最左、最右、最上、最下)的个数。
- 凸包的每条边都是点集中两点的连线。
- 凸包的内部不包含任何点集中的点。
#### 3.1.2 Graham扫描算法
Graham扫描算法是一种计算点集凸包的经典算法。算法步骤如下:
1. **找到极左点:**在点集中找到横坐标最小的点,记为p0。
2. **按极角排序:**以p0为原点,按逆时针方向对其他点按极角排序,记为p1, p2, ..., pn。
3. **初始化凸包栈:**创建一个栈,并压入p0和p1。
4. **扫描点集:**从p2开始,逐个扫描点集中的点。
5. **判断凸性:**对于当前点pi,判断栈顶元素ptop和ptop-1是否与pi形成右转弯。如果是,则弹出栈顶元素。
6. **压入凸包栈:**将pi压入凸包栈。
7. **重复步骤5-6,直到扫描完所有点。**
```python
def graham_scan(points):
"""
Graham扫描算法计算点集凸包
参数:
points: 点集,每个点为一个元组(x, y)
返回:
凸包点集,按逆时针方向排序
"""
# 找到极左点
p0 = min(points, key=lambda p: p[0])
# 按极角排序
points.sort(key=lambda p: (p[1] - p0[1]) / (p[0] - p0[0]))
# 初始化凸包栈
stack = [p0, points[1]]
# 扫描点集
for i in range(2, len(points)):
# 判断凸性
while len(stack) >= 2:
ptop = stack[-1]
ptop_1 = stack[-2]
if cross_product(ptop, ptop_1, points[i]) > 0:
break
stack.pop()
# 压入凸包栈
stack.append(points[i])
return stack
```
**参数说明:**
* `points`:点集,每个点为一个元组`(x, y)`。
**代码逻辑:**
该代码实现了Graham扫描算法,通过按极角排序和判断凸性,逐个扫描点集中的点,将凸包的点压入栈中,最终返回按逆时针方向排序的凸包点集。
**逻辑分析:**
Graham扫描算法的时间复杂度为O(n log n),其中n是点集中的点个数。算法的正确性基于以下事实:凸包的边数等于极值点的个数,并且凸包的每条边都是点集中两点的连线。
# 4. 计算几何的应用
### 4.1 图形学中的计算几何
计算几何在图形学领域有着广泛的应用,特别是在三维建模和光线追踪中。
#### 4.1.1 三维建模中的多边形表示
在三维建模中,多边形是表示三维物体的基本单元。计算几何提供了高效的多边形表示方法,例如三角形网格和四边形网格。这些表示方法可以有效地存储和处理三维物体的几何信息,从而实现逼真的渲染和交互式操作。
#### 4.1.2 光线追踪中的射线相交检测
光线追踪是一种渲染技术,它通过模拟光线在场景中的传播来生成逼真的图像。计算几何中的射线相交检测算法在光线追踪中至关重要。这些算法可以快速确定射线是否与场景中的物体相交,从而计算出光线与物体表面的交点和法线。
### 4.2 机器学习中的计算几何
计算几何在机器学习中也扮演着重要的角色,特别是在聚类算法和降维算法中。
#### 4.2.1 聚类算法中的点集分割
聚类算法旨在将数据点划分为不同的组或簇。计算几何中的点集分割算法可以帮助确定数据点之间的相似性和距离,从而生成高质量的聚类结果。
#### 4.2.2 降维算法中的主成分分析
主成分分析 (PCA) 是一种降维算法,它可以将高维数据投影到低维空间中。计算几何中的凸包算法可以帮助识别数据点中的主成分,从而实现有效的降维。
### 4.3 计算机视觉中的计算几何
计算几何在计算机视觉中也有着广泛的应用,例如图像分割和目标检测。
#### 4.3.1 图像分割中的凸包算法
图像分割旨在将图像划分为不同的区域或对象。计算几何中的凸包算法可以帮助识别图像中连通区域的凸包,从而实现有效的图像分割。
#### 4.3.2 目标检测中的线段相交检测
目标检测算法旨在识别图像中的特定对象。计算几何中的线段相交检测算法可以帮助确定目标边界与图像中其他元素之间的相交关系,从而提高目标检测的准确性。
# 5. 计算几何的扩展
### 5.1 计算几何中的空间数据结构
空间数据结构是用于组织和管理空间数据的结构。它们在计算几何中至关重要,因为它们可以有效地存储和查询空间对象,例如点、线段和多边形。
#### 5.1.1 四叉树
四叉树是一种树形数据结构,用于对二维空间进行划分。它将空间递归地划分为四个相等的子区域,直到达到预定义的深度或满足某些条件。
**代码块:**
```python
class QuadTree:
def __init__(self, boundary, depth=0):
self.boundary = boundary
self.depth = depth
self.children = [None] * 4
def insert(self, point):
if not self.boundary.contains(point):
return
if self.depth == 0:
self.children = [None] * 4
self.children[0] = QuadTree(self.boundary.nw_child(), self.depth + 1)
self.children[1] = QuadTree(self.boundary.ne_child(), self.depth + 1)
self.children[2] = QuadTree(self.boundary.sw_child(), self.depth + 1)
self.children[3] = QuadTree(self.boundary.se_child(), self.depth + 1)
child_index = self.boundary.get_child_index(point)
self.children[child_index].insert(point)
def query(self, query_boundary):
if not self.boundary.intersects(query_boundary):
return []
if self.depth == 0:
return [point for point in self.points if query_boundary.contains(point)]
results = []
for child in self.children:
results.extend(child.query(query_boundary))
return results
```
**逻辑分析:**
* `__init__`方法初始化四叉树,指定边界和深度。
* `insert`方法递归地将点插入四叉树。如果点不在边界内,则返回。如果深度为0,则创建四个子四叉树。否则,将点插入相应的子四叉树。
* `query`方法递归地查询四叉树中的点。如果查询边界与四叉树边界不相交,则返回空列表。如果深度为0,则返回边界内所有点。否则,查询所有子四叉树并返回结果。
#### 5.1.2 K-D树
K-D树是一种树形数据结构,用于对多维空间进行划分。它将空间递归地划分为两个子区域,每个子区域沿一个维度划分。
**代码块:**
```python
class KDTree:
def __init__(self, points, depth=0):
self.points = points
self.depth = depth
self.axis = depth % len(points[0])
self.left = None
self.right = None
def build(self):
if len(self.points) <= 1:
return
self.points.sort(key=lambda point: point[self.axis])
median_index = len(self.points) // 2
median_point = self.points[median_index]
self.left = KDTree(self.points[:median_index], self.depth + 1)
self.right = KDTree(self.points[median_index + 1:], self.depth + 1)
def query(self, query_point, radius):
if self.points is None:
return []
results = []
if self.distance(query_point, self.points[0]) <= radius:
results.append(self.points[0])
if self.axis == 0:
if query_point[0] <= self.points[0][0]:
results.extend(self.left.query(query_point, radius))
else:
results.extend(self.right.query(query_point, radius))
else:
if query_point[1] <= self.points[0][1]:
results.extend(self.left.query(query_point, radius))
else:
results.extend(self.right.query(query_point, radius))
return results
```
**逻辑分析:**
* `__init__`方法初始化K-D树,指定点和深度。
* `build`方法递归地构建K-D树。它对点进行排序,选择中值点,并创建两个子树。
* `query`方法递归地查询K-D树中的点。它检查查询点是否在子树的范围内,并返回距离查询点半径范围内的点。
### 5.2 计算几何中的算法优化
算法优化技术可以提高计算几何算法的效率。这些技术包括:
#### 5.2.1 并行算法
并行算法利用多核处理器或分布式系统来同时执行多个任务。这可以显著提高算法的性能,尤其是对于大型数据集。
#### 5.2.2 近似算法
近似算法提供近似解决方案,而不是精确解决方案。这可以减少算法的计算复杂度,同时仍然提供合理的答案。
# 6. 计算几何的前沿研究
计算几何作为一门不断发展的学科,近年来在多个领域取得了突破性的进展。以下是一些计算几何前沿研究方向:
### 6.1 计算几何中的拓扑学
拓扑学是数学的一个分支,它研究几何对象的形状和连接性,而不考虑它们的度量。计算几何中的拓扑学研究将拓扑概念应用于计算几何问题。
一个重要的研究领域是**拓扑数据分析**,它使用拓扑技术来分析数据并识别其潜在结构。这在医疗成像、材料科学和金融等领域具有广泛的应用。
### 6.2 计算几何中的代数方法
代数方法在计算几何中发挥着越来越重要的作用。**代数几何**将代数和几何结合起来,为解决计算几何问题提供了新的工具。
例如,**多项式优化**使用多项式方程来表示几何对象,并通过求解这些方程来优化几何问题。这在机器人运动规划和计算机图形学中有着重要的应用。
### 6.3 计算几何在其他领域的应用
计算几何的原则和技术在其他领域也得到了广泛的应用,例如:
* **生物信息学:**计算几何用于分析蛋白质结构、DNA序列和基因组数据。
* **材料科学:**计算几何用于设计和模拟新材料的结构和性能。
* **金融:**计算几何用于分析金融数据并识别市场模式。
这些前沿研究方向表明,计算几何是一个充满活力的领域,它不断为解决各种实际问题提供新的方法和见解。
0
0
相关推荐
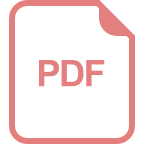
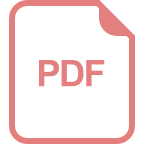
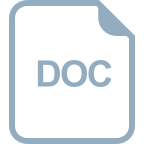





