Unveiling the Doris Database Architecture: A Comprehensive Analysis from Storage to Querying
发布时间: 2024-09-14 22:25:20 阅读量: 45 订阅数: 35 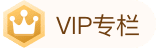
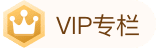
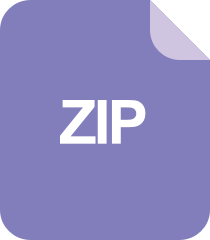
(179979052)基于MATLAB车牌识别系统【带界面GUI】.zip
# 1. Doris Database Overview
Doris is an open-source distributed MPP (Massively Parallel Processing) database designed for large-scale data analytics. It utilizes columnar storage and MPP architecture to efficiently process petabyte-level data and offers sub-second query response times.
Key features of Doris include:
- **High Performance:** Columnar storage and MPP architecture enable Doris to quickly process large-scale data queries.
- **High Availability:** Doris employs a replica mechanism and failover mechanism to ensure data high availability and reliability.
- **Scalability:** Doris can easily scale up to hundreds of nodes to meet growing data demands.
- **Ease of Use:** Doris supports standard SQL syntax and provides a rich set of APIs and tools for developers.
# 2. Doris Storage Architecture
### 2.1 Columnar Storage Principles
#### 2.1.1 Data Layout and Compression
Doris adopts a columnar storage architecture that stores data on disk by column. This approach has several advantages compared to traditional row-based storage:
***High Data Compression Rate:** Similar data types and values within the same column lead to more efficient compression.
***Faster Query Speed:** Only relevant columns are read during queries, reducing IO overhead.
***Good Scalability:** Columns can be added or removed easily without affecting other columns' data.
Doris uses various compression algorithms, including Snappy, Zlib, and LZ4, to further enhance data compression rates.
#### 2.1.2 Data Partitioning and Replicas
To enhance query performance and data reliability, Doris partitions data into multiple segments. Each partition contains records within a specific time or data range.
Doris also supports data replication to ensure data redundancy and high availability. Replicas can be stored on different machines, so if one machine fails, other replicas can provide data service.
### 2.2 Storage Engine Implementation
#### 2.2.1 Storage Formats and Indexes
Doris uses Parquet file format for data storage. Parquet is a columnar storage format that supports various compression algorithms and encoding schemes.
Doris supports multiple index types, including Bloom filters, bitmap indexes, and skip list indexes. These indexes can accelerate query performance, especially for filtering and aggregation operations.
#### 2.2.2 Data Loading and Updates
Doris supports various data loading methods, including:
***Streaming Loading:** Real-time loading of data through Kafka or other streaming data sources.
***Batch Loading:** Loading large amounts of data through files or HDFS.
***Incremental Loading:** Only loading the data that has been updated since the last load.
Doris also supports data update operations, including insertion, update, and deletion. Update operations are implemented by writing to a WAL (Write-Ahead Log) to ensure data consistency and reliability.
**Code Block:**
```python
import doris
# Create a Doris client
client = doris.Client("***.*.*.*", 8030)
# Create a table
client.create_table("test_table", {
"id": "INT",
"name": "STRING",
"age": "INT"
})
# Load data
client.load_data("test_table", "hdfs://path/to/data.parquet")
# Query data
result = client.query("SELECT * FROM test_table")
# Print results
for row in result:
print(row)
```
**Logical Analysis:**
This code demonstrates how to use the Doris client to create tables, load data, and query data.
* The `create_table` function is used to create a table and specify the column names and data types.
* The `load_data` function is used to load data from HDFS into the table.
* The `query` function is used to query data in the table.
* The `result` variable is a generator that iterates over the query results.
* The `for` loop is used to print each row in the query results.
**Parameter Explanation:**
* `client`: Doris client object.
* `table_name`: Name of the table to create or query.
* `schema`: Columns and data types of the table.
* `data_path`: Path of the data to be loaded.
* `sql`: SQL query to execute.
# 3. Doris Query Engine
### 3.1 Query Optimizer
The query optimizer is the core component of the Doris query engine, responsible for converting user queries into efficient execution plans.
#### 3.1.1 Query Plan Generation
The query optimizer first performs syntactic and semantic analysis on the user query, generating a query tree. Then, it applies a series of optimization rules to optimize the query tree, such as:
- **Predicate Pushdown:** Pushing predicate conditions down to subqueries or join operations to reduce the amount of data that needs to be processed.
- **Join Reordering:** Reordering join operations to optimize the execution plan, e.g., using hash join or nested loop join.
- **Subquery Unnesting:** Unnesting subqueries into inline views to eliminate unnecessary nested queries.
#### 3.1.2 Cost Estimation
After generating query plans, the query optimizer performs cost estimation for each plan to choose the optimal execution plan. Cost estimation is based on statistical information, such as table size, column cardinality, and query predicate selectivity.
### 3.2 Execution Engine
The execution engine is responsible for executing query plans. It uses vectorized and parallel execution techniques to improve query performance.
#### 3.2.1 Vectorized Execution
Vectorized execution organizes data in the query into vectors rather than processing data row by row. This significantly reduces memory access and CPU overhead, thereby increasing query speed.
For example, the following code demonstrates an example of vectorized execution:
```python
import numpy as np
# Create a DataFrame with 10 million rows of data
df = pd.DataFrame({'col1': np.random.randint(1000, size=***), 'col2': np.random.rand(***)})
# Query using vectorized execution
result = df.query('col1 > 500 and col2 < 0.5')
```
#### 3.2.2 Parallel Execution
Parallel execution breaks down query tasks into multiple subtasks and executes these subtasks in parallel on multiple computing nodes. This significantly reduces query time, especially when dealing with large datasets.
For example, the following mermaid flowchart illustrates an example of parallel execution:
```mermaid
sequenceDiagram
participant User
participant Query Optimizer
participant Execution Engine
User->Query Optimizer: Send query
Query Optimizer->Execution Engine: Generate execution plan
Execution Engine->User: Return execution plan
Execution Engine->Node 1: Execute subtask 1
Execution Engine->Node 2: Execute subtask 2
Node 1->Execution Engine: Return subtask 1 result
Node 2->Execution Engine: Return subtask 2 result
Execution Engine->User: Return query result
```
# 4. Doris Application Scenarios
Doris database showcases its powerful performance and flexible architecture in a variety of application scenarios. This chapter will delve into Doris's applications in real-time analytics and offline analytics domains and provide specific examples and best practices.
## 4.1 Real-Time Analytics
Real-time analytics refers to the processing and analysis of continuously changing data in real-time to obtain the latest insights. Doris has several advantages in real-time analytics:
- **Low Latency Data Ingestion:** Doris supports various data ingestion methods, including Kafka, Flume, and HTTP API, allowing for fast and efficient ingestion of streaming data.
- **Real-Time Computing:** Doris's query engine supports stream processing, enabling real-time computation and aggregation of incoming data to generate real-time dashboards and alerts.
### 4.1.1 Stream Processing
Doris can serve as a stream processing platform for real-time analysis of streaming data from various sources. Its streaming processing capabilities include:
- **Window Functions:** Doris supports a variety of window functions, such as sliding windows, hopping windows, and session windows, enabling grouping and aggregation of streaming data.
- **Time Series Analysis:** Doris provides a rich set of time series analysis functions for trend analysis, anomaly detection, and forecasting of time series data.
```sql
CREATE TABLE stream_data (
user_id INT,
event_time TIMESTAMP,
event_type STRING,
event_value DOUBLE
) ENGINE=OLAP
DISTRIBUTED BY HASH(user_id) BUCKETS 10;
INSERT INTO stream_data (user_id, event_time, event_type, event_value)
VALUES (1, '2023-03-08 10:00:00', 'purchase', 100.00),
(2, '2023-03-08 10:05:00', 'view', 10.00),
(3, '2023-03-08 10:10:00', 'purchase', 200.00);
SELECT user_id,
SUM(event_value) AS total_value
FROM stream_data
WHERE event_time >= '2023-03-08 10:00:00'
GROUP BY user_id
WINDOW AS (PARTITION BY user_id ORDER BY event_time ROWS BETWEEN 1 PRECEDING AND CURRENT ROW);
```
### 4.1.2 Real-Time Dashboards
Doris can act as the underlying data source for real-time dashboards, providing real-time visual data insights to users. Its real-time dashboard features include:
- **Dashboard Building:** Doris supports building real-time dashboards through SQL statements or third-party tools, displaying various metrics and charts.
- **Data Refreshing:** Doris's real-time dashboards can automatically refresh data, ensuring users always see the latest information.
## 4.2 Offline Analytics
Offline analytics refers to the batch processing and analysis of historical data to obtain long-term trends and patterns. Doris has several advantages in offline analytics:
- **Big Data Processing:** Doris can process vast amounts of data, supporting PB-level storage and analytics.
- **Flexible Data Models:** Doris supports flexible data models, adapting easily to ever-changing business needs.
### 4.2.1 Big Data Processing
Doris can serve as a big data processing platform, analyzing big data from various sources. Its big data processing features include:
- **Data Importing:** Doris supports various data importing methods, including Hive, HDFS, and CSV files, enabling efficient importation of large-scale data.
- **Data Processing:** Doris provides a rich set of SQL functions and UDFs for various data processing operations, such as filtering, aggregation, and transformation.
```sql
CREATE TABLE sales_data (
order_id INT,
product_id INT,
quantity INT,
sales_amount DOUBLE
) ENGINE=OLAP
DISTRIBUTED BY HASH(order_id) BUCKETS 10;
INSERT INTO sales_data (order_id, product_id, quantity, sales_amount)
SELECT order_id, product_id, SUM(quantity), SUM(sales_amount)
FROM raw_sales_data
GROUP BY order_id, product_id;
SELECT product_id,
SUM(sales_amount) AS total_sales
FROM sales_data
GROUP BY product_id;
```
### 4.2.2 Data Warehouse
Doris can act as a data warehouse, providing a unified data view for enterprises and supporting multidimensional analysis and decision-making. Its data warehouse features include:
- **Data Integration:** Doris can integrate data from various sources, including relational databases, NoSQL databases, and file systems.
- **Data Modeling:** Doris supports flexible data modeling, capable of building star schemas, snowflake schemas, and dimension models.
# 5. Doris Best Practices
### 5.1 Performance Tuning
#### 5.1.1 Hardware Configuration Optimization
***CPU:** Choose CPUs with high frequencies and sufficient core counts to meet query processing needs.
***Memory:** Allocate adequate memory to cache query data and intermediate results, reducing disk IO.
***Storage:** Use SSD or NVMe storage devices to improve data read speeds.
***Network:** Ensure network bandwidth and latency meet the requirements for parallel query execution.
#### 5.1.2 SQL Statement Optimization
***Use Columnar Storage Formats:** Doris utilizes columnar storage, optimizing query performance for specific columns.
***Avoid Full Table Scans:** Use WHERE clauses and indexes to filter data, reducing the amount of data scanned.
***Use Vectorized Execution:** Doris supports vectorized execution, processing multiple data rows at once, increasing query speed.
***Optimize JOIN Operations:** Use appropriate JOIN algorithms (e.g., Nested Loop Join, Hash Join) and consider data distribution.
***Use Materialized Views:** Pre-calculate frequently queried data and store it in materialized views to increase query speed.
### 5.2 Operations Management
#### 5.2.1 Cluster Deployment and Monitoring
***Cluster Deployment:** Choose an appropriate cluster size and configuration based on business needs and data volume.
***Monitoring:** Use monitoring tools (e.g., Prometheus, Grafana) to monitor the health of the cluster, including CPU, memory, storage, and network usage.
#### 5.2.2 Fault Handling and Recovery
***Fault Handling:** Establish fault handling mechanisms, including automatic failover, data backup, and recovery.
***Data Backup:** Regularly back up data to prevent data loss and consider off-site backups to enhance disaster recovery capabilities.
***Data Recovery:** Use backup data to recover the cluster in the event of a failure and minimize data loss.
0
0