this.render = function () { this.renderer.render(this.scene, this.camera); }; this.add = function (elem) { this.scene.add(elem); }; this.remove = function (elem) { this.scene.remove(elem); }; this.container = document.getElementById('game'); // renderer this.renderer = new THREE.WebGLRenderer({ antialias: true, alpha: false }); this.renderer.setSize(window.innerWidth, window.innerHeight); this.renderer.setClearColor('#D0CBC7', 1); this.container.appendChild(this.renderer.domElement);
时间: 2024-02-14 18:20:43 浏览: 25
这段代码看起来像是关于Three.js的渲染器和场景的初始化和设置。首先,它创建了一个`WebGLRenderer`对象,并设置了抗锯齿和背景颜色。然后,它将渲染器的输出添加到HTML页面的一个元素上。接着,它定义了一个场景对象,并添加了一个`add`函数,用于向场景添加元素。最后,它定义了一个`render`函数,用于渲染场景。
相关问题
gui.add(mesh.position, 'x', 0, 100).onChange(function(value) { mesh.position.x = value; console.log(camera.parent); console.log(mesh.position.x==value); //TODO:数据绑定成功,需要把结果渲染至页面上 renderer.render(camera,scene);
这段代码看起来是在使用 dat.gui 库来创建一个滑块控制网格对象的 X 轴位置。根据你提供的代码,错误 "camera is not an instance of THREE.Camera" 可能出现在 `renderer.render(camera, scene)` 这一行。
要解决这个问题,你可以尝试以下几个步骤:
1. 确保你已经正确地创建了 `camera` 和 `renderer` 对象,并将它们与正确的场景 (`scene`) 关联起来。检查代码中是否有其他地方对 `camera` 进行了错误的赋值。
2. 确保 `camera` 是 `THREE.Camera` 的一个实例。你可以在创建相机对象时使用正确的构造函数,例如 `THREE.PerspectiveCamera` 或 `THREE.OrthographicCamera`。
3. 检查 `renderer` 对象是否已正确初始化,并且已经设置了正确的大小和其他参数。确保将 `renderer` 对象与正确的 HTML 元素关联起来,并在渲染之前将其添加到 DOM 中。
4. 确保 `scene` 包含了你想要渲染的网格对象 (`mesh`)。检查代码中是否正确添加了 `mesh` 对象到 `scene` 中。
如果以上步骤仍然无法解决问题,请提供更多关于你的代码和错误上下文的信息,以便更进一步帮助你解决问题。
import * as THREE from 'three'; const scene = new THREE.Scene(); const geometry = new THREE.BoxGeometry(100, 100, 100); const material = new THREE.MeshPhongMaterial({ color: 0xff0000, shininess: 20, specular: 0x444444, side: 0 }); const mesh = new THREE.Mesh(geometry, material); mesh.position.set(0, 0, 0); scene.add(mesh); const width = 800; //宽度 const height = 500; //高度 const camera = new THREE.PerspectiveCamera(30, width / height, 1, 3000); camera.position.set(200, 200, 200); camera.lookAt(mesh.position); //镜头中心对准对象的原点 const renderer = new THREE.WebGLRenderer(); renderer.setSize(width, height); const gui = new GUI(); gui.add(mesh.position, 'x', 0, 100).onChange(function(value) { mesh.position.x = value; console.log(camera.parent); console.log(mesh.position.x==value); renderer.render(camera,scene);
根据你提供的代码,我注意到在创建材质对象 `material` 的代码行末尾有一个额外的分号 `;`。这个分号可能导致语法错误,从而导致后续的代码无法正确执行。
请尝试删除这个额外的分号,将代码修改为:
```javascript
const material = new THREE.MeshPhongMaterial({
color: 0xff0000,
shininess: 20,
specular: 0x444444,
side: 0
});
```
然后重新运行你的代码,看看是否仍然出现 "camera is not an instance of THREE.Camera" 错误。如果错误仍然存在,请提供完整的错误消息和堆栈跟踪,以便更进一步帮助你解决问题。
相关推荐
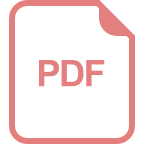
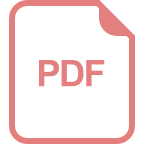












