texture = cv2.remap(image, pos_interpolated[:, :, :2].astype(np.float32), None, interpolation=cv2.INTER_LINEAR, borderMode=cv2.BORDER_CONSTANT, borderValue=0)是什么意思
时间: 2024-02-29 16:54:32 浏览: 113
这段代码使用了 OpenCV 库中的 remap 函数,对输入的图像 image 进行了重映射操作。其中,pos_interpolated 是一个二维数组,存储了输入图像中的每个像素点在输出图像中对应的位置。这个数组的大小应该和输入图像的大小一致,每个元素包含两个浮点数表示输出图像中对应像素的位置。函数的第二个参数就是这个数组,它被转换为 float32 类型并传递给了 remap 函数。
函数的第三个参数为 None,表示不使用 mask。interpolation 参数指定了插值方法,这里使用了线性插值(INTER_LINEAR)。borderMode 参数指定了边界填充方式,这里使用了常数填充(BORDER_CONSTANT)。borderValue 参数指定了常数填充时的值,这里填充为 0。
最终,函数返回经过重映射后的图像 texture。
相关问题
这段代码什么意思def run_posmap_300W_LP(bfm, image_path, mat_path, save_folder, uv_h = 256, uv_w = 256, image_h = 256, image_w = 256): # 1. load image and fitted parameters image_name = image_path.strip().split('/')[-1] image = io.imread(image_path)/255. [h, w, c] = image.shape info = sio.loadmat(mat_path) pose_para = info['Pose_Para'].T.astype(np.float32) shape_para = info['Shape_Para'].astype(np.float32) exp_para = info['Exp_Para'].astype(np.float32) # 2. generate mesh # generate shape vertices = bfm.generate_vertices(shape_para, exp_para) # transform mesh s = pose_para[-1, 0] angles = pose_para[:3, 0] t = pose_para[3:6, 0] transformed_vertices = bfm.transform_3ddfa(vertices, s, angles, t) projected_vertices = transformed_vertices.copy() # using stantard camera & orth projection as in 3DDFA image_vertices = projected_vertices.copy() image_vertices[:,1] = h - image_vertices[:,1] - 1 # 3. crop image with key points kpt = image_vertices[bfm.kpt_ind, :].astype(np.int32) left = np.min(kpt[:, 0]) right = np.max(kpt[:, 0]) top = np.min(kpt[:, 1]) bottom = np.max(kpt[:, 1]) center = np.array([right - (right - left) / 2.0, bottom - (bottom - top) / 2.0]) old_size = (right - left + bottom - top)/2 size = int(old_size*1.5) # random pertube. you can change the numbers marg = old_size*0.1 t_x = np.random.rand()*marg*2 - marg t_y = np.random.rand()*marg*2 - marg center[0] = center[0]+t_x; center[1] = center[1]+t_y size = size*(np.random.rand()*0.2 + 0.9) # crop and record the transform parameters src_pts = np.array([[center[0]-size/2, center[1]-size/2], [center[0] - size/2, center[1]+size/2], [center[0]+size/2, center[1]-size/2]]) DST_PTS = np.array([[0, 0], [0, image_h - 1], [image_w - 1, 0]]) tform = skimage.transform.estimate_transform('similarity', src_pts, DST_PTS) cropped_image = skimage.transform.warp(image, tform.inverse, output_shape=(image_h, image_w)) # transform face position(image vertices) along with 2d facial image position = image_vertices.copy() position[:, 2] = 1 position = np.dot(position, tform.params.T) position[:, 2] = image_vertices[:, 2]*tform.params[0, 0] # scale z position[:, 2] = position[:, 2] - np.min(position[:, 2]) # translate z # 4. uv position map: render position in uv space uv_position_map = mesh.render.render_colors(uv_coords, bfm.full_triangles, position, uv_h, uv_w, c = 3) # 5. save files io.imsave('{}/{}'.format(save_folder, image_name), np.squeeze(cropped_image)) np.save('{}/{}'.format(save_folder, image_name.replace('jpg', 'npy')), uv_position_map) io.imsave('{}/{}'.format(save_folder, image_name.replace('.jpg', '_posmap.jpg')), (uv_position_map)/max(image_h, image_w)) # only for show # --verify # import cv2 # uv_texture_map_rec = cv2.remap(cropped_image, uv_position_map[:,:,:2].astype(np.float32), None, interpolation=cv2.INTER_LINEAR, borderMode=cv2.BORDER_CONSTANT,borderValue=(0)) # io.imsave('{}/{}'.format(save_folder, image_name.replace('.jpg', '_tex.jpg')), np.squeeze(uv_texture_map_rec))
这段代码是一个函数,用于将一张人脸图像进行三维重建并生成该人脸在二维图像上的 UV 位置图。具体步骤如下:
1. 加载人脸图像和拟合参数。
2. 生成人脸三维模型,并进行变换,得到变换后的人脸模型顶点位置。
3. 根据人脸关键点进行裁剪,得到人脸区域的图像,并记录变换参数。
4. 在 UV 空间中渲染人脸模型,并生成 UV 位置图。
5. 保存裁剪后的图像和 UV 位置图。
其中,变换参数包括旋转、平移、缩放等。UV 位置图是将人脸模型在 UV 空间中的位置映射到二维图像上的一张纹理图。
## extract texture ## TODO: current resolution 256x256, support higher resolution, and add visibility uv_pverts = self.render.world2uv(trans_verts) uv_gt = F.grid_sample(images, uv_pverts.permute(0,2,3,1)[:,:,:,:2], mode='bilinear', align_corners=False) if self.cfg.model.use_tex: ## TODO: poisson blending should give better-looking results if self.cfg.model.extract_tex: uv_texture_gt = uv_gt[:,:3,:,:]*self.uv_face_eye_mask + (uv_texture[:,:3,:,:]*(1-self.uv_face_eye_mask)) else: uv_texture_gt = uv_texture[:,:3,:,:] else: uv_texture_gt = uv_gt[:,:3,:,:]*self.uv_face_eye_mask + (torch.ones_like(uv_gt[:,:3,:,:])*(1-self.uv_face_eye_mask)*0.7) 怎么更改分辨率
在这段代码中,提取纹理的过程中使用了`F.grid_sample`函数进行双线性插值,该函数的第二个参数是采样的位置,可以通过调整这个参数来更改纹理分辨率。具体来说,你可以按照以下步骤进行调整:
1. 找到`F.grid_sample`函数的第二个参数,即`uv_pverts.permute(0,2,3,1)[:,:,:,:2]`。
2. 根据需要更改采样的位置,使其适应你想要的纹理分辨率。具体来说,你可以将采样的位置缩小或者放大,来调整纹理分辨率。例如,如果你想将纹理分辨率提高到512x512,可以将该参数改为`uv_pverts.permute(0,2,3,1)[:,:,:,:2]*2`。
3. 保存更改后,重新运行程序即可。
希望这个回答能够对你有所帮助。如果你有任何其他问题,请随时问我。
阅读全文
相关推荐
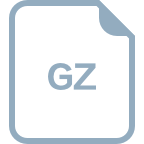
















