下列给定程序中,函数fun的功能是:用下面的公式求pi的近似值,直到最后一项的绝对值小于指定的数(参数 num)为止。 pi/4≈1-1/3+1/5-1/7+... 例如程序运行后,若输入0.0001,则程序输出3.1414 请修改程序中的错误,使它能得出正确的结果。 注意: 请将修改正确后的完整源程序拷贝粘贴到答题区内。 不得增行或删行,也不得更改程序的结构。 对于没有错误的语句,请不要修改,修改原本正确的语句也要扣分。 当且仅当错误全部改正,且程序运行结果调试正确,才给加5分。 经教师手工核对后,如果未用指针做函数参数编程,那么即使做对也不给分。 #include <stdio.h> #include <math.h> int fun(float num) { int s; float n,t,pi; t=0; pi=0; n=0; s=-1; while(t>=num); { pi=pi+t; n=n+1; s=+s; t=s%n; } pi=pi*4; return pi; } main() { float n1,n2; printf("Enter a float number: "); scanf(" %d",&n1); n2=fun(n1); printf( "%6.4f\n ",n2); }
时间: 2024-03-23 10:43:28 浏览: 48
程序中有以下错误:
1.在scanf中,输入参数为%f,而不是%d;
2.在while循环中,分号位置不正确,应该放在while条件的后面;
3.在计算t的值时,应该使用1/n而不是s/n。
修改后的程序如下:
```c
#include <stdio.h>
#include <math.h>
int fun(float num) {
int s;
float n,t,pi;
t=0;
pi=0;
n=0;
s=-1;
while(t>=num)
{
pi=pi+t;
n=n+1;
s=-s;
t=s/(2*n-1);
}
pi=pi*4;
return pi;
}
int main() {
float n1,n2;
printf("Enter a float number: ");
scanf("%f",&n1);
n2=fun(n1);
printf("%6.4f\n",n2);
return 0;
}
```
相关问题
本题要求编写程序,用序列求和公式 求自然对数底e的近似值,直到最后一项的绝对值小于给定精度eps。
可以使用以下序列求和公式来计算自然对数底e的近似值:
e ≈ 1 + 1/1! + 1/2! + 1/3! + ... + 1/n!
其中n是一个很大的正整数,eps是给定的精度。我们可以通过不断增加n的值来逼近e的真实值,直到最后一项的绝对值小于eps为止。
具体实现时,可以使用一个循环来计算序列的每一项,并将它们累加起来。在每次循环中,需要计算当前项的值和绝对值,并判断绝对值是否小于eps。如果是,则退出循环并返回当前的近似值。
以下是一个示例代码:
```
#include <stdio.h>
#include <math.h>
double approx_e(double eps) {
double e = 1.;
double term = 1.;
int n = 1;
do {
term /= n;
e += term;
n++;
term *= n;
} while (fabs(term) > eps);
return e;
}
int main() {
double eps = 1e-6;
double e = approx_e(eps);
printf("e = %lf\n", e);
return ;
}
```
在这个示例中,我们使用了一个do-while循环来计算序列的每一项。变量e表示当前的近似值,变量term表示当前项的值,变量n表示当前项的下标。在每次循环中,我们首先计算当前项的值,然后将它累加到e中。接着,我们更新n和term的值,以便计算下一项。最后,我们判断最后一项的绝对值是否小于eps,如果是,则退出循环并返回当前的近似值。
在主函数中,我们定义了eps的值为1e-6,然后调用approx_e函数来计算e的近似值。最后,我们输出e的值并结束程序。
python格里高利公式计算π的近似值,直到最后一项的绝对值小于给定精度eps。
可以使用以下代码实现:
```python
import math
def gregory_leibniz(eps):
pi_estimate = 0
iterations = 0
while True:
numerator = 4 * (-1) ** iterations
denominator = 2 * iterations + 1
term = numerator / denominator
pi_estimate += term
iterations += 1
if abs(term) < eps:
break
return pi_estimate
print(gregory_leibniz(0.0001))
```
这个程序使用格里高利-莱布尼茨级数计算π的近似值,直到最后一项的绝对值小于给定精度eps。
相关推荐
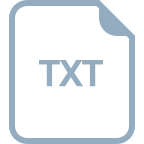
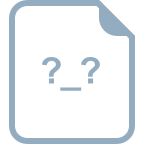
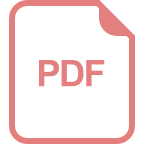
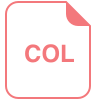

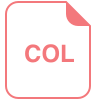










