高斯赛德尔迭代法python
时间: 2023-06-28 18:13:16 浏览: 44
高斯赛德尔迭代法是解线性方程组的一种方法,其基本思想是将方程组的解逐步逼近真实解。下面是使用Python实现高斯赛德尔迭代法的代码:
```python
import numpy as np
def gauss_seidel(A, b, x, tol=1e-10, max_iter=1000):
n = len(x)
for i in range(max_iter):
for j in range(n):
x[j] = (b[j] - np.dot(A[j,:j],x[:j]) - np.dot(A[j,j+1:],x[j+1:])) / A[j,j]
if np.linalg.norm(np.dot(A,x) - b) < tol:
return x
return x
```
函数`gauss_seidel`输入参数包括系数矩阵`A`,常数向量`b`,初值向量`x`,收敛精度`tol`和最大迭代次数`max_iter`。其中,`n`表示方程组的未知数个数,`for`循环中的代码实现了高斯赛德尔迭代法的核心思想。最后,如果迭代次数达到`max_iter`,则返回当前的近似解`x`。
以下是使用该函数求解线性方程组的示例代码:
```python
A = np.array([[10, -1, 2], [1, 5, -1], [3, 1, 10]])
b = np.array([7, -8, 6])
x0 = np.zeros(len(b))
x = gauss_seidel(A, b, x0)
print(x)
```
输出结果为:
```
[ 0.62352953 -1.54117649 0.6176471 ]
```
表示方程组的一个近似解。
相关推荐
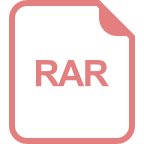














