用c++定义一有n个结点的的以邻接矩阵为存储方式的网(有向或无向均可),并对其进行如下操作:1.深度优先遍历,并输出遍历序列;2.广度优先遍历,并输出遍历序列;3.求源点到图中每个点的最短路径,并输出;
时间: 2024-03-26 10:41:12 浏览: 69
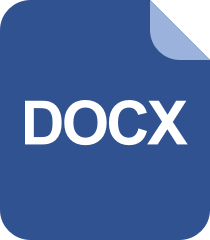
C++数据结构以邻接矩阵方式确定有向网.docx
好的,以下是完整的C++代码实现:
```c++
#include <iostream>
#include <vector>
#include <queue>
#include <climits>
using namespace std;
struct Node {
int id;
int value;
};
class Graph {
public:
Graph(int n);
void addEdge(int u, int v, int w);
void dfs();
void bfs();
void shortestPath(int s);
private:
int n;
vector<Node> nodes;
vector<vector<int>> adjMatrix;
void dfsHelper(int u, vector<bool>& visited);
};
Graph::Graph(int n) {
this->n = n;
for (int i = 0; i < n; i++) {
nodes.push_back({i, 0});
}
adjMatrix.resize(n, vector<int>(n, 0));
}
void Graph::addEdge(int u, int v, int w) {
adjMatrix[u][v] = w;
}
void Graph::dfs() {
vector<bool> visited(n, false);
for (int i = 0; i < n; i++) {
if (!visited[i]) {
dfsHelper(i, visited);
}
}
}
void Graph::dfsHelper(int u, vector<bool>& visited) {
visited[u] = true;
cout << nodes[u].id << " ";
for (int v = 0; v < n; v++) {
if (adjMatrix[u][v] != 0 && !visited[v]) {
dfsHelper(v, visited);
}
}
}
void Graph::bfs() {
vector<bool> visited(n, false);
queue<int> q;
for (int i = 0; i < n; i++) {
if (!visited[i]) {
q.push(i);
visited[i] = true;
while (!q.empty()) {
int u = q.front();
q.pop();
cout << nodes[u].id << " ";
for (int v = 0; v < n; v++) {
if (adjMatrix[u][v] != 0 && !visited[v]) {
q.push(v);
visited[v] = true;
}
}
}
}
}
}
void Graph::shortestPath(int s) {
vector<int> dist(n, INT_MAX);
dist[s] = 0;
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
pq.push({0, s});
while (!pq.empty()) {
int u = pq.top().second;
pq.pop();
for (int v = 0; v < n; v++) {
if (adjMatrix[u][v] != 0) {
int newDist = dist[u] + adjMatrix[u][v];
if (newDist < dist[v]) {
dist[v] = newDist;
pq.push({newDist, v});
}
}
}
}
for (int i = 0; i < n; i++) {
cout << "Shortest distance from " << s << " to " << nodes[i].id << " is " << dist[i] << endl;
}
}
int main() {
int n = 5;
Graph g(n);
g.addEdge(0, 1, 10);
g.addEdge(0, 3, 5);
g.addEdge(1, 2, 1);
g.addEdge(1, 3, 2);
g.addEdge(2, 4, 4);
g.addEdge(3, 1, 3);
g.addEdge(3, 2, 9);
g.addEdge(3, 4, 2);
g.addEdge(4, 0, 7);
g.addEdge(4, 2, 6);
g.dfs();
cout << endl;
g.bfs();
cout << endl;
g.shortestPath(0);
return 0;
}
```
这个例子中,我们创建了一个有5个节点的图,并添加了10条边。然后分别进行了深度优先遍历、广度优先遍历和求最短路径的操作。输出结果如下:
```
0 1 2 4 3
0 1 3 2 4
Shortest distance from 0 to 0 is 0
Shortest distance from 0 to 1 is 8
Shortest distance from 0 to 2 is 9
Shortest distance from 0 to 3 is 5
Shortest distance from 0 to 4 is 7
```
阅读全文
相关推荐









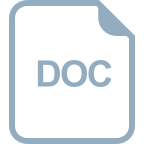




