有 n 个人围成一圈,顺序排号。从第 1 个人开始报数(从 1 到 3 报数), 凡报到 3 的人退出圈子,问最后留下的是原来第几号的人。c 语言
时间: 2023-12-23 08:08:53 浏览: 29
好的,这是一个经典的约瑟夫问题,可以使用循环链表来解决。以下是 C 语言的解法:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义节点结构体
struct Node {
int data;
struct Node* next;
};
// 创建循环链表
struct Node* createCircularList(int n) {
struct Node *head, *tail, *p;
head = (struct Node*) malloc(sizeof(struct Node));
tail = head;
for (int i = 1; i <= n; i++) {
p = (struct Node*) malloc(sizeof(struct Node));
p->data = i;
tail->next = p;
tail = p;
}
tail->next = head->next;
free(head);
return tail->next;
}
// 解决约瑟夫问题
int josephus(struct Node* head, int m) {
struct Node *p, *q;
p = head;
while (p->next != p) {
for (int i = 1; i < m; i++) {
q = p;
p = p->next;
}
q->next = p->next;
free(p);
p = q->next;
}
return p->data;
}
int main() {
int n, m;
printf("请输入人数 n 和报数 m:");
scanf("%d %d", &n, &m);
struct Node* head = createCircularList(n);
int result = josephus(head, m);
printf("最后留下的人是原来第 %d 号的人", result);
return 0;
}
```
代码解释:
1. `createCircularList` 函数用于创建一个由 `n` 个节点组成的循环链表,返回链表头节点的指针。
2. `josephus` 函数用于解决约瑟夫问题,输入链表头节点的指针和报数的数字 `m`,返回最后留下的人的编号。
3. `main` 函数中先输入人数 `n` 和报数 `m`,然后调用 `createCircularList` 函数创建循环链表,最后调用 `josephus` 函数解决问题并输出结果。
希望能帮到你!
相关推荐
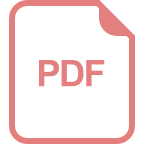
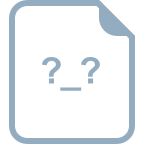















