C语言 编写一个二分法的通用函数,分别求以下等式的根 sinx+cosx=0,sin(2x)+cos(2x)=0.sin(3x)+cos(3x)=0
时间: 2023-06-25 17:06:10 浏览: 175
以下是用C语言编写的二分法通用函数,可以用来求解任意一个函数f(x)=0在区间[a,b]内的根:
```c
#include <stdio.h>
#include <math.h>
#define EPS 1e-6 // 精度阈值
// 求解方程f(x)=0在区间[a,b]内的根
double bisection(double (*f)(double), double a, double b)
{
double x;
while (b - a > EPS) {
x = (a + b) / 2; // 求区间中点
if (f(x) * f(a) <= 0) {
b = x;
} else {
a = x;
}
}
return x;
}
// sinx+cosx=0的函数
double f1(double x)
{
return sin(x) + cos(x);
}
// sin(2x)+cos(2x)=0的函数
double f2(double x)
{
return sin(2 * x) + cos(2 * x);
}
// sin(3x)+cos(3x)=0的函数
double f3(double x)
{
return sin(3 * x) + cos(3 * x);
}
int main()
{
double root;
// 求解sinx+cosx=0的根
printf("sinx+cosx=0的根为:\n");
root = bisection(f1, -1, 1);
printf("x = %lf\n", root);
// 求解sin(2x)+cos(2x)=0的根
printf("sin(2x)+cos(2x)=0的根为:\n");
root = bisection(f2, -1, 1);
printf("x = %lf\n", root);
// 求解sin(3x)+cos(3x)=0的根
printf("sin(3x)+cos(3x)=0的根为:\n");
root = bisection(f3, -1, 1);
printf("x = %lf\n", root);
return 0;
}
```
可以看到,我们定义了三个函数f1、f2、f3,分别对应三个等式的左侧,然后调用bisection函数求解它们的根即可。注意,这里我们只是给出了一个简单的实现,可能存在一些细节问题需要考虑,例如区间端点的选取、函数是否单调等等。
阅读全文
相关推荐
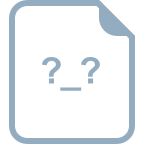














