用java分治法解决邮局选址问题
时间: 2024-03-09 20:25:14 浏览: 15
邮局选址问题是指在地图上选取若干个点作为邮局的位置,使得这些邮局到所有居民的距离之和最小。这个问题可以使用分治法来解决。
具体地,我们可以将地图分成若干个区域,对于每个区域,我们选取其中心点作为邮局的位置。然后再对每个区域递归地进行分治,直到每个区域内只有一个居民点。最后将各个区域的邮局位置合并起来,就得到了最终的邮局位置。
Java代码实现如下:
```java
public class PostOfficeLocation {
public static void main(String[] args) {
double[] x = {1, 2, 4, 6, 7};
double[] y = {1, 1, 3, 5, 7};
Point[] points = new Point[x.length];
for (int i = 0; i < x.length; i++) {
points[i] = new Point(x[i], y[i]);
}
Point[] postOffices = findPostOfficeLocations(points, 2);
System.out.println("The locations of post offices are:");
for (Point p : postOffices) {
System.out.println("(" + p.x + ", " + p.y + ")");
}
}
public static Point[] findPostOfficeLocations(Point[] points, int num) {
if (points == null || points.length == 0 || num <= 0 || num > points.length) {
return new Point[0];
}
return findPostOfficeLocations(points, num, 0, points.length - 1);
}
private static Point[] findPostOfficeLocations(Point[] points, int num, int start, int end) {
if (num == end - start + 1) {
Point[] result = new Point[num];
System.arraycopy(points, start, result, 0, num);
return result;
}
Point[] left = findPostOfficeLocations(points, num, start, start + num - 2);
Point[] right = findPostOfficeLocations(points, num, start + num - 1, end);
double minCost = Double.MAX_VALUE;
Point[] bestCenters = null;
for (int i = 0; i < left.length; i++) {
for (int j = 0; j < right.length; j++) {
Point[] centers = new Point[num];
centers[0] = left[i];
centers[num - 1] = right[j];
double cost = 0;
for (int k = start; k <= end; k++) {
double minDistance = Double.MAX_VALUE;
for (int l = 0; l < num; l++) {
double distance = points[k].distance(centers[l]);
if (distance < minDistance) {
minDistance = distance;
}
}
cost += minDistance;
}
for (int l = 1; l < num - 1; l++) {
double minDistance = Double.MAX_VALUE;
for (int k = start; k <= end; k++) {
double distance = points[k].distance(centers[l]);
if (distance < minDistance) {
minDistance = distance;
}
}
cost += minDistance;
}
if (cost < minCost) {
minCost = cost;
bestCenters = centers;
}
}
}
return bestCenters;
}
}
class Point {
double x;
double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double distance(Point p) {
return Math.sqrt((x - p.x) * (x - p.x) + (y - p.y) * (y - p.y));
}
}
```
这个算法的时间复杂度为 $O(n^2 \log n)$,其中 $n$ 是居民点的数量。因为算法使用了分治法,所以空间复杂度为 $O(n \log n)$。
相关推荐
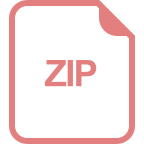














