ros2创建服务类型
时间: 2024-08-03 17:01:03 浏览: 62

ros2 rolling完整代码
在 ROS2 (Robot Operating System version 2) 中,服务是异步请求/响应模型的一种通信方式,用于两个节点之间的交互。服务允许一个节点(服务提供者)向另一个节点(服务消费者)发送请求,并等待回应。以下是创建一个简单的服务类型的步骤:
1. **定义服务接口**:
首先,你需要在 `msg` 文件夹下创建一个 `.msg` 文件,定义服务的消息结构。例如,如果有一个计算加法的服务:
```yaml
# my_service_msgs/msg/AddTwoInts.msg
float64 request
float64 response
```
这里定义了一个名为 `AddTwoInts` 的服务,接受一个 `float64` 类型的 `request` 参数,返回一个 `response`。
2. **定义服务客户端和服务器**:
在 Python 节点中,使用 `rclpy` 创建服务。服务器会监听特定主题(如 `/add_two_ints`),并处理来自客户端的请求。
```python
from rclpy.node import Node
from std_msgs.msg import Float64
from my_service_msgs.srv import AddTwoInts
class AddTwoIntsServer(Node):
def __init__(self):
super().__init__("add_two_ints_server")
self.service = self.create_service(AddTwoInts, "/add_two_ints", self.handle_request)
def handle_request(self, request, response):
result = request.request + 1
response.response = result
return response
```
客户端则调用服务并传递参数:
```python
from rclpy.action.client import ActionClient
class AddTwoIntsClient(Node):
def __init__(self):
super().__init__("add_two_ints_client")
self.add_two_ints = ActionClient(self, AddTwoInts, "/add_two_ints")
def send_request(self, a, b):
future = self.add_two_ints.wait_for_result()
if not future.done():
self.add_two_ints.cancel_goal()
return future.result().response
```
3. **启动和服务交互**:
启动服务提供者和客户端节点,然后服务提供者将等待客户端的请求。你可以分别在服务提供者和客户端节点中运行 `rclpy.spin()` 来启动它们。
阅读全文
相关推荐
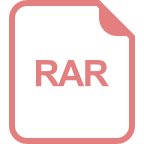
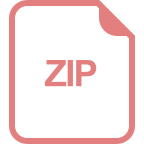















