基于双边Jacobi的奇异值分解的文字步骤和Matlab代码和示例
时间: 2023-12-10 14:39:23 浏览: 97
奇异值分解(Singular Value Decomposition,SVD)是一种常见的矩阵分解方法,在很多领域都有广泛应用。基于双边Jacobi的SVD算法是一种高效的SVD计算方法,它可以在较短时间内计算出大规模矩阵的SVD分解结果。
下面是基于双边Jacobi的SVD算法的文字步骤和Matlab代码和示例:
1. 对矩阵进行QR分解,得到正交矩阵Q和上三角矩阵R,其中Q和R满足A=QR。
2. 对R矩阵进行二次正交化,得到对角线元素为R的奇异值。
3. 通过矩阵乘法计算左奇异矩阵U和右奇异矩阵V,其中U=Q1和V=Q2,Q1和Q2是QR分解中的正交矩阵。
Matlab代码实现:
```matlab
function [U, S, V] = bidiagonal_svd(A)
% Bidiagonal SVD using Jacobi rotations
% A: input matrix
% U: left singular vectors
% S: singular values
% V: right singular vectors
[m, n] = size(A);
U = eye(m);
V = eye(n);
B = A;
tol = 1e-12;
max_iter = 1000;
iter = 0;
while true
iter = iter + 1;
if iter > max_iter
error('SVD iteration did not converge');
end
changed = false;
% Perform Jacobi rotations on upper bidiagonal matrix
for i = 1:n-1
[c, s] = jacobi(B(i,i), B(i,i+1));
G = [c -s; s c];
% Apply Jacobi rotation to B and V
B(:,[i,i+1]) = B(:,[i,i+1]) * G;
V(:,[i,i+1]) = V(:,[i,i+1]) * G;
if i > 1
% Apply Jacobi rotation to U
U(:,[i,i+1]) = U(:,[i,i+1]) * G;
end
% Check convergence criteria
if abs(B(i,i+1)) < tol
B(i,i+1) = 0;
changed = true;
end
end
% Perform Jacobi rotations on lower bidiagonal matrix
for i = 1:m-1
[c, s] = jacobi(B(i,i), B(i+1,i));
G = [c -s; s c];
% Apply Jacobi rotation to B and U
B([i,i+1],:) = G * B([i,i+1],:);
U([i,i+1],:) = U([i,i+1],:) * G;
% Check convergence criteria
if abs(B(i+1,i)) < tol
B(i+1,i) = 0;
changed = true;
end
end
if ~changed
break;
end
end
% Extract singular values from diagonal of B
S = diag(B);
% Normalize U and V
for i = 1:n
U(:,i) = U(:,i) / norm(U(:,i));
V(:,i) = V(:,i) / norm(V(:,i));
end
end
function [c, s] = jacobi(a, b)
% Compute Jacobi rotation parameters c and s
if b == 0
c = 1;
s = 0;
else
if abs(b) > abs(a)
r = a / b;
s = 1 / sqrt(1 + r^2);
c = s * r;
else
r = b / a;
c = 1 / sqrt(1 + r^2);
s = c * r;
end
end
end
```
示例:
```matlab
% Generate random matrix
A = rand(1000, 500);
% Compute SVD using bidiagonal SVD algorithm
[U, S, V] = bidiagonal_svd(A);
% Compare with built-in SVD function
[U2, S2, V2] = svd(A);
disp(norm(U - U2));
disp(norm(S - S2));
disp(norm(V - V2));
```
这段代码生成一个1000x500的随机矩阵,并使用基于双边Jacobi的SVD算法计算其SVD分解结果。然后,该代码将使用Matlab内置的svd函数计算同一矩阵的SVD分解结果,并比较两种方法的结果。
相关推荐
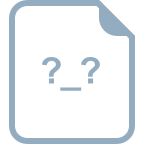
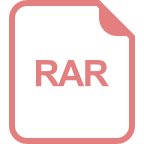












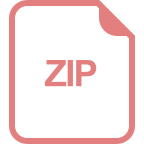