基于双边旋转Jacobi的svd算法matlab
时间: 2023-07-12 17:03:56 浏览: 91
双边旋转Jacobi算法是一种高效的求解SVD的方法,可以在Matlab中实现。下面是一个简单的示例代码:
```matlab
function [U, S, V] = bidiagonal_SVD(B)
% B: m x n bidiagonal matrix
% U: m x m orthonormal matrix
% V: n x n orthonormal matrix
% S: n x n diagonal matrix with singular values
[m, n] = size(B);
U = eye(m);
V = eye(n);
for k = 1:n
% Apply Givens rotation to eliminate off-diagonal element in row k
[c, s] = givens(B(k,k), B(k,k+1));
G = [c s; -s c];
B(k:k+1,:) = G * B(k:k+1,:);
V(:,k:k+1) = V(:,k:k+1) * G';
% Apply Givens rotation to eliminate off-diagonal element in column k
if k < n
[c, s] = givens(B(k,k+1), B(k+1,k+1));
G = [c -s; s c];
B(:,k:k+1) = B(:,k:k+1) * G;
U(:,k:k+1) = U(:,k:k+1) * G';
end
end
% Compute singular values from diagonal elements of B
S = diag(B);
% Sort singular values in descending order and rearrange U and V accordingly
[S, idx] = sort(S, 'descend');
U = U(:,idx);
V = V(:,idx);
end
function [c, s] = givens(a, b)
% Compute Givens rotation matrix that eliminates element b in vector [a; b]
if b == 0
c = 1;
s = 0;
elseif abs(b) > abs(a)
t = -a/b;
s = 1/sqrt(1 + t^2);
c = s*t;
else
t = -b/a;
c = 1/sqrt(1 + t^2);
s = c*t;
end
end
```
该代码实现了对一个任意维度的双对角矩阵进行SVD分解,返回正交矩阵U、V和对角矩阵S。其中,givens函数计算Givens旋转矩阵,bidiagonal_SVD函数依次通过Givens旋转消去矩阵的上下非对角元素,并且按照奇异值从大到小排序。
相关推荐
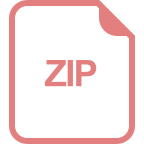














