使用PX4和mavros编写无人机在各种模式下的例程代码,如offboard、mission、takeoff、land、return、hold等模式
时间: 2024-02-01 10:04:40 浏览: 34
以下是使用PX4和mavros编写无人机在各种模式下的例程代码:
1. Offboard模式:
```
import rospy
from mavros_msgs.msg import *
from mavros_msgs.srv import *
def set_mode(mode):
rospy.wait_for_service('/mavros/set_mode')
try:
flight_mode = rospy.ServiceProxy('/mavros/set_mode', SetMode)
flight_mode(custom_mode=mode)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_offboard_mode():
set_mode("OFFBOARD")
def set_arm():
rospy.wait_for_service('/mavros/cmd/arming')
try:
arm = rospy.ServiceProxy('/mavros/cmd/arming', CommandBool)
arm(True)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_disarm():
rospy.wait_for_service('/mavros/cmd/arming')
try:
arm = rospy.ServiceProxy('/mavros/cmd/arming', CommandBool)
arm(False)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_attitude(roll, pitch, yaw, thrust):
rospy.wait_for_service('/mavros/cmd/command')
try:
set_att = rospy.ServiceProxy('/mavros/cmd/command', CommandLong)
set_att(0, 0, 186, 0, 0, 0, roll, pitch, yaw, thrust)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def offboard():
rospy.init_node('offboard_node', anonymous=True)
rate = rospy.Rate(20)
set_offboard_mode()
set_arm()
while not rospy.is_shutdown():
set_attitude(0, 0, 0, 0.5)
rate.sleep()
set_disarm()
```
2. Mission模式:
```
import rospy
from mavros_msgs.msg import *
from mavros_msgs.srv import *
def set_mode(mode):
rospy.wait_for_service('/mavros/set_mode')
try:
flight_mode = rospy.ServiceProxy('/mavros/set_mode', SetMode)
flight_mode(custom_mode=mode)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_arm():
rospy.wait_for_service('/mavros/cmd/arming')
try:
arm = rospy.ServiceProxy('/mavros/cmd/arming', CommandBool)
arm(True)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_disarm():
rospy.wait_for_service('/mavros/cmd/arming')
try:
arm = rospy.ServiceProxy('/mavros/cmd/arming', CommandBool)
arm(False)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_waypoint(x, y, z):
rospy.wait_for_service('/mavros/cmd/mission/push')
try:
set_wp = rospy.ServiceProxy('/mavros/cmd/mission/push', WaypointPush)
wp = Waypoint()
wp.frame = 3
wp.command = 16
wp.is_current = False
wp.autocontinue = True
wp.param1 = 0
wp.param2 = 0
wp.param3 = 0
wp.param4 = 0
wp.x_lat = x
wp.y_long = y
wp.z_alt = z
set_wp(start_index=0, waypoints=[wp])
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_mission_mode():
set_mode("AUTO.MISSION")
def mission():
rospy.init_node('mission_node', anonymous=True)
rate = rospy.Rate(20)
set_arm()
set_waypoint(0, 0, 5)
set_waypoint(10, 0, 5)
set_waypoint(10, 10, 5)
set_waypoint(0, 10, 5)
set_waypoint(0, 0, 5)
set_mission_mode()
while not rospy.is_shutdown():
rate.sleep()
set_disarm()
```
3. Takeoff模式:
```
import rospy
from mavros_msgs.msg import *
from mavros_msgs.srv import *
def set_mode(mode):
rospy.wait_for_service('/mavros/set_mode')
try:
flight_mode = rospy.ServiceProxy('/mavros/set_mode', SetMode)
flight_mode(custom_mode=mode)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_arm():
rospy.wait_for_service('/mavros/cmd/arming')
try:
arm = rospy.ServiceProxy('/mavros/cmd/arming', CommandBool)
arm(True)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_disarm():
rospy.wait_for_service('/mavros/cmd/arming')
try:
arm = rospy.ServiceProxy('/mavros/cmd/arming', CommandBool)
arm(False)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_takeoff_altitude(altitude):
rospy.wait_for_service('/mavros/cmd/takeoff')
try:
takeoff = rospy.ServiceProxy('/mavros/cmd/takeoff', CommandTOL)
takeoff(min_pitch=0, yaw=0, latitude=0, longitude=0, altitude=altitude)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def takeoff():
rospy.init_node('takeoff_node', anonymous=True)
rate = rospy.Rate(20)
set_arm()
set_takeoff_altitude(5)
set_mode("AUTO.TAKEOFF")
while not rospy.is_shutdown():
rate.sleep()
set_disarm()
```
4. Land模式:
```
import rospy
from mavros_msgs.msg import *
from mavros_msgs.srv import *
def set_mode(mode):
rospy.wait_for_service('/mavros/set_mode')
try:
flight_mode = rospy.ServiceProxy('/mavros/set_mode', SetMode)
flight_mode(custom_mode=mode)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_arm():
rospy.wait_for_service('/mavros/cmd/arming')
try:
arm = rospy.ServiceProxy('/mavros/cmd/arming', CommandBool)
arm(True)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_disarm():
rospy.wait_for_service('/mavros/cmd/arming')
try:
arm = rospy.ServiceProxy('/mavros/cmd/arming', CommandBool)
arm(False)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_land():
rospy.wait_for_service('/mavros/cmd/land')
try:
land = rospy.ServiceProxy('/mavros/cmd/land', CommandTOL)
land(min_pitch=0, yaw=0, latitude=0, longitude=0, altitude=0)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def land():
rospy.init_node('land_node', anonymous=True)
rate = rospy.Rate(20)
set_arm()
set_mode("AUTO.LAND")
set_land()
while not rospy.is_shutdown():
rate.sleep()
set_disarm()
```
5. Return模式:
```
import rospy
from mavros_msgs.msg import *
from mavros_msgs.srv import *
def set_mode(mode):
rospy.wait_for_service('/mavros/set_mode')
try:
flight_mode = rospy.ServiceProxy('/mavros/set_mode', SetMode)
flight_mode(custom_mode=mode)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_arm():
rospy.wait_for_service('/mavros/cmd/arming')
try:
arm = rospy.ServiceProxy('/mavros/cmd/arming', CommandBool)
arm(True)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_disarm():
rospy.wait_for_service('/mavros/cmd/arming')
try:
arm = rospy.ServiceProxy('/mavros/cmd/arming', CommandBool)
arm(False)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_return():
rospy.wait_for_service('/mavros/cmd/rtl')
try:
rtl = rospy.ServiceProxy('/mavros/cmd/rtl', CommandTOL)
rtl(min_pitch=0, yaw=0, latitude=0, longitude=0, altitude=0)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def return_home():
rospy.init_node('return_node', anonymous=True)
rate = rospy.Rate(20)
set_arm()
set_mode("AUTO.RTL")
set_return()
while not rospy.is_shutdown():
rate.sleep()
set_disarm()
```
6. Hold模式:
```
import rospy
from mavros_msgs.msg import *
from mavros_msgs.srv import *
def set_mode(mode):
rospy.wait_for_service('/mavros/set_mode')
try:
flight_mode = rospy.ServiceProxy('/mavros/set_mode', SetMode)
flight_mode(custom_mode=mode)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_arm():
rospy.wait_for_service('/mavros/cmd/arming')
try:
arm = rospy.ServiceProxy('/mavros/cmd/arming', CommandBool)
arm(True)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_disarm():
rospy.wait_for_service('/mavros/cmd/arming')
try:
arm = rospy.ServiceProxy('/mavros/cmd/arming', CommandBool)
arm(False)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def set_hold():
rospy.wait_for_service('/mavros/cmd/command')
try:
set_att = rospy.ServiceProxy('/mavros/cmd/command', CommandLong)
set_att(0, 0, 186, 0, 0, 0, 0, 0, 0, 0)
except rospy.ServiceException as e:
print("Service call failed: %s"%e)
def hold():
rospy.init_node('hold_node', anonymous=True)
rate = rospy.Rate(20)
set_arm()
set_mode("AUTO.LOITER")
set_hold()
while not rospy.is_shutdown():
rate.sleep()
set_disarm()
```
注意:以上代码仅供参考,具体实现可能需要根据不同的硬件、软件版本进行修改。
相关推荐
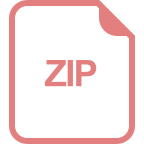
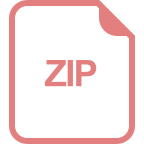
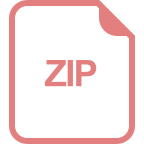














