python time series predict model multi example
时间: 2024-05-10 09:20:25 浏览: 18
Here is an example of a Python time series prediction model using multiple variables:
```
# Import necessary libraries
import pandas as pd
import numpy as np
from sklearn.linear_model import LinearRegression
# Load the dataset
df = pd.read_csv('sales_data.csv', parse_dates=['Date'])
# Create new features
df['Year'] = df['Date'].dt.year
df['Month'] = df['Date'].dt.month
df['Day'] = df['Date'].dt.day
df['Weekday'] = df['Date'].dt.weekday
# Split the data into training and testing sets
train = df[df['Year'] < 2017]
test = df[df['Year'] >= 2017]
# Define the input and output variables
X_train = train[['Month', 'Day', 'Weekday']]
y_train = train['Sales']
X_test = test[['Month', 'Day', 'Weekday']]
y_test = test['Sales']
# Create and fit the model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
from sklearn.metrics import mean_absolute_error
mae = mean_absolute_error(y_test, y_pred)
print("MAE:", mae)
```
In this example, we are predicting sales using the month, day, and weekday as input variables. We split the data into training and testing sets, create the model using linear regression, and evaluate the model using the mean absolute error metric. This is just one example of how you can build a time series prediction model using multiple variables in Python.
相关推荐
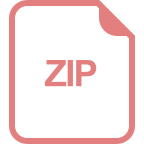
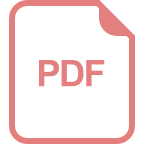














