把一个点云订阅和发布节点封装为ros service服务端,当客户端发送请求时,服务端开始订阅和发布,之后关掉服务端,c++代码
时间: 2024-05-01 22:22:15 浏览: 156
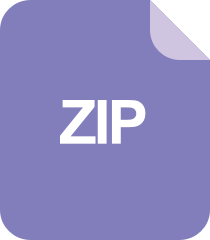
ROS发布者publisher和订阅者subscriber编程实现,C++实验代码
以下是一个简单的将点云订阅和发布封装为ROS服务的C++代码示例:
```cpp
#include <ros/ros.h>
#include <sensor_msgs/PointCloud2.h>
#include <pcl_conversions/pcl_conversions.h>
bool handlePointCloudService(std_srvs::Empty::Request& req, std_srvs::Empty::Response& res)
{
// Create a node handle to communicate with ROS
ros::NodeHandle nh;
// Subscribe to the input point cloud topic
ros::Subscriber sub = nh.subscribe<sensor_msgs::PointCloud2>("input_cloud_topic", 1, boost::function<void(const sensor_msgs::PointCloud2::ConstPtr&)>([&](const sensor_msgs::PointCloud2::ConstPtr& input_cloud) {
// Create a publisher for the output point cloud topic
ros::Publisher pub = nh.advertise<sensor_msgs::PointCloud2>("output_cloud_topic", 1);
// Convert the input point cloud to PCL format
pcl::PCLPointCloud2 pcl_input_cloud;
pcl_conversions::toPCL(*input_cloud, pcl_input_cloud);
pcl::PointCloud<pcl::PointXYZ>::Ptr pcl_input_cloud_xyz(new pcl::PointCloud<pcl::PointXYZ>);
pcl::fromPCLPointCloud2(pcl_input_cloud, *pcl_input_cloud_xyz);
// Process the point cloud (e.g. apply some filter)
pcl::PointCloud<pcl::PointXYZ>::Ptr pcl_output_cloud_xyz(new pcl::PointCloud<pcl::PointXYZ>);
// ...
// Convert the output point cloud from PCL format to ROS format
sensor_msgs::PointCloud2 output_cloud;
pcl::toROSMsg(*pcl_output_cloud_xyz, output_cloud);
// Publish the output point cloud
pub.publish(output_cloud);
// Shutdown the subscriber and publisher
sub.shutdown();
pub.shutdown();
}));
// Spin until the subscriber receives a point cloud
ros::spin();
return true;
}
int main(int argc, char** argv)
{
// Initialize the ROS node
ros::init(argc, argv, "point_cloud_service");
// Create a node handle to advertise the service
ros::NodeHandle nh;
// Advertise the service
ros::ServiceServer service = nh.advertiseService("point_cloud_service", handlePointCloudService);
// Spin
ros::spin();
return 0;
}
```
在上面的代码中,我们首先定义了一个名为 `handlePointCloudService` 的回调函数,用于处理服务请求。当客户端发送请求时,该函数会创建一个ROS节点并订阅输入点云主题。一旦接收到点云,它会执行一些处理,然后将结果发布到输出点云主题。最后,它会关闭订阅器和发布器并返回服务响应。
然后,在 `main` 函数中,我们初始化了一个ROS节点,并通过它来创建一个名为 `point_cloud_service` 的服务。我们将 `handlePointCloudService` 函数作为服务回调函数,这意味着当客户端发送请求时,服务将调用该函数。
最后,我们开始ROS循环并等待服务请求。
阅读全文
相关推荐

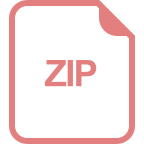
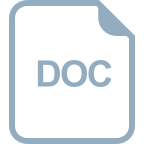







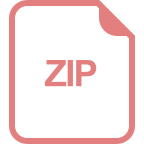
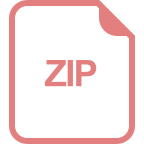
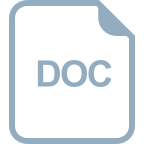


