请利用栈将随机输入的中缀算术表达式转换为后缀表达式,并计算表达式的值。中缀和后缀表达式对应的字符串长度不超过50。请提交实验代码和结果截图。要适用于Dev-c++。
时间: 2024-12-24 22:22:52 浏览: 7
中缀表达式转后缀表达式(也称为逆波兰表示法,RPN)的过程通常涉及以下几个步骤:
1. 创建一个空栈和一个空列表用于存放后缀表达式。
2. 遍历中缀表达式的每个字符:
- 如果遇到操作数(数字),直接将其添加到后缀表达式列表中。
- 如果遇到运算符,检查它是否有优先级高于当前栈顶的运算符。如果没有,就把它压入栈;如果有,则持续弹出栈顶的运算符直到找到一个低优先级的,然后一起压入栈,最后压入当前运算符。
3. 当遍历完所有字符后,如果栈非空,继续处理剩余的运算符,因为可能存在优先级相同的运算符需要一起处理。
4. 最后,栈中剩下的都是操作数,从栈中依次取出并添加到后缀表达式列表中。
对于Dev-c++,你可以使用`std::stack`来实现这个过程。这是一个简单的算法示例,你需要根据实际需求调整代码以处理更大规模的输入:
```cpp
#include <iostream>
#include <string>
#include <stack>
#include <sstream>
int precedence(char op) {
// 填充运算符优先级表...
}
std::string infixToPostfix(const std::string& expression) {
std::stack<char> ops;
std::string postfix;
for (char c : expression) {
if ('0' <= c && c <= '9') { // 操作数
postfix += c;
} else if (c == '+' || c == '-' || ...){ // 运算符
while (!ops.empty() && precedence(c) <= precedence(ops.top())) {
postfix += ops.top();
ops.pop();
}
ops.push(c);
}
}
while (!ops.empty()) {
postfix += ops.top();
ops.pop();
}
return postfix;
}
int evaluatePostfix(const std::string& postfix) {
std::stack<int> stack;
for (char c : postfix) {
if ('0' <= c && c <= '9') { // 加入数字到栈
int num = 0;
while (isdigit(c)) {
num *= 10;
num += c - '0';
c = getchar(); // 假设getchar函数获取下一个字符
}
stack.push(num);
} else { // 执行运算
int right = stack.top(); stack.pop();
int left = stack.top(); stack.pop();
switch (c) {
case '+': stack.push(left + right); break;
case '-': stack.push(left - right); break;
// 更多运算符...
}
}
}
return stack.top();
}
int main() {
std::string infix;
std::cout << "Enter an infix expression (max length 50): ";
getline(std::cin, infix);
if (infix.length() > 50) {
std::cerr << "Expression too long. Limit is 50 characters." << std::endl;
return 1;
}
std::string postfix = infixToPostfix(infix);
int result = evaluatePostfix(postfix);
std::cout << "Result of the postfix expression: " << result << std::endl;
// 提供一个实验代码截图是不可能的,但你可以运行程序并在控制台看到结果。
return 0;
}
```
阅读全文
相关推荐
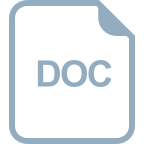
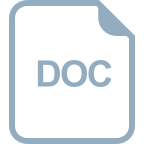
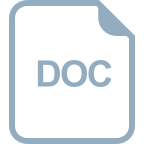

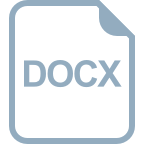




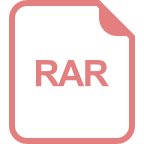
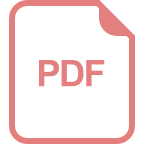
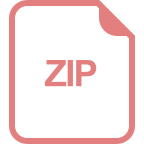







