ue5 c++头文件
时间: 2024-09-25 22:08:36 浏览: 61
在Unreal Engine 5 (UE5) 的C++开发中,头文件(Header Files)是非常重要的组成部分,它们包含了函数、变量、结构体和类的声明,而不包含它们的具体实现。当你在UE5项目中编写C++代码时,通常会包含相关的头文件以便其他模块能够引用并使用这些声明。
例如,如果你有一个自定义的类`MyClass`,你会在一个`.h`或`.hpp`(有时也用.hx)文件中写下它的声明:
```cpp
// MyClass.h
#pragma once
class MyClass
{
public:
// 成员函数声明
void MyFunction();
virtual float GetSomeValue() const;
private:
// 类成员变量声明
int m私下变量;
};
```
然后在另一个源文件(`.cpp`)中,你可以包含这个头文件,并使用这个类:
```cpp
// MyClass.cpp
#include "MyClass.h"
void MyClass::MyFunction()
{
// 实现细节...
}
float MyClass::GetSomeValue() const
{
// 具体的实现...
}
```
相关问题
ue5 c++ FRuntimeAssetCache 需要 include 什么头文件 且如何修改build文件
在使用 `FRuntimeAssetCache` 的时候,需要包含头文件 "RuntimeAssetCache.h"。在使用前可以通过以下方式引入:
```c++
#include "RuntimeAssetCache.h"
```
同时,需要在构建文件中添加对 `RuntimeAssetCache` 模块的引用,以及将 `RuntimeAssetCache` 模块的路径添加到 `PublicIncludePaths` 中。示例代码如下:
```c++
using UnrealBuildTool;
public class MyProject : ModuleRules
{
public MyProject(ReadOnlyTargetRules Target) : base(Target)
{
PrivateDependencyModuleNames.AddRange(new string[] { "Core", "CoreUObject", "Engine", "InputCore" });
PublicDependencyModuleNames.AddRange(new string[] { "RuntimeAssetCache" });
PublicIncludePaths.Add(ModuleDirectory + "/Public");
}
}
```
在 `PublicDependencyModuleNames` 中添加 "RuntimeAssetCache" 模块,表示依赖该模块。在 `PublicIncludePaths` 中添加 `RuntimeAssetCache` 模块的公共头文件路径,以便在代码中包含 "RuntimeAssetCache.h"。
注意:如果 `RuntimeAssetCache` 是一个插件,而不是项目的一部分,那么需要将 `RuntimeAssetCache` 插件添加到项目中并启用它。在插件的构建文件中,可以添加以下代码将插件添加到项目中:
```c++
PublicDependencyModuleNames.AddRange(new string[] { "RuntimeAssetCache" });
```
完整的示例代码如下:
```c++
using UnrealBuildTool;
public class MyProject : ModuleRules
{
public MyProject(ReadOnlyTargetRules Target) : base(Target)
{
PrivateDependencyModuleNames.AddRange(new string[] { "Core", "CoreUObject", "Engine", "InputCore" });
PublicDependencyModuleNames.AddRange(new string[] { "RuntimeAssetCache" });
PublicIncludePaths.Add(ModuleDirectory + "/Public");
}
}
```
UE5 c++ handingmodel
在UE5中,可以使用C++编写自定义的HandingModel。HandlingModel是一种用于控制角色移动和旋转的控制器。以下是创建HandlingModel的步骤:
1.创建一个新的C++类,继承自UE4的ACharacter类。
2.在头文件中声明HandlingModel类,并实现其构造函数和Update方法。
3.实现Update方法来处理角色的移动和旋转。
4.使用HandlingModel类来替换默认的CharacterMovementComponent。
5.在角色类中调用HandlingModel的Update方法,以便在每帧更新角色的位置和方向。
需要注意的是,使用C++编写HandlingModel需要一定的编程知识和经验,需要了解UE4的引擎架构和API。同时,还需要进行针对性的测试和优化,以确保HandlingModel的稳定性和性能。
阅读全文
相关推荐
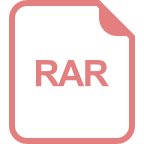
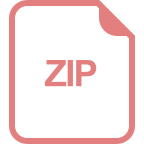
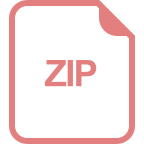













