前序遍历和中序遍历求后序遍历
时间: 2023-09-08 15:08:37 浏览: 74
对于给定的二叉树,前序遍历的顺序是先遍历根节点,然后是左子树,最后是右子树。中序遍历的顺序是先遍历左子树,然后是根节点,最后是右子树。要求后序遍历,可以通过递归的方式进行求解。
下面给出一个实现的例子:
```python
def build_tree(preorder, inorder):
if not preorder or not inorder:
return None
# 获取根节点值
root_val = preorder[0]
root = TreeNode(root_val)
# 在中序遍历中找到根节点的索引位置
idx = inorder.index(root_val)
# 切分左右子树的中序遍历列表
left_inorder = inorder[:idx]
right_inorder = inorder[idx+1:]
# 切分左右子树的前序遍历列表
left_preorder = preorder[1:1+len(left_inorder)]
right_preorder = preorder[1+len(left_inorder):]
# 递归构建左右子树
root.left = build_tree(left_preorder, left_inorder)
root.right = build_tree(right_preorder, right_inorder)
return root
def postorder_traversal(root):
if not root:
return []
result = []
# 递归遍历左子树
if root.left:
result += postorder_traversal(root.left)
# 递归遍历右子树
if root.right:
result += postorder_traversal(root.right)
# 添加当前节点值到结果列表
result.append(root.val)
return result
preorder = [1, 2, 4, 5, 3, 6, 7]
inorder = [4, 2, 5, 1, 6, 3, 7]
root = build_tree(preorder, inorder)
postorder = postorder_traversal(root)
print(postorder)
```
使用前序遍历列表和中序遍历列表构建二叉树,并最终得到后序遍历的结果。以上是一个简单的实现示例,希望能对你有所帮助。
相关推荐
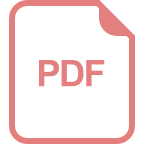
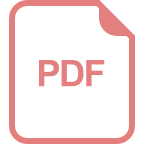
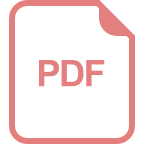
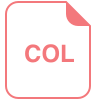
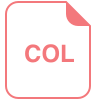
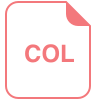
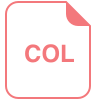
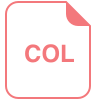









