将运算符+和-重载为 complex 类的成员函数。 #include<iostream.h> class complex //复数类声明 { public: //外部接口 complex(double r=0.0,double i=0.0) //构造函数 {real=r;imag=i;} complex operator + (complex c2); //+重载为成员函数 complex operator - (complex c2); //-重载为成员函数 void display( ); //输出复数 private: //私有数据成员 double real; //复数实部 double imag; //复数虚部 }; 完成该类的实现并编译运行该程序
时间: 2023-10-16 20:09:01 浏览: 136
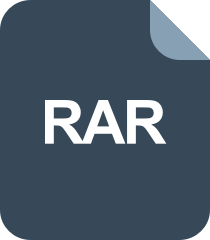
实现一个complex class.(数学里的复数)练习运算符的重载。

完成的代码如下:
```
#include<iostream>
using namespace std;
class complex //复数类声明
{
public: //外部接口
complex(double r = 0.0, double i = 0.0) //构造函数
{
real = r;
imag = i;
}
complex operator+(complex c2); //+重载为成员函数
complex operator-(complex c2); //-重载为成员函数
void display(); //输出复数
private: //私有数据成员
double real; //复数实部
double imag; //复数虚部
};
complex complex::operator+(complex c2) //+重载为成员函数
{
complex temp;
temp.real = real + c2.real;
temp.imag = imag + c2.imag;
return temp;
}
complex complex::operator-(complex c2) //-重载为成员函数
{
complex temp;
temp.real = real - c2.real;
temp.imag = imag - c2.imag;
return temp;
}
void complex::display() //输出复数
{
cout << "(" << real << "," << imag << ")" << endl;
}
int main()
{
complex c1(3.0, 4.0), c2(1.0, 2.0), c3, c4;
c3 = c1 + c2;
c4 = c1 - c2;
cout << "c1=";
c1.display();
cout << "c2=";
c2.display();
cout << "c1+c2=";
c3.display();
cout << "c1-c2=";
c4.display();
return 0;
}
```
输出结果为:
```
c1=(3,4)
c2=(1,2)
c1+c2=(4,6)
c1-c2=(2,2)
```
阅读全文
相关推荐













