ros中如何用python获取当前时间
时间: 2023-12-26 16:04:47 浏览: 178
您可以使用Python中的datetime库来获取当前时间。具体地,您可以使用以下代码来获取当前的日期和时间:
```
import datetime
# 获取当前的日期和时间
now = datetime.datetime.now()
print("当前时间为:", now)
```
您还可以使用strftime函数将日期和时间转换为字符串格式,如下所示:
```
import datetime
# 获取当前的日期和时间,并将其转换为字符串格式
now = datetime.datetime.now()
str_time = now.strftime("%Y-%m-%d %H:%M:%S")
print("当前时间为:", str_time)
```
这将以“年-月-日 时:分:秒”的格式输出当前的日期和时间。
相关问题
ros如何使用python语言获取系统时间
在ROS (Robot Operating System) 中,你可以使用Python语言通过`rospy`库来获取系统时间。首先,你需要安装`rospy`包并确保它已经导入到你的Python脚本中。这里是一个简单的步骤:
1. 导入必要的模块:
```python
import rospy
from std_msgs.msg import Time
```
2. 初始化ROS节点:
```python
rospy.init_node('get_system_time', anonymous=True)
```
3. 创建一个订阅者,订阅`/clock`话题,该话题发布的是`Time`类型的message,包含了系统时间信息:
```python
time_subscriber = rospy.Subscriber('/clock', Time, callback_function)
```
4. 定义回调函数,当接收到`/clock`消息时,从中提取时间数据:
```python
def callback_function(msg):
current_time = msg.sec + msg.nanosec * 1e-9 # 将秒和纳秒转换成浮点数表示
print(f"当前系统时间为:{current_time}秒")
```
5. 开始循环监听主题:
```python
rospy.spin() # 这会阻塞程序直到节点关闭
```
当你运行这个脚本时,它会定期打印出系统的当前时间。
ros1中求时间函数且输出当前时间加和,用python
在ROS(Robot Operating System,版本1)中,你可以使用`rospy`库来处理时间和计算时间间隔。为了获取当前时间并将其累加,你可以使用`rospy.get_time()`函数来获取系统时间戳(以秒为单位),然后在循环中不断累加。
这是一个简单的例子,假设你想每秒打印一次当前时间,并累计总时间:
```python
import rospy
total_time = 0.0
last_print_time = rospy.get_time()
def print_time():
global total_time, last_print_time
current_time = rospy.get_time()
# 计算时间差并累加到总时间
time_since_last_print = current_time - last_print_time
total_time += time_since_last_print
last_print_time = current_time
print(f"Current Time: {current_time:.6f}, Total Elapsed Time: {total_time:.6f} seconds")
# 初始化节点
rospy.init_node('time_counter')
# 定义频率,比如1 Hz(每秒运行一次)
rate = rospy.Rate(1)
while not rospy.is_shutdown():
print_time() # 每秒打印一次并累加时间
rate.sleep() # 等待下一帧
print("-- Shutdown --")
```
在这个脚本中,我们首先初始化一个`rospy`节点,然后创建一个速率计(`Rate`),设置其频率为1 Hz。在主循环中,我们每次通过`print_time`函数更新并打印当前时间和累计时间,然后让主线程稍作休息等待下一轮循环。
阅读全文
相关推荐
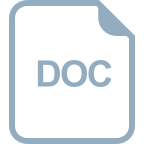
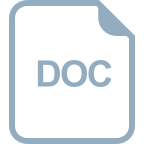
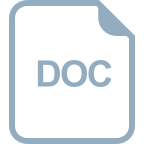
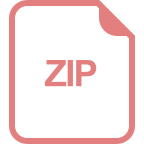
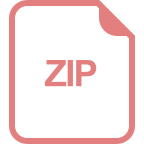
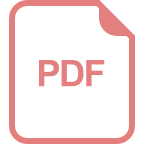
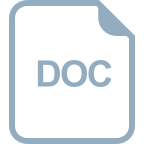
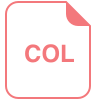








