用windows计时器实现c语言控制台版的贪吃蛇代码实现
时间: 2024-02-27 19:58:21 浏览: 76
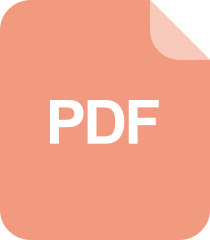
基于C语言实现的贪吃蛇游戏完整实例代码
好的,以下是用 Windows 计时器实现 C 语言控制台版贪吃蛇的代码实现:
```c
#include <stdio.h>
#include <conio.h>
#include <Windows.h>
#include <stdlib.h>
#include <time.h>
#define width 60
#define height 20
enum Direction {UP, DOWN, LEFT, RIGHT};
int snake[width * height][2];
int food[2];
int length = 0;
int score = 0;
int direction = RIGHT;
int speed = 100;
void gotoxy(int x, int y)
{
COORD pos = { x, y };
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void hidecursor()
{
CONSOLE_CURSOR_INFO cursor_info = { 1, 0 };
SetConsoleCursorInfo(GetStdHandle(STD_OUTPUT_HANDLE), &cursor_info);
}
void initSnake()
{
int x = width / 2, y = height / 2;
for (int i = 0; i < 5; i++)
{
snake[i][0] = x - i;
snake[i][1] = y;
}
length = 5;
}
void initFood()
{
srand(time(NULL));
int x = rand() % (width - 1) + 1;
int y = rand() % (height - 1) + 1;
food[0] = x;
food[1] = y;
}
void drawSnake()
{
for (int i = 0; i < length; i++)
{
gotoxy(snake[i][0], snake[i][1]);
printf("*");
}
}
void drawFood()
{
gotoxy(food[0], food[1]);
printf("#");
}
void move()
{
switch (direction)
{
case UP:
snake[length][0] = snake[length - 1][0];
snake[length][1] = snake[length - 1][1] - 1;
break;
case DOWN:
snake[length][0] = snake[length - 1][0];
snake[length][1] = snake[length - 1][1] + 1;
break;
case LEFT:
snake[length][0] = snake[length - 1][0] - 1;
snake[length][1] = snake[length - 1][1];
break;
case RIGHT:
snake[length][0] = snake[length - 1][0] + 1;
snake[length][1] = snake[length - 1][1];
break;
}
if (snake[length][0] == food[0] && snake[length][1] == food[1])
{
initFood();
length++;
score++;
if (score % 5 == 0)
{
speed -= 10;
}
}
for (int i = 0; i < length; i++)
{
if (i == length - 1)
{
break;
}
if (snake[length - 1][0] == snake[i][0] && snake[length - 1][1] == snake[i][1])
{
printf("\a");
gotoxy(0, height + 1);
printf("Game Over!\n");
exit(0);
}
}
for (int i = 0; i < length - 1; i++)
{
snake[i][0] = snake[i + 1][0];
snake[i][1] = snake[i + 1][1];
}
}
void updateScore()
{
gotoxy(0, height + 1);
printf("Score: %d", score);
}
void changeDirection()
{
if (_kbhit())
{
char ch = _getch();
switch (ch)
{
case 'w':
case 'W':
if (direction != DOWN)
{
direction = UP;
}
break;
case 's':
case 'S':
if (direction != UP)
{
direction = DOWN;
}
break;
case 'a':
case 'A':
if (direction != RIGHT)
{
direction = LEFT;
}
break;
case 'd':
case 'D':
if (direction != LEFT)
{
direction = RIGHT;
}
break;
}
}
}
void gameLoop()
{
while (1)
{
drawSnake();
drawFood();
updateScore();
changeDirection();
move();
Sleep(speed);
system("cls");
}
}
int main()
{
hidecursor();
initSnake();
initFood();
gameLoop();
return 0;
}
```
代码中用到了 Windows API 中的一些函数,如 `getch()`、`Sleep()`、`SetConsoleCursorPosition()` 等,需要在 Windows 平台上运行。其中,`initSnake()` 函数初始化蛇的位置,`initFood()` 函数初始化食物的位置,`drawSnake()` 和 `drawFood()` 函数分别绘制蛇和食物,`move()` 函数控制蛇的移动,`updateScore()` 函数更新分数,`changeDirection()` 函数监听键盘输入并改变蛇的移动方向,`gameLoop()` 函数是游戏的主循环,其中不断重绘和更新蛇、食物、分数等。
阅读全文
相关推荐
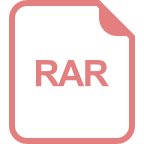















