[[nodiscard]] shared_ptr<const _Ty> shared_from_this() const{ return shared_ptr<const _Ty>(_Wptr) }
时间: 2024-12-25 20:32:33 浏览: 6
这段代码片段是在C++标准库中的智能指针`std::shared_ptr`内部的一个成员函数`shared_from_this()`的实现。`shared_from_this()`是一个用于获取当前对象自身引用的方法,当被其他已经持有该对象引用的共享所有权容器(如`shared_ptr`)所持有时。这里`_Ty`代表指向的对象类型,`const`说明返回的是常量引用。
函数返回了一个`shared_ptr<const _Ty>`类型的临时引用,即一个指向常量对象的共享所有权指针。这个方法的作用是在不需要传递原始对象的情况下,让外部拥有者能够间接访问并控制这个对象,同时保证了线程安全。
示例使用:
```cpp
class MyClass {
public:
std::shared_ptr<const MyClass> shared_from_this() const {
return shared_ptr<const MyClass>(static_cast<MyClass*>(this));
}
};
MyClass obj;
auto shared_ref = obj.shared_from_this();
```
相关问题
无法将参数1从std::shared_ptr<PointCloud<PointXYZ>>转为为const boost::shared_ptr<const PointCloud<PointXYZ>>
在C++中,`std::shared_ptr` 和 `boost::shared_ptr` 都是智能指针,用于管理动态分配的对象的生命周期。当你尝试从一个 `std::shared_ptr<PointCloud<PointXYZ>>` 转换为 `const boost::shared_ptr<const PointCloud<PointXYZ>>` 时,可能会遇到问题,因为转换不是直接兼容的。
`PointCloud<PointXYZ>` 是一个非常量类型,而 `const boost::shared_ptr<const PointCloud<PointXYZ>>` 指向的是一个不可修改的常量指针,这意味着你需要确保原始的 `std::shared_ptr` 指向的数据是可以被视为常量的,并且转换后的指针应该保持这个常量性质。
如果你想要这样做,通常需要满足以下条件:
1. 数据本身已经是常量,即 `PointCloud<PointXYZ>` 实际上是一个 `const PointCloud<PointXYZ>`。
2. 或者,如果数据允许临时 const 属性,那么可以复制一份新的常量版本并传递指向它的共享指针。
如果你不能满足上述条件,编译器会报错,因为默认情况下这种转换是不允许的。为了完成这种转换,你需要显式地创建一个新的 `const` 版本的指针,例如:
```cpp
// 假设cloud是已知的std::shared_ptr
if (cloud->lock()->get() == cloud.lock()) { // 确保数据是const的
const auto cloud_const = std::make_shared<const PointCloud<PointXYZ>>(*cloud); // 创建新常量指针
// 使用cloud_const...
}
```
没有与这些操作数匹配的 "=" 运算符C/C++(349) listener_str.cpp(12, 23): 操作数类型为: std::shared_ptr<rclcpp::Subscription<std_msgs::msg::String, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<std_msgs::msg::String, std::allocator<void>>>> = std::shared_ptr<rclcpp::Subscription<const std::shared_ptr<std_msgs::msg::String_<std::allocator<void>>> &, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<const std::shared_ptr<std_msgs::msg::String_<std::allocator<void>>> &, std::allocator<void>>>>
这个错误通常是因为尝试将一个类型为`std::shared_ptr<rclcpp::Subscription<const std::shared_ptr<std_msgs::msg::String_<std::allocator<void>>> &, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<const std::shared_ptr<std_msgs::msg::String_<std::allocator<void>>> &, std::allocator<void>>>>`的变量赋值给一个类型为`std::shared_ptr<rclcpp::Subscription<std_msgs::msg::String, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<std_msgs::msg::String, std::allocator<void>>>>`的变量,这两个类型虽然都是指向`rclcpp::Subscription`的`shared_ptr`,但是模板参数不同,无法直接赋值。
解决方法是将两个类型匹配,可以通过使用`std::static_pointer_cast`将其中一个类型转换成另一个类型,例如:
```
std::shared_ptr<rclcpp::Subscription<std_msgs::msg::String, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<std_msgs::msg::String, std::allocator<void>>>> sub;
std::shared_ptr<rclcpp::Subscription<const std::shared_ptr<std_msgs::msg::String_<std::allocator<void>>> &, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<const std::shared_ptr<std_msgs::msg::String_<std::allocator<void>>> &, std::allocator<void>>>> sub_const;
// 将 sub_const 转换成 sub 的类型
sub = std::static_pointer_cast<rclcpp::Subscription<std_msgs::msg::String, std::allocator<void>, rclcpp::message_memory_strategy::MessageMemoryStrategy<std_msgs::msg::String, std::allocator<void>>>>(sub_const);
```
阅读全文
相关推荐
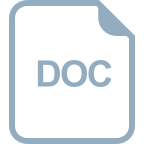
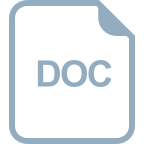
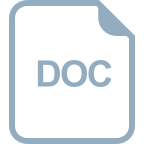











class B { public: B(int value) : value_(value) {} int getValue() const { return value_; } private: int value_; }; class A { public: void setB(shared_ptr b) { pb_ = b; } void doSomething() { if (auto p = pb_.lock()) cout << "A::doSomething() called, B's value is " << p->getValue() << endl; else cout << "B has been deleted" << endl; } private: weak_ptr pb_; }; int main() { shared_ptr pa = make_shared();//先创建对象A和B shared_ptr pb = make_shared(42); pa->setB(pb);//将对象B传递给A(这里使用weak_ptr传递) pa->doSomething(); pb.reset(); pa->doSomething(); return 0; }注释



