Openssl3 rsaaes-oaep解密c++代码
时间: 2024-11-26 14:21:03 浏览: 0
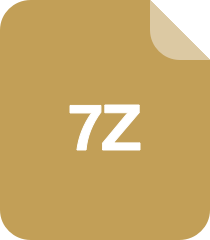
Win64OpenSSL-3-1-0.exe
OpenSSL是一个流行的开源加密库,在C++中使用它进行RSA算法(包括RSAAES-OAEP)的操作涉及到一些库函数调用。RSAAES-OAEP是一种用于RSA公钥加密的数据封装机制,提供了一种安全的方式对数据进行加密。
下面是一个简化的C++示例,展示了如何使用OpenSSL 3.x进行RSA AES-OAEP解密:
```cpp
#include <openssl/aes.h>
#include <openssl/pem.h>
#include <openssl/rsa.h>
// 解密函数
std::vector<uint8_t> rsa_aes_oaep_decrypt(const std::string& encrypted_data,
const RSA* rsa_key) {
// 加载私钥
BIO* bio = BIO_new_mem_buf(pem_password_cb, -1);
if (!bio || !PEM_read_bio_RSAPrivateKey(bio, &rsa_key, NULL, NULL)) {
throw std::runtime_error("Failed to load RSA private key");
}
BIO_free_all(bio);
// 将数据转换为OpenSSL字节缓冲
unsigned char *decrypted_buffer = new unsigned char[AES_BLOCK_SIZE];
int decrypted_length;
if (RSA_public_decrypt(encrypted_data.size(), reinterpret_cast<const unsigned char*>(encrypted_data.data()),
decrypted_buffer, rsa_key, RSA_PKCS1_OAEP_PADDING) <= 0) {
delete[] decrypted_buffer;
throw std::runtime_error("Decryption failed");
}
// 返回解密后的数据
std::vector<uint8_t> decrypted(decrypted_buffer, decrypted_buffer + decrypted_length);
delete[] decrypted_buffer;
return decrypted;
}
// 定义密码回调函数,这里假设为无密码
static int pem_password_cb(char* buf, int size, int rwflag, void* u) {
memset(buf, 0, size);
return 0;
}
阅读全文
相关推荐
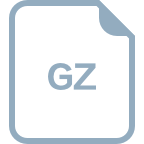















