尝试编写一个算法来计算霍夫曼树的 WPL。
时间: 2024-02-05 18:11:12 浏览: 85
首先,我们需要知道什么是霍夫曼树和 WPL。
霍夫曼树是一种满足最小带权路径长度的二叉树,其中带权路径长度是指从根节点到叶子节点的路径长度乘以该节点的权值之和。WPL(Weighted Path Length)是指霍夫曼树的带权路径长度。
计算霍夫曼树的 WPL 的算法如下:
1. 对所有节点的权值进行排序,按照从小到大的顺序依次取出两个权值最小的节点。
2. 将这两个节点合并成一个新节点,权值为这两个节点的权值之和。将新节点插入到原来的节点集合中,并删除原来的两个节点。
3. 重复步骤1和步骤2,直到所有的节点都被合并成一个根节点为止。
4. 对霍夫曼树进行遍历,计算每个叶子节点的带权路径长度,将它们相加得到 WPL。
下面是 Python 代码实现:
```python
class Node:
def __init__(self, val, weight):
self.val = val
self.weight = weight
self.left = None
self.right = None
def huffman_tree_wpl(nodes):
# 1. 对节点按权值排序
nodes.sort(key=lambda x: x.weight)
# 2. 构建霍夫曼树
while len(nodes) > 1:
left = nodes.pop(0)
right = nodes.pop(0)
parent = Node(None, left.weight + right.weight)
parent.left = left
parent.right = right
nodes.append(parent)
nodes.sort(key=lambda x: x.weight)
# 3. 计算 WPL
def dfs(node, depth):
if not node:
return 0
if not node.left and not node.right:
return depth * node.weight
return dfs(node.left, depth + 1) + dfs(node.right, depth + 1)
root = nodes[0]
wpl = dfs(root, 0)
return wpl
```
这个算法的时间复杂度为 $O(n \log n)$,其中 $n$ 是节点的数量。
阅读全文
相关推荐
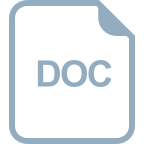
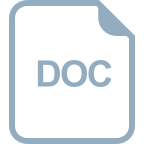
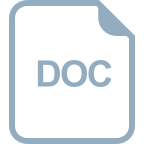
















