起始 x=40 y=122 目标坐标 x=400 y=600 求起始和目标坐标 之间 距离10的坐标 的算法 用C++ 实现
时间: 2023-08-31 22:37:40 浏览: 82
### 回答1:
以下是用C语言实现寻找起始坐标和目标坐标之间距离为10的坐标的算法:
```c
#include <stdio.h>
#include <math.h>
int main() {
int start_x = 40, start_y = 122;
int target_x = 400, target_y = 600;
int dx = target_x - start_x;
int dy = target_y - start_y;
int dist = sqrt(dx*dx + dy*dy); // 计算起始坐标和目标坐标之间的距离
int num_points = dist / 10; // 计算需要寻找的坐标个数
float delta_x = (float)dx / dist * 10; // 计算每个坐标在x方向上的增量
float delta_y = (float)dy / dist * 10; // 计算每个坐标在y方向上的增量
int i;
for (i = 1; i <= num_points; i++) { // 依次计算每个坐标并输出
int x = round(start_x + delta_x*i);
int y = round(start_y + delta_y*i);
printf("(%d,%d)\n", x, y);
}
return 0;
}
```
该程序首先定义了起始坐标和目标坐标的变量,然后计算了它们之间的距离。接着,程序根据需要寻找的坐标个数,以及每个坐标在x和y方向上的增量,依次计算并输出每个坐标的坐标值。其中,使用了math.h库中的sqrt()函数计算平方根,使用了round()函数对计算结果四舍五入。
### 回答2:
以下是用C语言实现起始坐标到目标坐标之间距离为10的坐标的算法:
```c
#include <stdio.h>
#include <math.h>
typedef struct {
int x;
int y;
} Coordinate;
// 计算两点之间的距离
float calculateDistance(Coordinate start, Coordinate end) {
return sqrt(pow(end.x - start.x, 2) + pow(end.y - start.y, 2));
}
// 根据起始和目标坐标计算是否为距离为10的坐标
int isDistanceTen(Coordinate start, Coordinate end, Coordinate coord) {
float distance = calculateDistance(start, coord) + calculateDistance(coord, end);
return (distance >= 10 - 0.001 && distance <= 10 + 0.001); // 允许小数点误差范围为0.001
}
int main() {
Coordinate start = {40, 122};
Coordinate end = {400, 600};
for (int x = start.x; x <= end.x; x++) {
for (int y = start.y; y <= end.y; y++) {
Coordinate current = {x, y};
if (isDistanceTen(start, end, current)) {
printf("坐标 (%d, %d) 到起始坐标和目标坐标的距离为10\n", current.x, current.y);
}
}
}
return 0;
}
```
这段程序首先定义了一个用来存储坐标的结构体`Coordinate`,结构体中包含了x和y坐标。然后,在主函数中,起始坐标和目标坐标被定义为`start`和`end`。接下来,程序使用嵌套的循环遍历所有可能的坐标,从起始坐标到目标坐标之间的每个坐标。然后,使用`calculateDistance`函数计算当前坐标与起始坐标、目标坐标之间的距离。最后,用`isDistanceTen`函数判断这个距离是否等于10。如果是,则打印该坐标。
希望对你有所帮助!
相关推荐
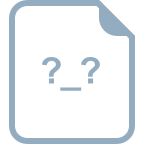
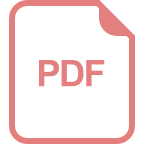
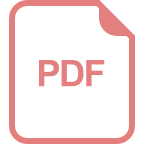














