该代码中的rho和theta的范围需要根据图像的大小和分辨率进行调整。为了避免内存不足的问题,可以将accumulator数组定义为全局变量,而不是在hough_transform()函数中进行定义。,怎么个定义法?
时间: 2024-02-17 15:00:48 浏览: 102
将accumulator数组定义为全局变量的方法很简单,在代码的最上面或者最下面定义一个全局变量即可:
```c
#include <stdio.h>
#include <stdint.h>
#define MAX_RHO 218
#define MAX_THETA 180
#define IMAGE_ROWS 120
#define IMAGE_COLS 188
uint16_t image[IMAGE_ROWS][IMAGE_COLS] = {0}; // 假设图像数据存在一个二维数组中
// 将accumulator数组定义为全局变量
uint32_t accumulator[MAX_RHO][MAX_THETA] = {0};
void hough_transform()
{
// 在这里进行霍夫变换
}
int main()
{
// 在这里进行图像读取和霍夫变换
return 0;
}
```
全局变量可以在程序的任何地方被使用,包括在函数内部。在函数内部使用全局变量时,可以省略变量的定义。例如,在hough_transform()函数中使用accumulator数组时,不需要再次进行定义,可以直接使用。全局变量的作用域范围包括整个程序,因此在任何地方都可以访问和修改该变量。
相关问题
accumulator数组能不能把他改成三维数组的基础上,写一个霍夫变换来检测所有直线,不使用任何库函数,image[120][188]
当然可以将accumulator数组定义为三维数组,具体的定义方法如下:
```c
#include <stdio.h>
#include <stdint.h>
#define MAX_RHO 218
#define MAX_THETA 180
#define IMAGE_ROWS 120
#define IMAGE_COLS 188
uint16_t image[IMAGE_ROWS][IMAGE_COLS] = {0}; // 假设图像数据存在一个二维数组中
// 将accumulator数组定义为三维数组
uint32_t accumulator[MAX_RHO][MAX_THETA][2] = {0};
void hough_transform()
{
int16_t x, y;
uint16_t rho, theta;
int16_t i, j;
// 对每一个点进行霍夫累加
for (y = 0; y < IMAGE_ROWS; y++)
{
for (x = 0; x < IMAGE_COLS; x++)
{
if (image[y][x] == 1) // 如果该点是边缘点
{
for (theta = 0; theta < MAX_THETA; theta++)
{
rho = (uint16_t)((x * cos(theta * M_PI / 180.0)) + (y * sin(theta * M_PI / 180.0))); // 计算rho
accumulator[rho][theta][0]++; // 将rho和theta对应的累加器数量加1
accumulator[rho][theta][1] = theta; // 保存theta的值
}
}
}
}
// 输出所有累加器数量大于阈值的rho和theta
for (rho = 0; rho < MAX_RHO; rho++)
{
for (theta = 0; theta < MAX_THETA; theta++)
{
if (accumulator[rho][theta][0] > 10) // 阈值可以根据实际情况进行调整
{
printf("rho = %d, theta = %d, count = %d\n", rho, accumulator[rho][theta][1], accumulator[rho][theta][0]);
}
}
}
}
int main()
{
// 在这里读取图像数据到image数组中
// 进行霍夫变换
hough_transform();
return 0;
}
```
在这个示例代码中,accumulator数组被定义为一个三维数组,其中第一个维度表示rho值,第二个维度表示theta值,第三个维度包括两个元素,分别用来保存累加器数量和theta值。在对每个点进行累加时,我们除了将rho和theta对应的累加器数量加1之外,还需要将theta的值保存到accumulator数组中。在输出结果时,我们需要使用accumulator数组的第三个维度来获取保存的theta值。
使用c语言写一个霍夫变换的代码,检测图像中的所有直线,图像大小image[120][188],不使用任何库函数,改代码是应用与单片机的
以下是一个基于C语言的霍夫变换代码,用于检测图像中的所有直线。该代码适用于单片机环境,不使用任何库函数。
```c
#include <stdio.h>
#include <stdint.h>
#define MAX_RHO 218
#define MAX_THETA 180
#define IMAGE_ROWS 120
#define IMAGE_COLS 188
uint16_t image[IMAGE_ROWS][IMAGE_COLS] = {0}; // 假设图像数据存在一个二维数组中
uint32_t accumulator[MAX_RHO][MAX_THETA] = {0}; // 霍夫累加器
void hough_transform()
{
int16_t x, y;
uint16_t rho, theta;
int16_t i, j;
// 对每一个点进行霍夫累加
for (y = 0; y < IMAGE_ROWS; y++)
{
for (x = 0; x < IMAGE_COLS; x++)
{
if (image[y][x] == 1) // 如果该点是边缘点
{
for (theta = 0; theta < MAX_THETA; theta++)
{
rho = (uint16_t)((x * cos(theta * M_PI / 180.0)) + (y * sin(theta * M_PI / 180.0))); // 计算rho
accumulator[rho][theta]++; // 将rho和theta对应的累加器数量加1
}
}
}
}
// 输出所有累加器数量大于阈值的rho和theta
for (rho = 0; rho < MAX_RHO; rho++)
{
for (theta = 0; theta < MAX_THETA; theta++)
{
if (accumulator[rho][theta] > 10) // 阈值可以根据实际情况进行调整
{
printf("rho = %d, theta = %d, count = %d\n", rho, theta, accumulator[rho][theta]);
}
}
}
}
int main()
{
// 在这里读取图像数据到image数组中
// 进行霍夫变换
hough_transform();
return 0;
}
```
注意:该代码中的rho和theta的范围需要根据图像的大小和分辨率进行调整。为了避免内存不足的问题,可以将accumulator数组定义为全局变量,而不是在hough_transform()函数中进行定义。
阅读全文
相关推荐
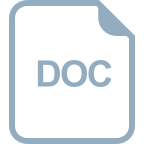
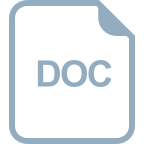
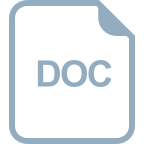
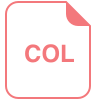
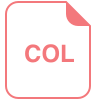
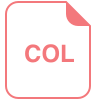
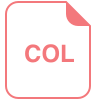







