最小费用最大流matlab
时间: 2023-07-20 21:45:22 浏览: 75
最小费用最大流是一个经典的网络流问题,可以使用MATLAB来解决。MATLAB中有许多网络流算法的实现,其中包括最小费用最大流算法。以下是一个简单的MATLAB代码,可以用来解决最小费用最大流问题:
```matlab
function [f, cost] = min_cost_max_flow(C, s, t, demand)
% C: cost matrix, C(i,j) is the cost of sending one unit of flow from i to j
% s: source node
% t: sink node
% demand: demand vector, demand(i) is the demand at node i
% f: flow matrix, f(i,j) is the flow from i to j
% cost: the minimum cost of sending the required demand
n = size(C, 1); % number of nodes
f = zeros(n); % initialize flow matrix
while true
% find shortest path from s to t using the Bellman-Ford algorithm
[dist, prev] = bellman_ford(C, s);
if dist(t) == inf % if there is no path from s to t, terminate
break
end
% initialize residual capacity matrix
res_cap = zeros(n);
for i = 1:n
for j = 1:n
res_cap(i,j) = C(i,j) - f(i,j);
end
end
% find the bottleneck capacity
bottleneck = demand(t);
node = t;
while node ~= s
bottleneck = min(bottleneck, res_cap(prev(node), node));
node = prev(node);
end
% update flow matrix and demand vector
node = t;
while node ~= s
f(prev(node), node) = f(prev(node), node) + bottleneck;
f(node, prev(node)) = f(node, prev(node)) - bottleneck;
demand(node) = demand(node) - bottleneck;
demand(prev(node)) = demand(prev(node)) + bottleneck;
node = prev(node);
end
end
% calculate minimum cost
cost = 0;
for i = 1:n
for j = 1:n
cost = cost + f(i,j) * C(i,j);
end
end
end
function [dist, prev] = bellman_ford(C, s)
% C: cost matrix, C(i,j) is the cost of sending one unit of flow from i to j
% s: source node
% dist: distance vector, dist(i) is the shortest distance from s to i
% prev: predecessor vector, prev(i) is the node that precedes i on the shortest path from s to i
n = size(C, 1); % number of nodes
dist = inf(1, n); % initialize distance vector
prev = zeros(1, n); % initialize predecessor vector
dist(s) = 0; % distance from source to source is zero
for k = 1:n-1 % iterate n-1 times
for i = 1:n
for j = 1:n
if C(i,j) ~= 0 && dist(i) + C(i,j) < dist(j)
dist(j) = dist(i) + C(i,j);
prev(j) = i;
end
end
end
end
end
```
这个代码实现了最小费用最大流算法,其中使用了Bellman-Ford算法来寻找最短路径。你可以根据自己的需求来调整代码,并在MATLAB中运行它。
相关推荐
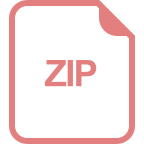
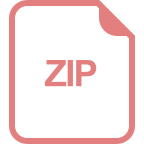














